This blog post shows how to write a Java program to print the Pyramid pattern of stars, numbers, and alphabets with an example of each. It also covers the hollow, inverted, and inverted hollow pyramid patterns with the best approach.
Although this article shows the best possible way to print the various kinds of pyramid patterns, we haven’t mentioned the code analysis. Because there is a dedicated article for each sub-section, and we provide a hyperlink to it. Please click the link to find an alternative approach and the code explanation.
Java Program to Print Pyramid Pattern of Stars
This program accepts the user-inserted rows. Next, we used the nested for loop to iterate the rows and columns and print the pyramid pattern. Please refer to this article to understand the code.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Rows = "); int rw = sc.nextInt(); for (int i = 1; i <= rw; i++) { for (int j = 0; j < rw - i; j++) { System.out.print(" "); } for (int k = 0; k < (i * 2) - 1; k++) { System.out.print("*"); } System.out.println(); } } }
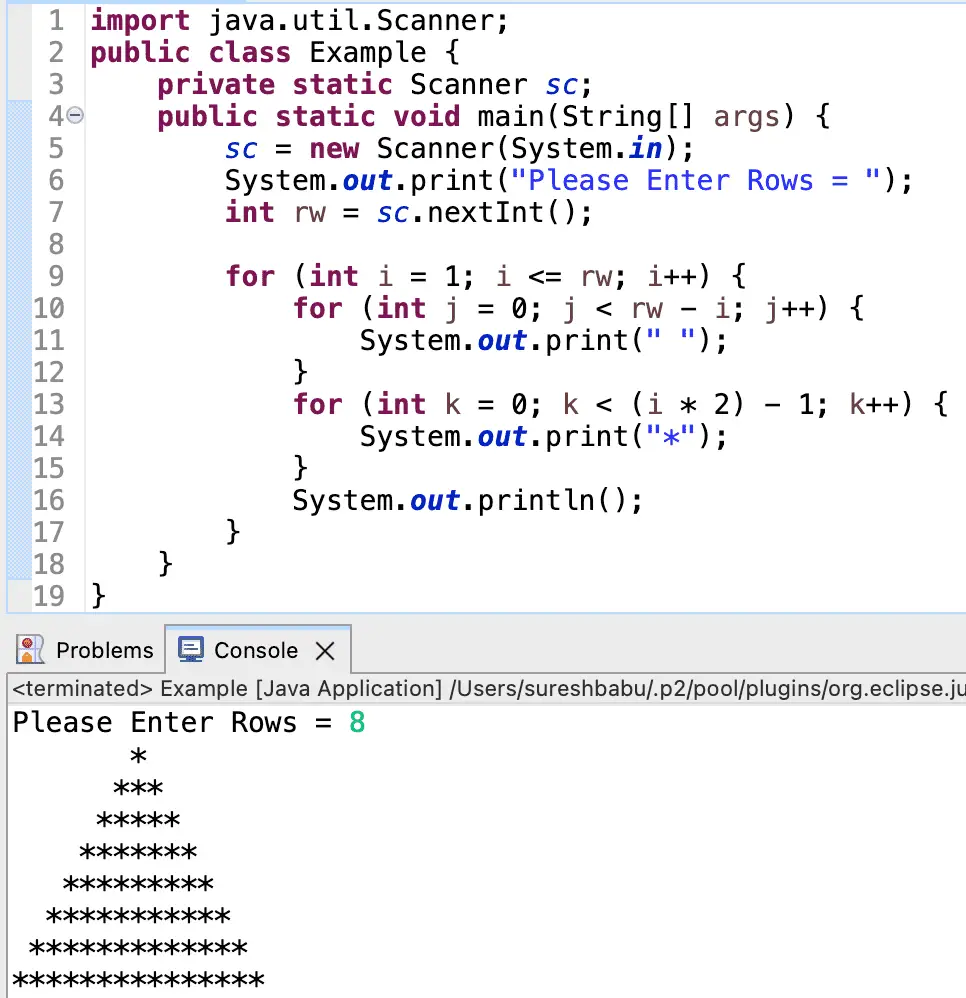
Hollow Pyramid Star Pattern
For more information on hollow example >> Click Here.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Rows = "); int rw = sc.nextInt(); int i, j, k; for (i = 1; i <= rw; i++) { for (j = 1; j <= rw - i; j++) { System.out.print(" "); } if (i == 1 || i == rw) { for (k = 1; k <= (i * 2) - 1; k++) { System.out.print("*"); } } else { for (k = 1; k <= (i * 2) - 1; k++) { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } } } System.out.println(); } } }
Output
Enter Rows = 12
*
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
***********************
Inverted Pyramid Pattern of Stars
For more information on inverted >> Click Here.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter rows = "); int rw = sc.nextInt(); for (int i = rw; i >= 1; i--) { for (int j = 0; j < rw - i; j++) { System.out.print(" "); } for (int k = 0; k != (2 * i) - 1; k++) { System.out.print("*"); } System.out.println(); } } }
Output
Enter rows = 10
*******************
*****************
***************
*************
***********
*********
*******
*****
***
*
Hollow Inverted Pyramid of Stars
For more information on hollow inverted >> Click Here.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Rows = "); int rw = sc.nextInt(); for (int i = rw ; i > 0; i-- ) { for (int j = 1 ; j <= rw - i; j++ ) { System.out.print(" "); } for (int k = 1 ; k <= (2 * i) - 1; k++ ) { if (i == 1 || i == rw || k == 1 || k == (2 * i) - 1) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(); } } }
Output
Enter Rows = 9
*****************
* *
* *
* *
* *
* *
* *
* *
*
Java Program to Print Pyramid Pattern of Numbers
The above-mentioned programs print the pyramid pattern of stars however, you can also use the numbers to fill the pattern. For more information >> Click Here.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Rows = "); int rw = sc.nextInt(); for (int i = 1; i <= rw; i++ ) { for (int j = rw; j > i; j-- ) { System.out.print(" "); } for(int k = 1; k <= i; k++) { System.out.print(i + " "); } System.out.println(); } } }
Output
Enter Rows = 9
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
6 6 6 6 6 6
7 7 7 7 7 7 7
8 8 8 8 8 8 8 8
9 9 9 9 9 9 9 9 9
Java Program to Print Pyramid Pattern of Alphabets
This example program prints the characters or alphabets to fill the pattern. For more information >> Click Here.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Rows = "); int rw = sc.nextInt(); int alp = 64; for (int i = 1; i <= rw; i++) { for (int j = 1; j <= rw - i; j++) { System.out.print(" "); } for (int k = i; k > 0; k--) { System.out.print((char) (alp + k)); } for (int l = 2; l <= i; l++) { System.out.print((char) (alp + l)); } System.out.println(); } } }
Output
Enter Rows = 14
A
BAB
CBABC
DCBABCD
EDCBABCDE
FEDCBABCDEF
GFEDCBABCDEFG
HGFEDCBABCDEFGH
IHGFEDCBABCDEFGHI
JIHGFEDCBABCDEFGHIJ
KJIHGFEDCBABCDEFGHIJK
LKJIHGFEDCBABCDEFGHIJKL
MLKJIHGFEDCBABCDEFGHIJKLM
NMLKJIHGFEDCBABCDEFGHIJKLMN