Write a Java program to perform Arithmetic operations on Matrix with an example. Or write a Java program to perform addition, subtraction, multiplication, division, and modules on Matrix or Multidimensional array.
In this Java Matrix Arithmetic operations example, we declared two matrixes. Next, we used the For Loop to iterate the matrix items. We performed matrix Arithmetic operations on x and y matrixes within that loop and printed the same as the output.
public class MatrixArithmeticOperations { public static void main(String[] args) { int[][] x = {{15, 25, 35}, {45, 55, 65}, {75, 85, 95}}; int[][] y = {{ 2, 4, 5}, {6, 8, 5}, {10, 15, 20}}; int[][] sum = new int[3][3]; int i, j; System.out.println("\nAdd\tSub\tMul\tDiv\tMod"); for(i = 0; i < x.length; i++) { for(j = 0; j < x[0].length; j++) { System.out.format("%d \t", (x[i][j] + y[i][j])); System.out.format("%d \t", (x[i][j] - y[i][j])); System.out.format("%d \t", (x[i][j] * y[i][j])); System.out.format("%d \t", (x[i][j] / y[i][j])); System.out.format("%d \t", (x[i][j] % y[i][j])); System.out.println(""); } System.out.println(""); } } }
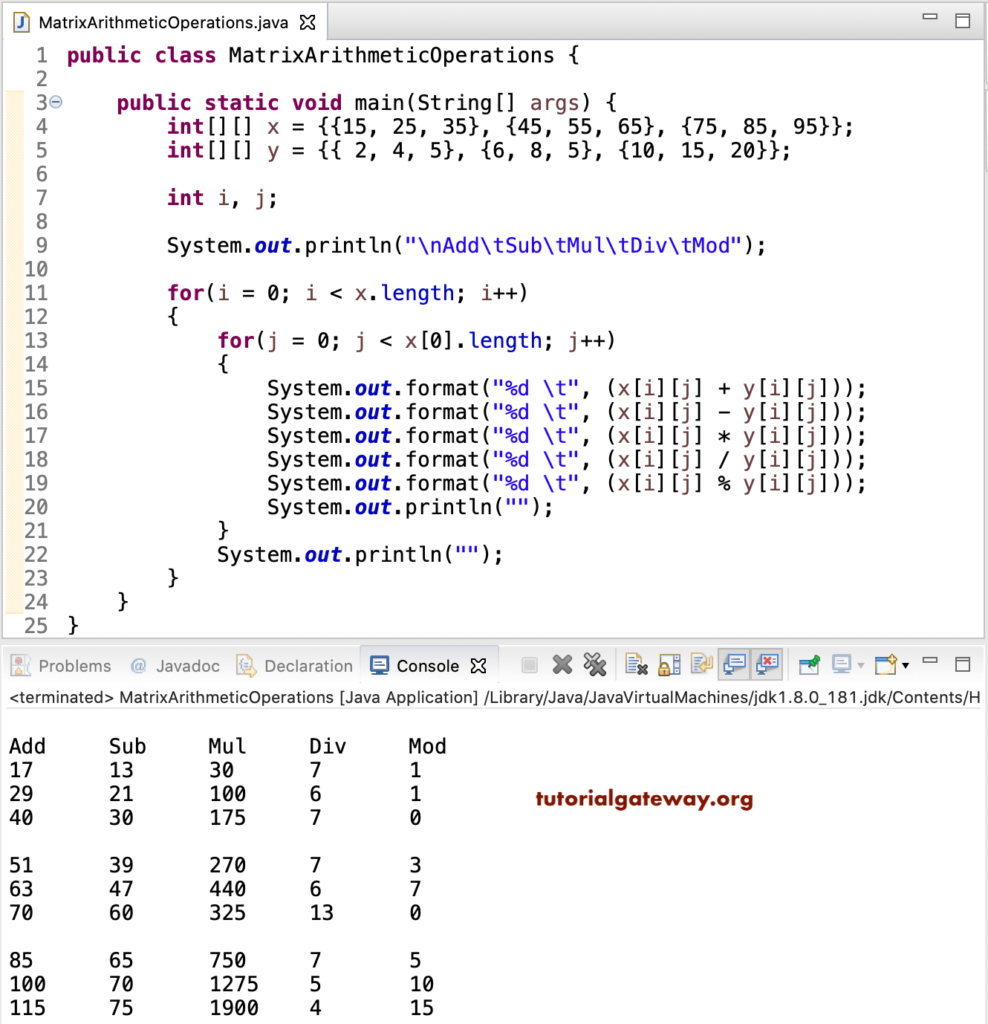
Java program to perform Arithmetic operations on Matrix example 2
This Java matrix Arithmetic operations logical code is the same as above. However, this Java code allows the user to enter the rows, columns of the matrices. Next, it reads the user inputs for both the matrices and then performs the Arithmetic operations. Please refer to the C program to perform Arithmetic operations on Matrix to understand the Arithmetic operator’s code analysis in iteration wise.
import java.util.Scanner; public class MatrixArithmeticOperations { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns; sc= new Scanner(System.in); System.out.println("\n Please Enter Matrix Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr1 = new int[rows][columns]; int[][] arr2 = new int[rows][columns]; int[][] add = new int[rows][columns]; int[][] sub = new int[rows][columns]; int[][] mul = new int[rows][columns]; int[][] div = new int[rows][columns]; int[][] mod = new int[rows][columns]; System.out.println("\n Please Enter the First Matrix Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr1[i][j] = sc.nextInt(); } } System.out.println("\n Please Enter the Second Matrix Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr2[i][j] = sc.nextInt(); } } for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { add[i][j] = arr1[i][j] + arr2[i][j]; sub[i][j] = arr1[i][j] - arr2[i][j]; mul[i][j] = arr1[i][j] * arr2[i][j]; div[i][j] = arr1[i][j] / arr2[i][j]; mod[i][j] = arr1[i][j] % arr2[i][j]; } } System.out.println("\nAdd\t Sub\t Mul\t Div\t Mod"); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { System.out.format("%d \t", add[i][j]); System.out.format("%d \t", sub[i][j]); System.out.format("%d \t", mul[i][j]); System.out.format("%d \t", div[i][j]); System.out.format("%d \t", mod[i][j]); System.out.println(""); } System.out.println(" "); } } }
Java Matrix Arithmetic operations output
Please Enter Matrix Rows and Columns :
3 3
Please Enter the First Matrix Items :
10 20 30
40 50 60
70 80 90
Please Enter the Second Matrix Items :
2 4 6
8 9 5
3 2 1
Add Sub Mul Div Mod
12 8 20 5 0
24 16 80 5 0
36 24 180 5 0
48 32 320 5 0
59 41 450 5 5
65 55 300 12 0
73 67 210 23 1
82 78 160 40 0
91 89 90 90 0