Write a Java program to find the largest of two numbers using Else If Statement and Conditional Operator.
Java Program to Find largest of Two Numbers using Else If
This Java program allows the user to enter two different values. Next, this Java program finds the largest number among those two numbers using Else If Statement
// Java Program to Find largest of Two Numbers import java.util.Scanner; public class LargestofTwo { private static Scanner sc; public static void main(String[] args) { int number1, number2; sc = new Scanner(System.in); System.out.print(" Please Enter the First Number : "); number1 = sc.nextInt(); System.out.print(" Please Enter the Second Number : "); number2 = sc.nextInt(); if(number1 > number2) { System.out.println("\n The Largest Number = " + number1); } else if (number2 > number1) { System.out.println("\n The Largest Number = " + number2); } else { System.out.println("\n Both are Equal"); } } }
ANALYSIS
- The first Java if condition checks whether number1 is greater than number2. If this condition is True, number1 is greater than number2.
- The first Else if condition check whether number2 is greater than number1. If this condition is True, number2 is greater than number1.
- If the above two conditions fail, they are equal.
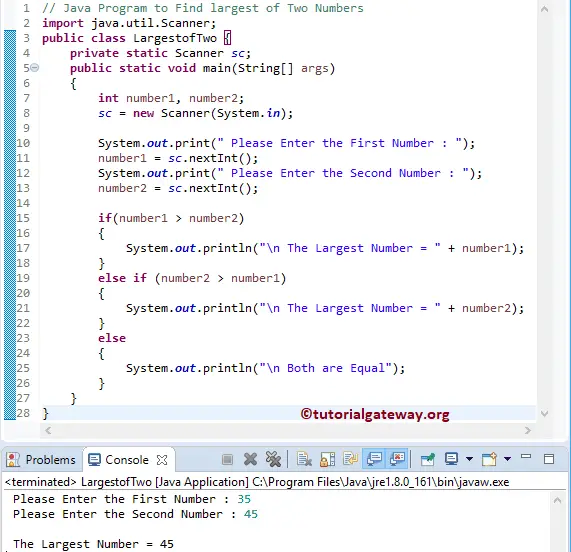
Java Program to Find largest of Two Numbers using Ternary Operator
This Java program returns the largest number among two numbers using Ternary Operator
// Java Program to Find largest of Two Numbers import java.util.Scanner; public class LargestofTwo1 { private static Scanner sc; public static void main(String[] args) { int number1, number2, largest; sc = new Scanner(System.in); System.out.print(" Please Enter the First Number : "); number1 = sc.nextInt(); System.out.print(" Please Enter the Second Number : "); number2 = sc.nextInt(); if(number1 == number2) { System.out.println("\n Both are Equal"); } else { largest = (number1 > number2)? number1: number2; System.out.println("\n The Largest Number = " + largest); } } }
ANALYSIS
- First, If condition checks whether number1 is equal to number2 or not if True, returns Both are Equal.
- Next, we used the Conditional Operator to check whether number1 is greater than number2. If this condition is True, it returns the first value after ? symbol, which is variable number1. If the first condition fails, return the value after the : symbol, which is variable number2.
Java largest of two numbers output 1
Please Enter the First Number : 45
Please Enter the Second Number : 22
The Largest Number = 45
OUTPUT 2
Please Enter the First Number : 45
Please Enter the Second Number : 45
Both are Equal
Java Program to find Largest of Two Numbers using Oops
In this example, we are dividing the code using the Object-Oriented Programming. To do this, First, we create a class that holds two methods to find the largest of two numbers.
// Class contains two methods to Find largest of Two Numbers public class LargestofTwoClass { int x, y, largest; public void LargestofTwo() { if(x == y) { System.out.println("\n Both are Equal"); } else { largest = (x > y)? x: y; System.out.println("\n LargestofTwo Method result : The Largest Number = " + largest); } } public int LargestofTwoNumber(int x, int y) { largest = (x > y)? x: y; return largest; } }
Within the Main program, we create an instance of the above-specified class and call the methods
// Java Program to Find largest of Two Numbers import java.util.Scanner; public class LargestofTwo2 { private static Scanner sc; public static void main(String[] args) { int number1, number2, largest; sc = new Scanner(System.in); System.out.print(" Please Enter the First Number : "); number1 = sc.nextInt(); System.out.print(" Please Enter the Second Number : "); number2 = sc.nextInt(); LargestofTwoClass ln = new LargestofTwoClass(); ln.x = number1; ln.y = number2; ln.LargestofTwo(); // Calling First Method largest = ln.LargestofTwoNumber(number1, number2); System.out.println("\n The Largest Number = " + largest); } }
Please Enter the First Number : 25
Please Enter the Second Number : 35
LargestofTwo Method result : The Largest Number = 35
The Largest Number = 35
LargestofTwo Class Analysis:
- First, we declared a function LargestOfTwo with zero arguments. Within the function, we used If Else statement to find largest of three numbers. And used the System.out.println statements to print the output as well.
- Next, we declared an integer function called LargestOfTwoNumbers with two arguments.
Main Class Analysis:
First we created an instance / created an Object of the LargestOfTwo Class
LargestofTwoClass ln = new LargestofTwoClass();
Next, we are assigning the user entered values to the LargestOfTwo Class variables.
ln.x = number1; ln.y = number2;
Next, we are calling the LargestOfTwo method. Note, this is the first method that we created with the void keyword. This method finds the largest of two, and print the output.
ln.LargestofTwo(); // Calling First Method
Next, we are calling the LargestOfTwoNumbers method by passing two arguments.
largest = ln.LargestofTwoNumber(number1, number2);
Lastly, System.out.println statement prints the output.
System.out.println("\n The Largest Number = " + largest);