The Java For loop is used to repeat a block of statements with the given number of times until the given condition is False. The for loop is one of the most used ones in Java or any other programming language. Let us see the syntax:
In the Java for loop, there are three expressions separated by the semi-colons (;) and the execution of these expressions is as follows:
- Initialization: It starts with the initialization statement. So, counter variables are initialized first (Example counter = 1 or i = 1). The initialization section is executed only once at the beginning.
- Test Condition: The value of the counter variable will test against the test condition. If the result evaluates to True, the compiler will execute the statements inside the body of the Java for loop. If the condition is false, it will terminate.
- Increment and decrement operator: This expression will run after the end of each iteration. This operator helps to increase or decrease the counter variable of for loop as per our requirement.
Java For loop Syntax
The syntax of the For Loop in Java Programming language is as follows:
for (initialization; test condition; increment/decrement operator) { Statement 1 Statement 2 ……… Statement n }
Java For Loop initialization
The Java For loop has the flexibility to omit one or more sections from the declaration. Although we can skip one or more sections from the declaration, we must put the semicolon (;) in place; otherwise, it will throw a compilation error.
Initialize the counter variable can skip from Java for loop, as shown below:
int i = 1; for( ; i <= 20; i++)
If you observe the above code snippet, the counter variable is declared in the previous line. Like the initialization condition, we can also skip the increment part of the Java For loop.
int i = 1; for( ; i <= 10; ) { //statements i++; }
If you observe the above Java for loop code snippet, the increment part is declared in the body. It also allows us to initialize more than one counter variable at a time with comma separate:
for(i = 1,j = 10; i < j; i++)
The Java For loop also allows using multiple conditions. Instead of using a comma, we have to use the logical operator to separate the two conditions.
for(i=1,j=20; i <= 10 && j >= 20; i++) { //statements j++; }
Like the test condition, Java for loop allows us to use more than one increment operator as follows.
for(i = 1, j = 1; i <= 10 && j <= 10; i++, j++)
Infinite For loop
for( ; ; )
The increment and decrement operator section initialization uses a comma to separate multiple declarations. To separate the test conditions, you must use logical operators to join conditions.
Java For loop Flow Chart
The screenshot below will show the flow chart of For Loop in Java Programming language.
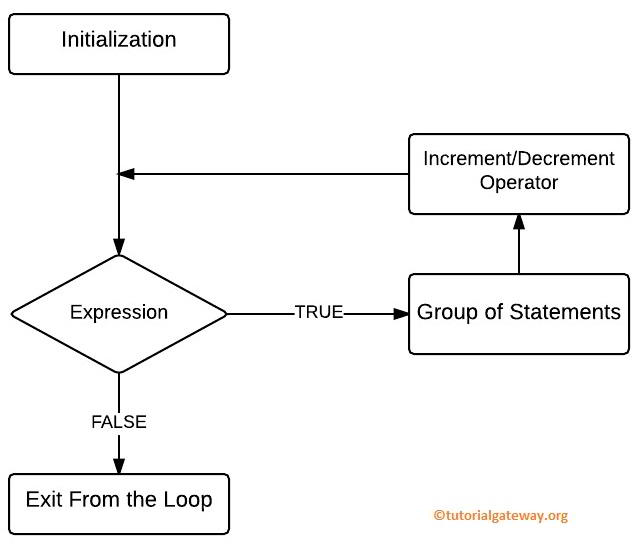
The execution process of Java for loop is:
- Initialization: We initialize the counter variable(s) here.
- Test condition: It will check the condition against the counter variable. If the condition is True, the compiler will execute the statements inside. If the condition is False, then it will exit.
- After completing the iteration, it will execute the Increment and Decrement Operator inside the Java for loop to increment or decrement the value.
- Again it will check the expression after the value is incremented. The statements inside this will execute as long as the condition is true.
Java For Loop example
This program allows the user to enter any integer values. Then it will calculate the sum of natural numbers up to the user’s entered number using the Java for loop.
package Loops; import java.util.Scanner; public class ForLoop { private static Scanner sc; public static void main(String[] args) { int i, number, sum = 1; sc = new Scanner(System.in); System.out.println("\n Please Enter the any integer Value: "); number = sc.nextInt(); for (i = 1; i <= number; i++) { sum = sum * i; } System.out.format(" Sum of the Numbers From the is: %d ", sum); } }
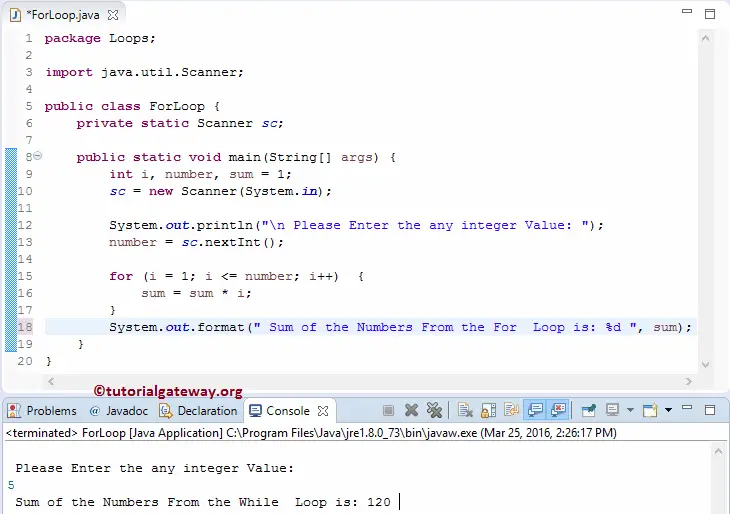
The first statements in this program will ask the user to enter any integer value below 10. Next, we assign the user entered value to the integer variable (number)
Next line, we use the Java For loop, and the Condition inside it will make sure that the value of i is less than or equal to the user-specified Number.
for(i = 1; i <= number; i++) { sum = sum * i; }
In this example, User Entered value: Number = 5 and we initialized the sum = 0
First Iteration
Within this, we initialized the i value as 1. Remember, Initialization happens only once. Next, the compiler will check the expression (i <= number), which is TRUE. So, the statement or block of code inside it will execute.
sum = sum * i
sum = 1 * 1 ==> 1
Next, i value will incremented by 1 (i++). Please refer to Increment and Decrement Operators article to understand this ++ notation.
Java for loop Second Iteration
Within the first Iteration, the values of i changed as i = 2. Next, it will evaluate the condition (i <= number), which is TRUE. So, the statement inside it will execute.
sum= 1 * 2 = 2
Next, the number will increment by 1 (number ++).
Third Iteration
Within the second Iteration, the values of both i and sum changed as i = 3 and sum = 2. Next, the compiler will check the condition (i <= number), which is TRUE.
sum = 2 * 3 = 6
Fourth Iteration
Within the third Iteration, the values of i = 4 and sum = 6. Next, the compiler will check the condition (i <= number), which is TRUE. So, the statement inside it will execute.
sum = 6 * 4 = 24
Fifth Iteration
Within the fourth Iteration, the values of i = 5 and sum = 24.
sum = 24 * 5 = 120
I = 6. So, the condition present in the code ( 6 <= 5) evaluates to false.
The last System statement will print the number of digits present in the given number as output.
Nested For Loop in Java
Placing For Loop inside another is called Nested For Loop in Java Programming. When working with multi-layered data, use these Java Nested For loops to extract the layered data, but please be careful while using it.
For example, when you are working with a single-dimensional Array, you can use Java For Loop to iterate from starting to array end. However, to work with Two Dimensional array or Multi-Dimensional Array, you have to use this Nested For Loop in Java. Before we get into the example, let us see the syntax of the Nested For loop.
Java nested for loop Syntax
The syntax of the Nested For Loop in Java Programming language is as follows:
for (initialization; test condition; increment/decrement operator) { //First for (initialization; test condition; increment/decrement operator) { // Second //Second For Loop Statements Statement 1 Statement 2 ……… Statement n } //First For Loop Statements Statement 1 ……… Statement n }
If you observe the above Java nested loop syntax, we placed the For loop inside another.
We have already explained the for loop syntax in our previous article. So, please refer to Java For Loop article to understand the loop functionality. Let me explain the details of this Java Nested For Loop Syntax.
Step 1: First, the compiler will check for the condition inside the first for loop.
- If the condition is True, then statements inside the For loop will be executed. It means the compiler will enter into the second For loop: Goto, Step 2.
- If the condition is False, then the compiler will exit from For Loop.
Step 2: Java compiler will check for the condition inside the second or nested for loop.
- If the condition is True, statements inside the second For loop will execute. It means it will execute from Statement 1 to N.
- If the condition is False, the compiler will exit from the second For Loop.
3rd Step: Once it exits from the second for loop, the compiler will check for the condition inside the for loop (repeating Step 1 ).
Nested For Loop in Java Example
This Nested for loop Java program allows the user to enter any integer values. Then it will print the Multiplication table from the user-specified number to 10. To do this, we are going to nest one for loop inside another for loop. This is also called nested for loop in java programming.
// example package Loops; import java.util.Scanner; public class NestedForLoop { private static Scanner sc; public static void main(String[] args) { int i, j; sc = new Scanner(System.in); System.out.println("Please Enter the any integer Value below 10: "); i = sc.nextInt(); for (; i <= 10; i++) { for (j = 1; j <= 10; j++) { System.out.format("%d * %d = %d\n",i ,j, i*j); } System.out.println(); } } }
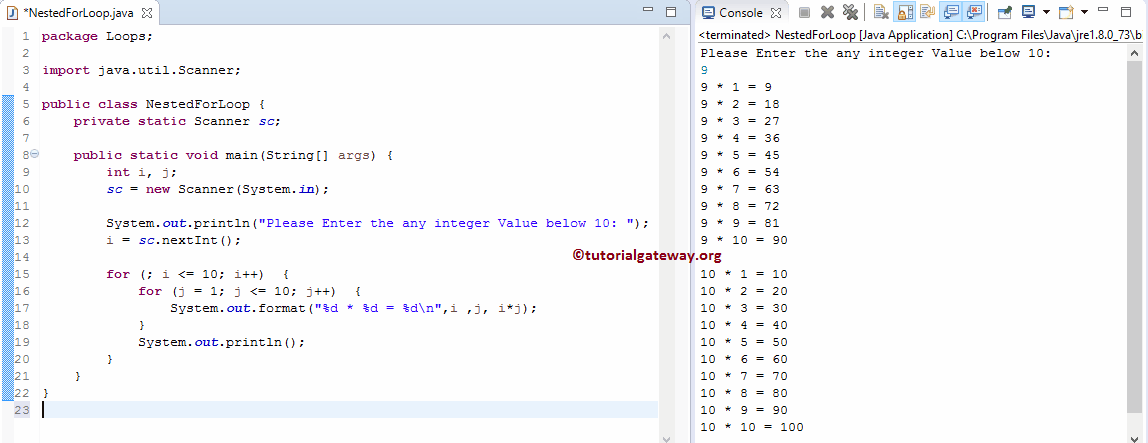
In this example, the following statements ask you to enter any integer value below 10. Next, we assign the user entered value to integer variable (i)
System.out.println("Please Enter the any integer Value below 10: "); i = sc.nextInt();
Next line, we used the Java Nested For loop, and the Condition inside the First For loop will make sure that the user-specified value i is less than or equal to 10.
for (; i <= 10; i++) { for (j = 1; j <= 10; j++) { System.out.format("%d * %d = %d\n",i ,j, i*j); } System.out.println(); }
From the above screenshot, you can observe that the User Entered value i: = 9. It means this Nested For Loop program prints the multiplication table for 9 and 10.
First For Loop First Iteration
In the first for loop, i initialized to value 9, and then it will check whether i is less than or equal to 10. This condition is True, so it will enter into the second for loop.
Second For Loop First Iteration
It is the Nested For loop in Java. In the second for loop j is initialized to value 1. Next, it will check whether j is less than or equal to 10. This condition is True, so the Javac compiler will execute the statements inside the second for loop.
i * j ==> 9 * 1 = 9
Next, j value will increment by 1 (j++). Please refer to Increment and Decrement Operators in Java article to understand this ++ notation.
Second For Loop Second Iteration
Here, j is incremented by 1. So, J =2. The compiler will check whether j is less than or equal to 10. This condition is True so that the compiler will execute the statements inside the second for loop.
i * j ==> 9 * 2 = 18
Next, j value will increment by 1 (j++).
This process will repeat until j reaches 11. Once the condition inside the second for loop fails, the compiler will exit from the second for loop, and i will increment by 1 (i++). Next,
First For Loop Second Iteration
Here, i is incremented by 1, so i =10. The compiler will check whether i is less than or equal to 10. This condition is True, so the Javac compiler will enter into the second for loop
Second For Loop First Iteration
In the second for loop, j initializes to value 1, and then it will check whether j is less than or equal to 10. This condition is True. So, the compiler will execute the statements inside the second for loop.
i * j ==> 10 * 1 = 10
Next, j value will increment by 1 (j++).
Second For Loop Second Iteration – nested for loop in java
Here, j will increment by 1, so J =2. The compiler will check whether j is less than or equal to 10. This condition is True so that the compiler will execute the statements inside the second for loop.
i * j ==> 10 * 2 = 20
Next, j value will increment by 1 (j++).
This process will repeat until j reaches to 11. Once the condition inside the second for loop fails, the compiler will exit from the second for loop, and i will increment by 1 (i++). Next,
First For Loop Third Iteration:
Here, i = 11, and the condition is False. For loop is terminated, No need to check the second loop, i.e., nested.