Write a Java Program to find the Sum of the Matrix Lower Triangle with an example. We have already explained the steps to return a Matrix lower triangle in our previous example.
In this Java Sum of the Matrix Lower Triangle example, we declared an integer matrix. Next, we used for loop to iterate the Matrix. Within the for loop, we find the sum of the lower triangle of a given matrix.
public class SumOfLowerTriangle { public static void main(String[] args) { int i, j, sum = 0; int[][] Lt_arr = {{111, 21, 311}, {421, 511, 611}, {711, 811, 911}}; for(i = 0; i < 3 ; i++) { for(j = 0; j < 3; j++) { if(i > j) { sum = sum + Lt_arr[i][j]; } } } System.out.println("\n The Sum of the Lower Triangle Matrix = " + sum); } }
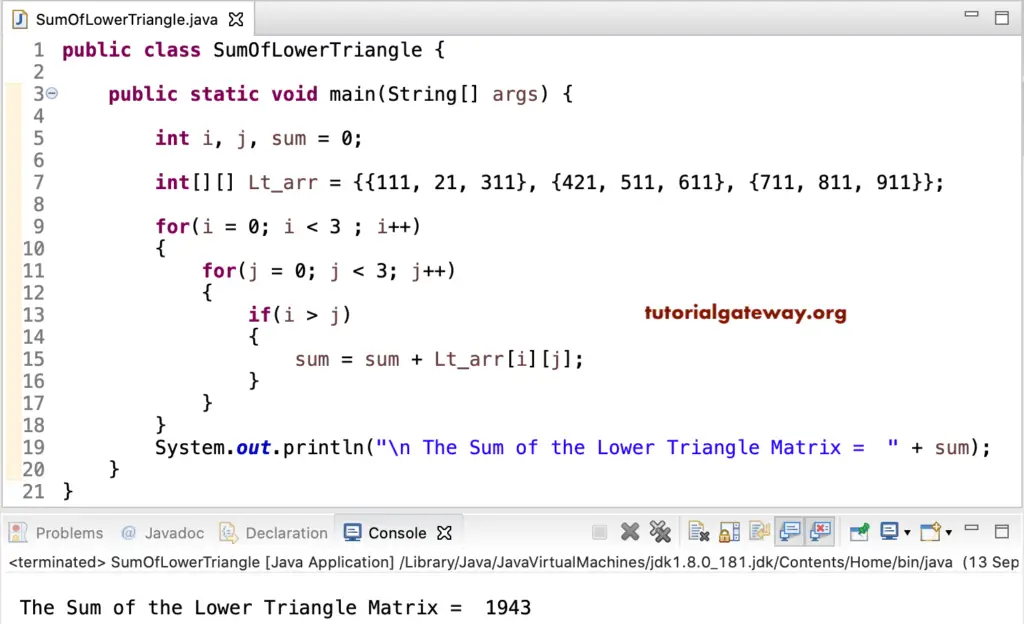
Java Program to find the Sum of the Matrix Lower Triangle example 2
This Java Lower Triangle Matrix Sum code is the same as the above. However, this Java code allows the user to enter the number of rows, columns, and the matrix items.
import java.util.Scanner; public class SumOfLowerTriangle { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns, sum = 0; sc= new Scanner(System.in); System.out.println("\n Please Enter LT Matrix Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr = new int[rows][columns]; System.out.println("\n Please Enter the LT Matrix Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr[i][j] = sc.nextInt(); } } for(i = 0; i < rows ; i++) { for(j = 0; j < columns; j++) { if(i > j) { sum = sum + arr[i][j]; } } } System.out.println("\n The Sum of Lower Triangle Matrix = " + sum); } }
Output of a Java Matrix Lower Triangle sum
Please Enter LT Matrix Rows and Columns :
3 3
Please Enter the LT Matrix Items :
10 20 30
40 50 60
70 80 90
The Sum of Lower Triangle Matrix = 190