Write a Java Program to Print Square Number Pattern using For Loop, and While Loop with example.
Java Program to Print Square Number Pattern using For Loop
This Java program allows entering any side of a square (all sides are equal). Next, this Java program displays a square number pattern of 1’s until it reaches to the user-specified rows and columns.
// Java Program to Print Square Number Pattern using For loop import java.util.Scanner; public class SquareNumber1 { private static Scanner sc; public static void main(String[] args) { int side, i, j; sc = new Scanner(System.in); System.out.print(" Please Enter any Side of a Square : "); side = sc.nextInt(); for(i = 0; i < side; i++) { for(j = 0; j < side; j++) { System.out.print("1 "); } System.out.print("\n"); } } }
Java Square Number Pattern using For Loop output
Please Enter any Side of a Square : 12
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 1 1 1 1
First, the For loop is to iterate from 1 to user entered side. Next, we used Nested For Loop to iterate j from 1 to user-entered value (side)
User entered value: side = 8
First For Loop – First Iteration: for(i = 0; i < 8; i++)
Condition is True. So, it enters into second For Loop
Second For Loop – First Iteration: for(j = 0; 1 < 8; 0++)
Condition is True. So, 1 inside the Java system.out.println printed.
Second For Loop – Second Iteration: for(j = 1; 1 < 8; 1++)
Condition is True. So, 1 inside the system.out.println printed.
Second For Loop repeats the process until j reaches 8. Because if j is 8, the condition fails so, it exits from the second loop.
First For Loop – Second Iteration: for(i = 1; i < 8; 1++)
Condition is True. So, it enters into second For Loop. Repeat the above process until i reaches to 8.
Java Program to Print Square Number Pattern using While Loop
This Java program to return square number pattern is the same as the above example, but we are using the While Loop.
// Java Program to Print Square Number Pattern using While loop import java.util.Scanner; public class SquareNumber2 { private static Scanner sc; public static void main(String[] args) { int side, i = 0, j; sc = new Scanner(System.in); System.out.print(" Please Enter any Side of a Square : "); side = sc.nextInt(); while(i < side) { j = 0; while(j < side) { System.out.print("1"); j++; } i++; System.out.print("\n"); } } }
Java Square Number Pattern using a While Loop output
Please Enter any Side of a Square : 10
1111111111
1111111111
1111111111
1111111111
1111111111
1111111111
1111111111
1111111111
1111111111
1111111111
Java Square Number Pattern Program Example 3
This program allows the user to enter any integer value, and then prints that integer in a square pattern.
// Java Program to Print Square Number Pattern import java.util.Scanner; public class SquareNumber3 { private static Scanner sc; public static void main(String[] args) { int side, number, i, j; sc = new Scanner(System.in); System.out.print(" Please Enter any Side of a Square : "); side = sc.nextInt(); System.out.print(" Please Enter any Integer Value : "); number = sc.nextInt(); for(i = 0; i < side; i++) { for(j = 0; j < side; j++) { System.out.print(number); } System.out.print("\n"); } } }
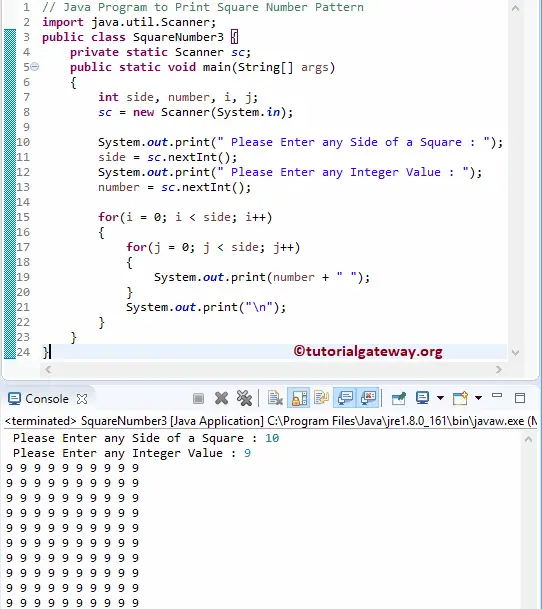