Write a Java Program to Print Unique Array Items with an example. Or how to write a Java Program to print non repeated or unique items in a given array.
In this unique array elements example, we used unqArr array of the same size as org_arr. Next, we assigned the unique items to this unqArr within the for loop.
package ArrayPrograms; import java.util.Scanner; public class UniqueArrayItems { private static Scanner sc; public static void main(String[] args) { int size, i, j, count; sc = new Scanner(System.in); System.out.print("\n Please Enter the Unique Array size :"); size = sc.nextInt(); int[] org_arr = new int[size]; int[] unqArr = new int[size]; System.out.format("\nEnter Unique Arrays %d Items : ", size); for(i = 0; i < size; i++) { org_arr[i] = sc.nextInt(); unqArr[i] = -1; } for(i = 0; i < size; i++) { count = 1; for(j = i + 1; j < size; j++) { if(org_arr[i] == org_arr[j]) { count++; unqArr[j] = 0; } } if(unqArr[i] != 0) { unqArr[i] = count; } } System.out.println("\nList of Unique Items in this Array :"); for(i = 0; i < size; i++) { if(unqArr[i] == 1) { System.out.format("%d ", org_arr[i]); } } } }
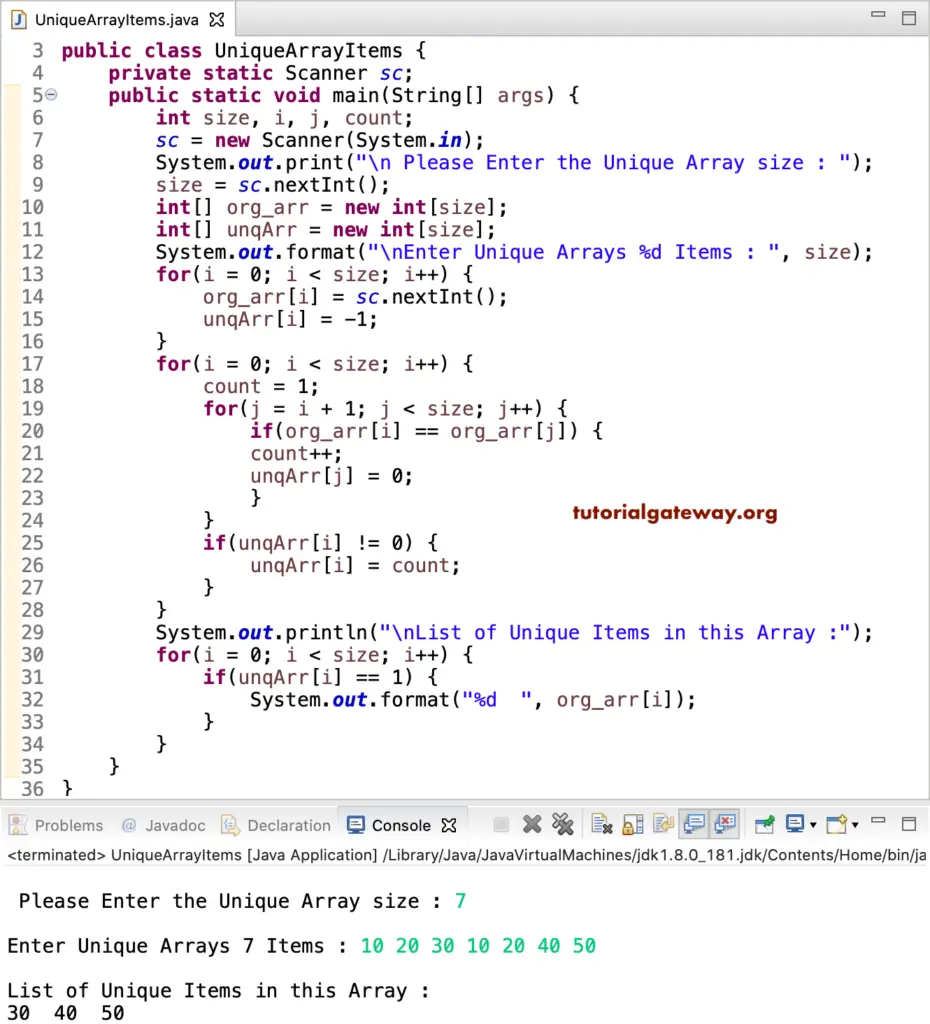
Java Program to Print Unique Array Items using Functions
In this Java unique array items example program, we created a separate function UniqueArrayElement to find and print the unique array.
package ArrayPrograms; import java.util.Scanner; public class UniqueArrayItems2 { private static Scanner sc; public static void main(String[] args) { int size, i; sc = new Scanner(System.in); System.out.print("\n Please Enter the size :"); size = sc.nextInt(); int[] org_arr = new int[size]; int[] unqArr = new int[size]; System.out.format("\nEnter %d Items : ", size); for(i = 0; i < size; i++) { org_arr[i] = sc.nextInt(); unqArr[i] = -1; } UniqueArrayElement(org_arr, unqArr, size); } public static void UniqueArrayElement(int[] org_arr, int[] unqArr, int size) { int i, j, count; for(i = 0; i < size; i++) { count = 1; for(j = i + 1; j < size; j++) { if(org_arr[i] == org_arr[j]) { count++; unqArr[j] = 0; } } if(unqArr[i] != 0) { unqArr[i] = count; } } System.out.println("\nList of Unique Items in this Array :"); for(i = 0; i < size; i++) { if(unqArr[i] == 1) { System.out.format("%d ", org_arr[i]); } } } }
Unique Array Items
Please Enter the size :11
Enter 11 Items : 10 20 30 10 40 20 50 90 60 70 30
List of Unique Items in this Array :
40 50 90 60 70