Write a Java program to generate random numbers with an example. For example, we can use the Math random method, class, and ThreadLocalRandom class to generate numbers.
The Java random generator is a Math Library function used to generate and return the Pseudo-random number between Zero and One. The basic syntax of the Math random is as shown below.
static double random(); //Return Type is Double // In order to use in program: Math.random();
Java Program to Generate Random Numbers using Math function
The Java Math random generator Function returns the Pseudo-random numbers between 0 and 1. In this program, we will use that function and display the output.
package MathFunctions; public class RandomMethod { public static void main(String[] args) { System.out.println(Math.random()); System.out.println(Math.random()); System.out.println(Math.random()); System.out.println(Math.random()); } }
The value generated by the random function is from 0 (included) and less than 1. If you observe the below output, We called the Function four times, returning four different values.
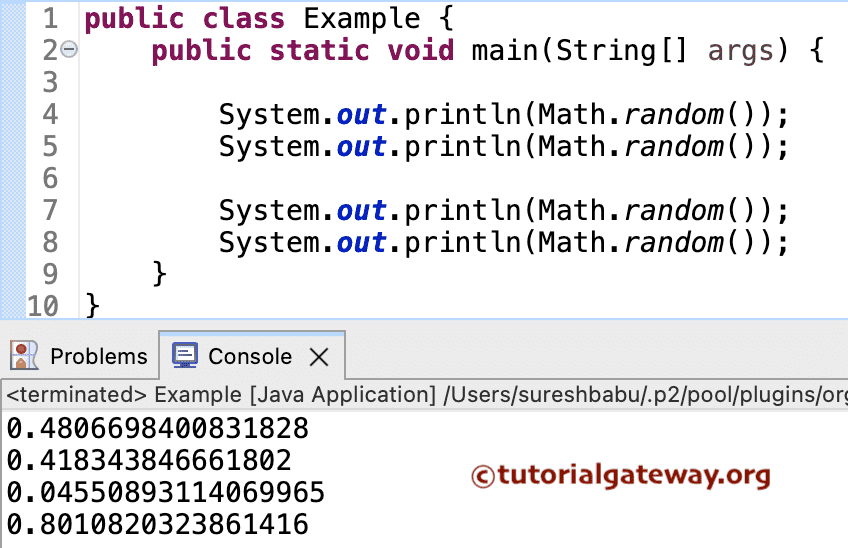
We use the Math.random() method in this example, which generates numbers between 0 and 1 of double type. We can also typecast the output to get the necessary data type.
package RemainingSimplePrograms; public class Example { public static void main(String[] args) { System.out.println("The First = " + Math.random()); System.out.println("The Second = " + Math.random()); System.out.println("The Third = " + Math.random()); System.out.println("The Fourth = " + Math.random()); } }
The First = 0.20037158212760142
The Second = 0.0012068894419960952
The Third = 0.18700555955501663
The Fourth = 0.3230234616758232
By using the Javac Math.random() * (max – min + 1) + min we can generate the numbers between the min and max values.
package RemainingSimplePrograms; public class Example2 { public static void main(String[] args) { int min = 100, max = 300; System.out.print("The Doubles between " + min + " and " + max + " = " ); double rand1 = Math.random() * (max - min + 1) + min; System.out.print(rand1); System.out.print("\nThe Number between " + min + " and " + max + " = " ); int rand2 = (int)(Math.random() * (max - min + 1) + min); System.out.print(rand2); } }
The Doubles between 100 and 300 = 101.43411590322778
The Number between 100 and 300 = 282
Java Program to Generate Random Numbers using for loop
Until now, we generated a single value between a range. However, with the help of a for loop, we can generate them within a range. In this program, we will show how to store random values in an array. Here, we will declare an array of double types and fill that array with distinct values generated by Math.random.
First, We declared an empty double Array. Next, We used Java For Loop to iterate the Array. Within the For Loop, we initialized the i value as 0. The compiler will check for the condition (i < 10).
The following Java Random Number Generator function statements will store the values in an array. If you observe the code snippet, we assign each index position with one value.
The compiler will call the Math function (static double random() ) to return a value between 0 and 1.
Next, to display the array values, We used another For Loop to iterate the Array. Within the For Loop, we initialized i value as 0, and the compiler will check the condition (i < myArray.length). The myArray.length finds the length of an array.
Within the loop, the Java System.out.println statements print the output.
package MathFunctions; public class RandomMethodInArray { public static void main(String[] args) { double [] myArray = new double[10]; for (int i = 0; i < 10; i++) { myArray[i] = Math.random(); } //Displaying Result for (int i = 0; i < myArray.length; i++) { System.out.println("Array Element = " + myArray[i]); } } }
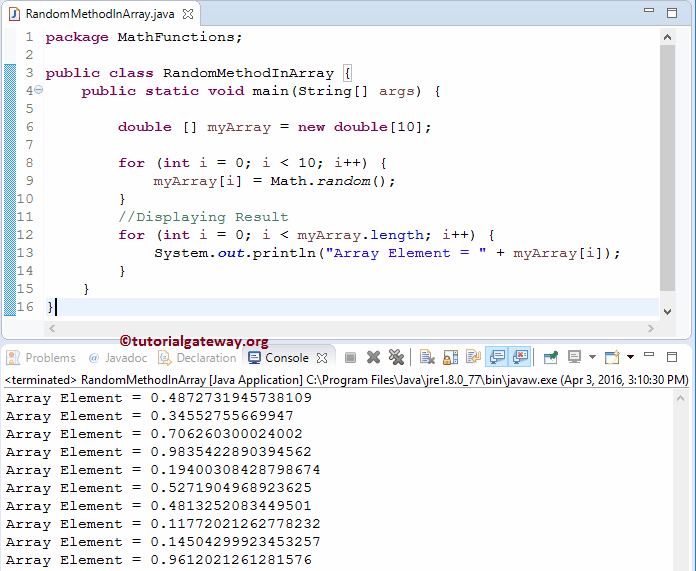
It is another example of generating an array of random numbers.
public class Example { public static void main(String[] args) { int min = 10, max = 100; System.out.println("Ten Numbers between " + min + " and " + max); for(int i = 1; i <= 10; i++) { int rdNum = (int)(Math.random() * (max - min + 1) + min); System.out.print(rdNum + " "); } System.out.println("\nTwenty Numbers between 100 and 250"); for(int i = 1; i <= 10; i++) { int rdNum = (int)(Math.random() * (250 - 100 + 1) + 100); System.out.print(rdNum + " "); } } }
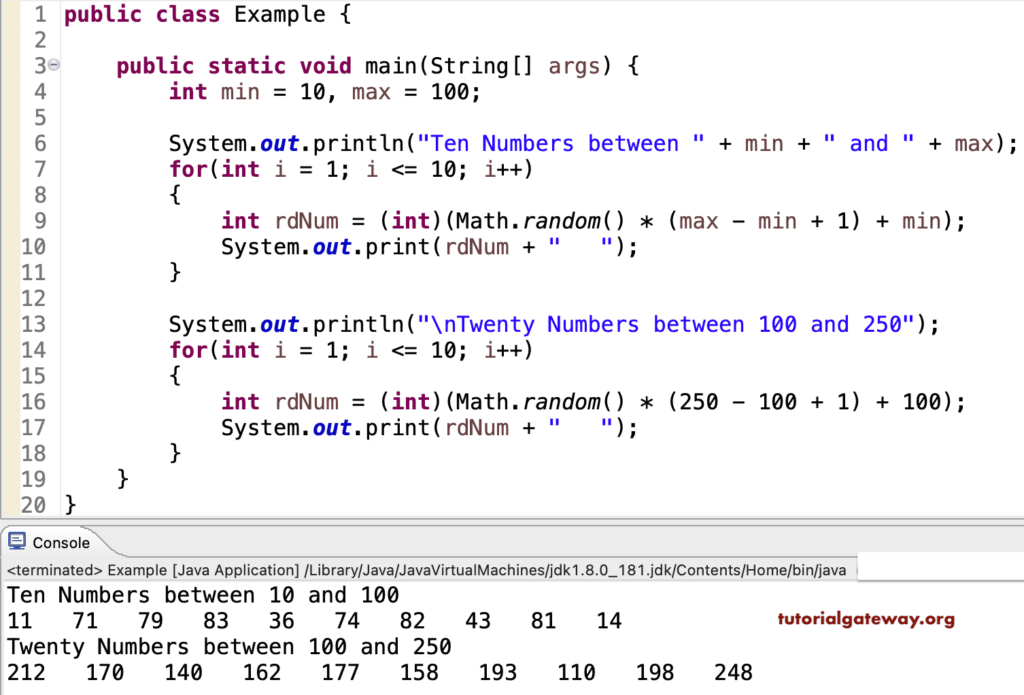
Java Program to Generate Random Numbers using class
This random class has different methods to generate numbers. They are
- nextInt() – Generate values between 0 and -1
- nextInt(max_Value)
- nextFloat() – Returns values between 0.0 and 1.0
- nextDouble() – Return values between 0.0 and 1.0
- nextLong()
- nextBoolean()
package RemainingSimplePrograms; import java.util.Random; public class Example4 { public static void main(String[] args) { Random rand = new Random(); System.out.println("Integer Values"); System.out.println(rand.nextInt()); System.out.println(rand.nextInt(20)); System.out.println(rand.nextInt(100)); System.out.println("Double Values"); System.out.println(rand.nextDouble()); System.out.println(rand.nextDouble()); System.out.println("Float Values"); System.out.println(rand.nextFloat()); System.out.println(rand.nextFloat()); System.out.println("Long Values"); System.out.println(rand.nextLong()); System.out.println(rand.nextLong()); } }
Integer Values
1907114976
4
34
Double Values
0.9425130257739933
0.35445514266974565
Float Values
0.26128042
0.47151804
Long Values
1983140345491940719
8269807721314480176
In this example, we used for loop to generate ten random numbers of Integer type of max limit value 100.
package RemainingSimplePrograms; import java.util.Random; public class Example5 { public static void main(String[] args) { Random rand = new Random(); for(int i = 1; i <= 10; i++) { System.out.print(rand.nextInt(100) + 1 + " "); } } }
9 45 62 84 18 2 10 33 28 60
Java Program to Generate Random Numbers using ThreadLocalRandom
We can also use the ThreadLocalRandom to generate random numbers within a range. The nextInt(), nextDouble(), and nextLong() accepts the minimum and maximum limit value.
package RemainingSimplePrograms; import java.util.concurrent.ThreadLocalRandom; public class Example6 { public static void main(String[] args) { System.out.println("Integer Values"); System.out.println(ThreadLocalRandom.current().nextInt()); System.out.println(ThreadLocalRandom.current().nextInt(20)); System.out.println(ThreadLocalRandom.current().nextInt(100, 500)); System.out.println("Double Values"); System.out.println(ThreadLocalRandom.current().nextDouble()); System.out.println(ThreadLocalRandom.current().nextDouble(50)); System.out.println(ThreadLocalRandom.current().nextDouble(30, 50)); System.out.println("Float Values"); System.out.println(ThreadLocalRandom.current().nextFloat()); System.out.println(ThreadLocalRandom.current().nextFloat()); System.out.println("Long Values"); System.out.println(ThreadLocalRandom.current().nextLong()); System.out.println(ThreadLocalRandom.current().nextLong(40)); System.out.println(ThreadLocalRandom.current().nextLong(400, 600)); } }
Integer Values
536433268
17
105
Double Values
0.35695024289926547
29.875628014935078
45.29405097588917
Float Values
0.62107533
0.9211104
Long Values
-1523132321353307594
4
542
This program uses ThreadLocalRandom and the for loop.
package RemainingSimplePrograms; import java.util.concurrent.ThreadLocalRandom; public class Example7 { public static void main(String[] args) { int min = 100, max = 300; System.out.println("Ten Numbers are"); for(int i = 1; i <= 10; i++) { System.out.print(ThreadLocalRandom.current().nextInt(100) + 1 + " "); } System.out.println("\nFifteen Numbers are"); for(int i = 0; i < 15; i++) { int rdNum = ThreadLocalRandom.current().nextInt(min, max); System.out.print(rdNum + " "); } } }
Ten Numbers are
10 88 47 68 41 79 45 74 1 41
Fifteen Numbers are
262 187 267 255 255 265 136 201 228 255 166 156 115 291 169