Write a Java Program to Print Rectangle Star Pattern using For Loop, and While Loop with example.
Java Program to Print Rectangle Star Pattern using For Loop
This Java program allows the user to enter the number of rows and column values. Next, this program prints the stars in a rectangle pattern until it reaches the user-specified rows and columns.
// Java Program to Print Rectangle Star Pattern import java.util.Scanner; public class RectangleStar1 { private static Scanner sc; public static void main(String[] args) { int rows, columns, i, j; sc = new Scanner(System.in); System.out.print(" Please Enter Number of Rows : "); rows = sc.nextInt(); System.out.print(" Please Enter Number of Columns : "); columns = sc.nextInt(); for(i = 1; i <= rows; i++) { for(j = 1; j <= columns; j++) { System.out.print("* "); } System.out.print("\n"); } } }
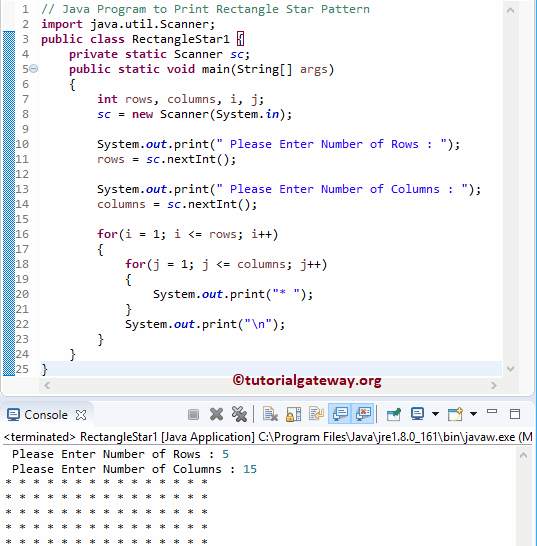
The first For loop is to iterate from 1 to user-entered rows. Next, we used Nested For Loop to iterate j from 1 to user given column value
User entered value: Rows = 5 and Columns = 15
First For Loop – First Iteration: for(i = 1; i <= 5; i++)
Condition is True. So, it enters into second For Loop
Second For Loop – First Iteration: for(j = 1; 1 <= 15; 1++)
Condition is True. So, * inside the Java system.out.println printed.
Second For Loop – Second Iteration: for(j = 2; 2 <= 15; 2++)
Condition is True. So, * inside the system.out.println printed.
The second For Loop repeats the process until j reaches to 16. Because, if j is 16, the condition fails. So, it exits from the second loop.
First For Loop – Second Iteration: for(i = 2; i <= 5; 2++)
Condition is True. So, it enters into second For Loop. Repeat the above process until i reaches to 6.
Java Program to Print Rectangle Star Pattern using While Loop
This Java program to display rectangular star pattern is the same as the above example, but we are using the While Loop.
// Java Program to Print Rectangle Star Pattern import java.util.Scanner; public class RectangleStar2 { private static Scanner sc; public static void main(String[] args) { int rows, columns, i = 1, j; sc = new Scanner(System.in); System.out.print(" Please Enter Number of Rows : "); rows = sc.nextInt(); System.out.print(" Please Enter Number of Columns : "); columns = sc.nextInt(); while(i <= rows) { j = 1; while(j <= columns) { System.out.print("*"); j++; } i++; System.out.print("\n"); } } }
Java Rectangle Pattern using While Loop output
Please Enter Number of Rows : 12
Please Enter Number of Columns : 30
Please Enter any Character : @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @ @
Java Program to Print Rectangle Pattern of Custom Character
This program allows the user to enter any character and then prints that character in a rectangle pattern.
// Java Program to Print Rectangle Star Pattern import java.util.Scanner; public class RectangleStar3 { private static Scanner sc; public static void main(String[] args) { int rows, columns, i, j; char ch; sc = new Scanner(System.in); System.out.print(" Please Enter Number of Rows : "); rows = sc.nextInt(); System.out.print(" Please Enter Number of Columns : "); columns = sc.nextInt(); System.out.print(" Please Enter any Character : "); ch = sc.next().charAt(0); for(i = 1; i <= rows; i++) { for(j = 1; j <= columns; j++) { System.out.print(ch + " "); } System.out.print("\n"); } } }
Please Enter Number of Rows : 15
Please Enter Number of Columns : 22
Please Enter any Character : *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * *