Write a Java Program to find Matrix is an Identity Matrix with an example. A Java Identity Matrix is a square matrix whose main diagonal items are 1’s, and all the other items are zeros.
In this Java Identity Matrix example, we declared a 3 * 3 integer matrix. Next, we used for loop to iterate the Matrix. Within the for loop, we used the If statement to check whether the diagonals are ones and the non-diagonal Matrix items are zeros. If it is true, we changed the Flag value to 0 and applied the break statement to exit from the loop. Next, we used the If Else statement to print the output based on the Flag value.
public class IdentityMatrix { public static void main(String[] args) { int i, j, Flag = 1; int[][] arr = {{1, 0, 0}, {0, 1, 0}, {0, 0, 1}}; for(i = 0; i < 3 ; i++) { for(j = 0; j < 3; j++) { if(arr[i][j] != 1 && arr[j][i] != 0) { Flag = 0; break; } } } if(Flag == 1) { System.out.println("\nMatrix is an Identity Matrix"); } else { System.out.println("\nMatrix is Not an Identity Matrix"); } } }
Java identity matrix output
Matrix is an Identity Matrix
Let me change the Matrix a bit and check for the Identity Matrix. int[][] arr = {{1, 0, 0}, {0, 1, 1}, {0, 0, 1}};
Matrix is Not an Identity Matrix
Java Program to find Matrix is an Identity Matrix example 2
This Java Identity Matrix code is the same as the above. However, this Java code allows the user to enter the number of rows, columns, and the matrix items.
import java.util.Scanner; public class IdentityMatrix { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns, Flag = 1; sc= new Scanner(System.in); System.out.println("\n Please Enter Identity Matrix Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr = new int[rows][columns]; System.out.println("\n Please Enter the Identity Matrix Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr[i][j] = sc.nextInt(); } } for(i = 0; i < rows ; i++) { for(j = 0; j < columns; j++) { if(arr[i][j] != 1 && arr[j][i] != 0) { Flag = 0; break; } } } if(Flag == 1) { System.out.println("\nMatrix is an Identity Matrix"); } else { System.out.println("\nMatrix is Not an Identity Matrix"); } } }
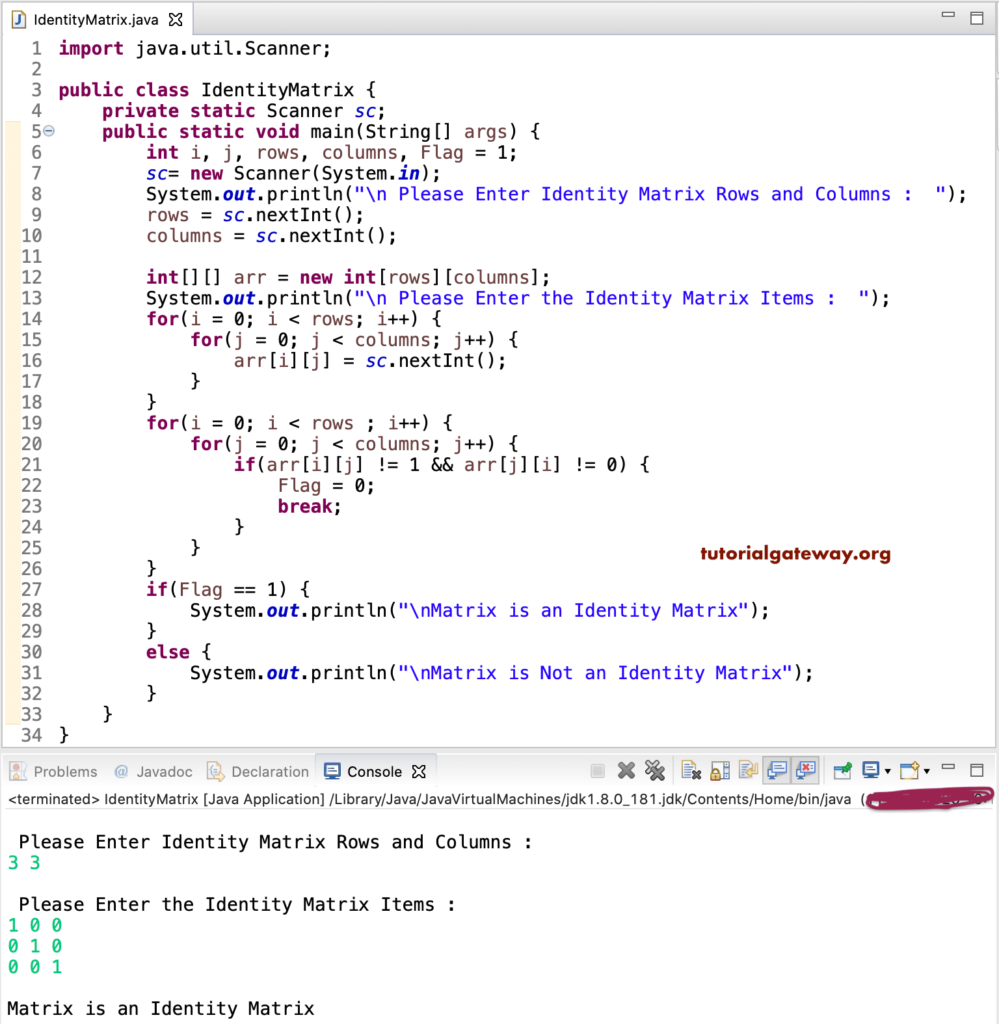
We can also use the Else If Statement to check whether the given matrix is an identity matrix. In this Java Identity Matrix example, we replaced the If statement in the above example with the Else If.
public class IdentityMatrix { public static void main(String[] args) { int i, j, Flag = 1; int[][] arr = {{1, 0, 0}, {0, 1, 0}, {0, 0, 1}}; for(i = 0; i < 3 ; i++) { for(j = 0; j < 3; j++) { if(i == j && arr[j][i] != 1) { Flag = 0; } else if(i != j && arr[j][i] != 0) { Flag = 0; } } } if(Flag == 1) { System.out.println("\nMatrix is an Identity Matrix"); } else { System.out.println("\nMatrix is Not an Identity Matrix"); } } }
Java identity matrix output
Matrix is an Identity Matrix
Here, we will try with wrong array. int[][] arr = {{1, 0, 0}, {0, 1, 1}, {0, 0, 1}};
Matrix is Not an Identity Matrix