Write a Java Program to display Matrix Lower Triangle with an example. A lower triangle is a square matrix whose items above the diagonal are zeros.
Java Program to display Matrix Lower Triangle
In this example, we declared an integer matrix. Next, we used for loop to iterate the two-dimensional array. Within the for loop, we used the If statement to check whether the rows index position is greater than or equal to the columns index position. Suppose it is true, the compiler prints the Matrix original value. Otherwise, it prints the values 0’s.
public class MatrixLowerTriangle { public static void main(String[] args) { int i, j; int[][] Lt_arr = {{11, 21, 31}, {41, 51, 61}, {71, 81, 91}}; System.out.println("\n---The Lower Triangle of the given Matrix---"); for(i = 0; i < 3 ; i++) { System.out.print("\n"); for(j = 0; j < 3; j++) { if(i >= j) { System.out.format("%d \t", Lt_arr[i][j]); } else { System.out.print("0 \t"); } } } } }
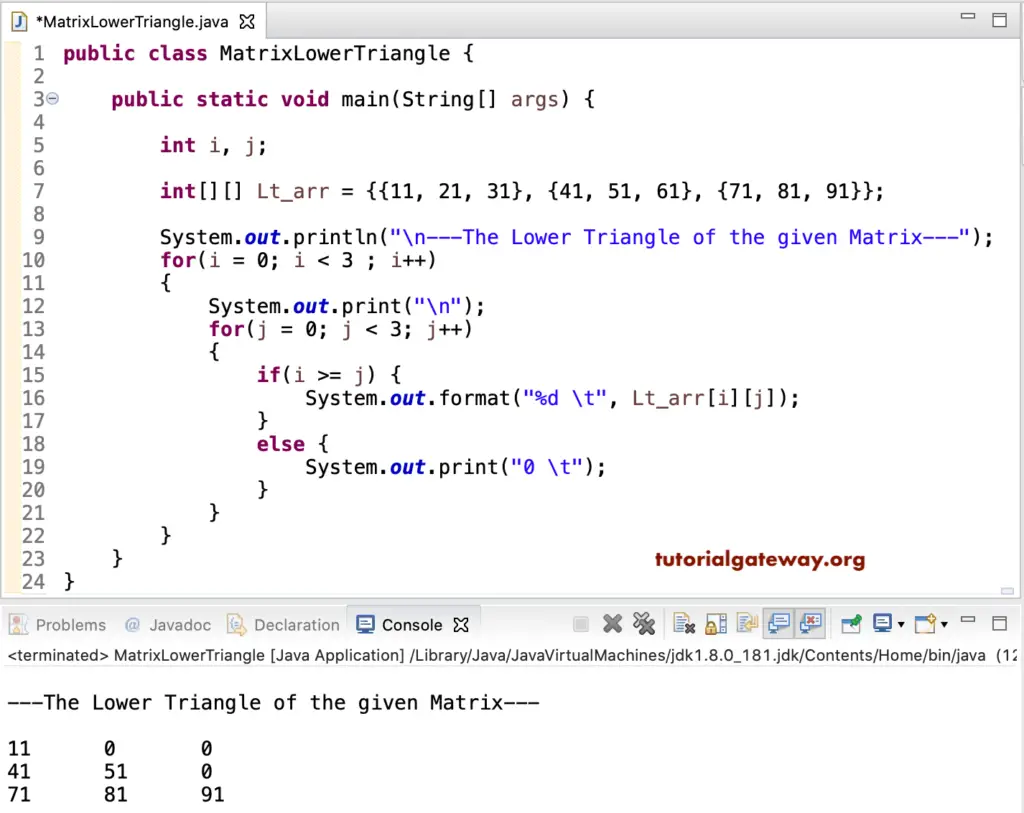
Java Program to display Matrix Lower Triangle using for loop
This Lower Triangle of a Matrix code is the same as the above. However, this Java code allows the user to enter the number of rows, columns, and items.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns; sc= new Scanner(System.in); System.out.println("\n Please Enter LT Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr = new int[rows][columns]; System.out.println("\n Please Enter the LT Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr[i][j] = sc.nextInt(); } } System.out.println("\n---The Lower Triangle of the given ---"); for(i = 0; i < rows ; i++) { System.out.print("\n"); for(j = 0; j < columns; j++) { if(i >= j) { System.out.format("%d \t", arr[i][j]); } else { System.out.print("0 \t"); } } } } }
Please Enter LT Rows and Columns :
3 3
Please Enter the LT Items :
10 20 99
33 55 88
77 89 44
---The Lower Triangle of the given ---
10 0 0
33 55 0
77 89 44