Write a Java Program to Print Negative Array Numbers with an example. Or how to write a Java Program to find and return the negative items in a given array. In this Java count negative array numbers example, we used while loop to iterate count_NegArr array and count negative items (a number less than zero), and prints the same.
package ArrayPrograms; public class NegativeArrayItems { public static void main(String[] args) { int j = 0; int[] Neg_arr = {-40, 15, -4, 11, -8, -13, 22, 16, -11, -99, 55, 18, -60}; System.out.print("\nList of Negative Numbers in NEG Array : "); while(j < Neg_arr.length) { if(Neg_arr[j] < 0) { System.out.format("%d ", Neg_arr[j]); } j++; } } }
Java Negative Array Numbers using a while loop output
List of Negative Numbers in NEG Array : -40 -4 -8 -13 -11 -99 -60
Java Program to Print Negative Array Numbers using For Loop
This Java negative array item example allows the user to enter the Neg_arr array size and items.
package ArrayPrograms; import java.util.Scanner; public class NegativeArrayItems1 { private static Scanner sc; public static void main(String[] args) { int Size, i; sc = new Scanner(System.in); System.out.print("\nPlease Enter the NEG Array size : "); Size = sc.nextInt(); int[] Neg_arr = new int[Size]; System.out.format("\nEnter NEG Array %d elements : ", Size); for(i = 0; i < Size; i++) { Neg_arr[i] = sc.nextInt(); } System.out.print("\nList of Negative Numbers in NEG Array : "); for(i = 0; i < Size; i++) { if(Neg_arr[i] < 0) { System.out.format("%d ", Neg_arr[i]); } } } }
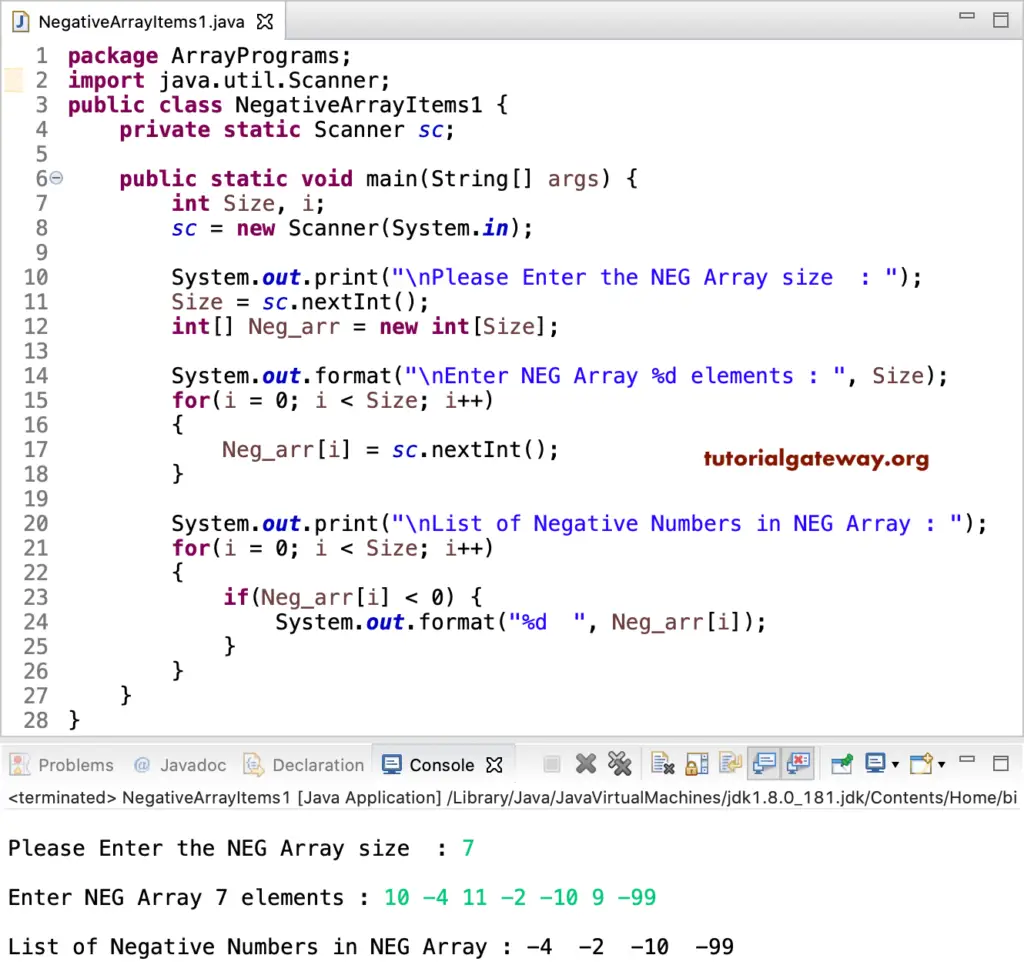
In this negative array item Java example, we created a separate function NegativeElement to print the negative array items in the given array.
package ArrayPrograms; import java.util.Scanner; public class NegativeArrayItems2 { private static Scanner sc; public static void main(String[] args) { int Size, i; sc = new Scanner(System.in); System.out.print("\nPlease Enter the NEG Array size : "); Size = sc.nextInt(); int[] Neg_arr = new int[Size]; System.out.format("\nEnter NEG Array %d elements : ", Size); for(i = 0; i < Size; i++) { Neg_arr[i] = sc.nextInt(); } NegativeElement(Neg_arr, Size); } public static void NegativeElement(int[] Neg_arr, int size ) { int i; System.out.print("\nList of Negative Numbers in NEG Array : "); for(i = 0; i < size; i++) { if(Neg_arr[i] < 0) { System.out.format("%d ", Neg_arr[i]); } } } }
Java Negative Array Items using For Loop and functions output
Please Enter the NEG Array size : 9
Enter NEG Array 9 elements : -1 5 0 11 -2 -3 99 3 -5
List of Negative Numbers in NEG Array : -1 -2 -3 -5