Write a Java Program to print a pyramid star pattern using for loop. This example uses two for loops nested inside one another to iterate the rows and display the stars.
package ShapePrograms; import java.util.Scanner; public class PyramidPattern1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Pyramid Pattern Rows = "); int rows = sc.nextInt(); System.out.println("--- Printing Pyramid Pattern of Stars ---"); for (int i = 1 ; i <= rows; i++ ) { for (int j = 0 ; j < rows - i; j++ ) { System.out.print(" "); } for (int k = 0 ; k < (i * 2) - 1; k++ ) { System.out.print("*"); } System.out.println(); } } }
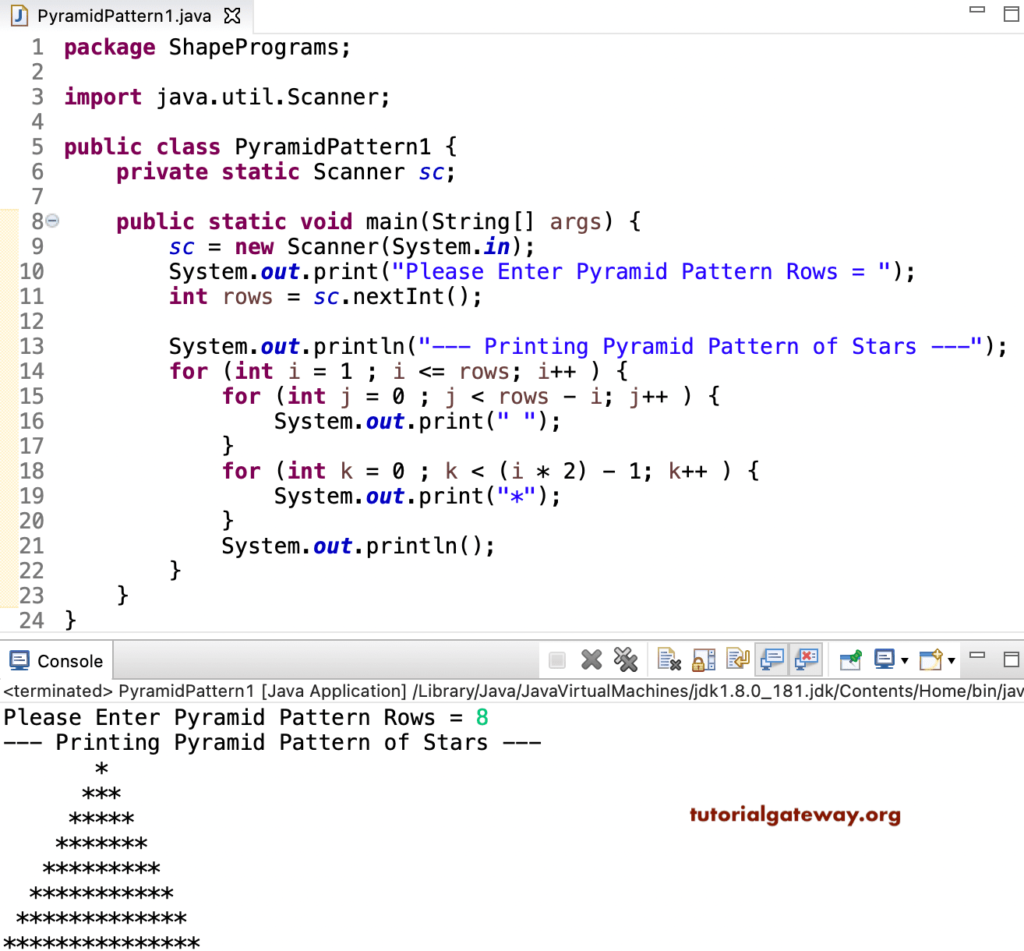
In this Java pyramid star pattern program, we replaced the for loops with whiles.
package ShapePrograms; import java.util.Scanner; public class PyramidPattern2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Pyramid Pattern Rows = "); int rows = sc.nextInt(); System.out.println("--------"); int i = 1 ; while( i <= rows) { int j = 0 ; while(j < rows - i ) { System.out.print(" "); j++; } int k = 0 ; while(k < (i * 2) - 1) { System.out.print("*"); k++; } System.out.println(); i++; } } }
Please Enter Pyramid Pattern Rows = 9
--------
*
***
*****
*******
*********
***********
*************
***************
*****************
Java Print Pyramid Star Pattern using do while loop
package ShapePrograms; import java.util.Scanner; public class PyramidPattern3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Pyramid Pattern Rows = "); int rows = sc.nextInt(); System.out.println("--------"); int j, k, i = 1; do { j = 0; do { System.out.print(" "); }while(j++ < rows - i ); k = 0; do { System.out.print("*"); }while(++k < (i * 2) - 1); System.out.println(); }while(++i <= rows); } }
Please Enter Pyramid Pattern Rows = 10
--------
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
In this example, the PyramidPattern function prints the pyramid pattern of a given symbol.
package ShapePrograms; import java.util.Scanner; public class PyramidPattern4 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Rows = "); int rows = sc.nextInt(); System.out.print("Please Enter Character for Pyramid Pattern = "); char ch = sc.next().charAt(0); System.out.println("--------"); PyramidPattern(rows, ch); } public static void PyramidPattern(int rows, char ch) { for (int i = 1 ; i <= rows; i++ ) { for (int j = 0 ; j < rows - i; j++ ) { System.out.print(" "); } for (int k = 0 ; k < (i * 2) - 1; k++ ) { System.out.print(ch); } System.out.println(); } } }
Please Enter Rows = 15
Please Enter Character for Pyramid Pattern = #
--------
#
###
#####
#######
#########
###########
#############
###############
#################
###################
#####################
#######################
#########################
###########################
#############################