Write a Java Program to Count Vowels and Consonants in a String using for loop, while loop, functions, and ASCII values with an example.
Java Program to Count Vowels and Consonants in a String using for loop
In this count of consonants and vowels in a string example, we first used for loop to iterate vowConsStr. Inside the loop, we assigned (ch = vowConsStr.charAt(i)) each character to ch to keep the code simple. Next, we used the Else If statement.
Within the if condition, we check whether the character is equal to a, e, i, o, u, A, E, I, O, and U. If true, we increment the value of the vowel. In the else if statement, we used (ch >= ‘a’ && ch <= ‘z’ || ch >= ‘A’ && ch <= ‘Z’) to check whether the character is an alphabet. If True, we increment the consonant value. Here, we can use else if (Character.isAlphabetic(ch)).
import java.util.Scanner; public class CountVowCons1 { private static Scanner sc; public static void main(String[] args) { String vowConsStr; int i, vowels, consonants; vowels = consonants = 0; char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter Vowel, Consonant String = "); vowConsStr = sc.nextLine(); for(i = 0; i < vowConsStr.length(); i++) { ch = vowConsStr.charAt(i); if(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U') { vowels++; } else if (ch >= 'a' && ch <= 'z' || ch >= 'A' && ch <= 'Z'){ consonants++; } } System.out.println("\nNumber of Vowel Characters = " + vowels); System.out.println("Number of Consonant Characters = " + consonants); } }
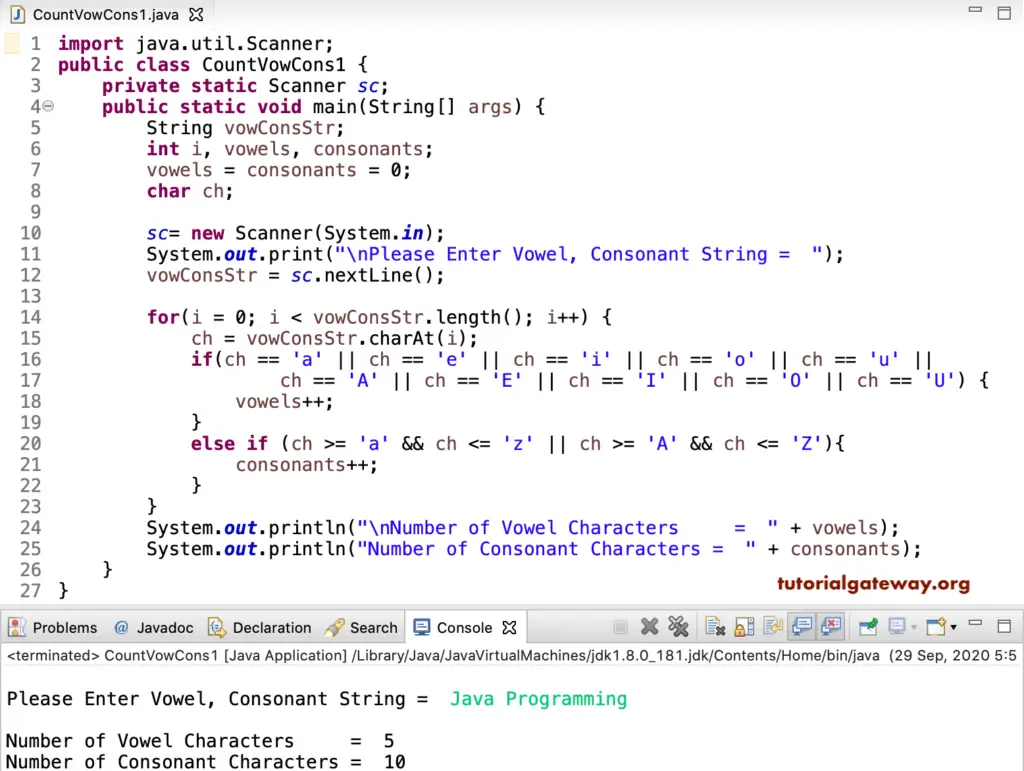
Java Program to Count Vowels and Consonants in a String using While Loop
The following toLowerCase() statement converts the given string to lowercase.
String lw_vowConsStr = vowConsStr.toLowerCase();
Instead of checking both uppercase and lowercase letters, we compare only lowercase and count the vowels and consonants.
import java.util.Scanner; public class CountVowCons2 { private static Scanner sc; public static void main(String[] args) { String vowConsStr; int i, vows, cons; i = vows = cons = 0; char ch; sc= new Scanner(System.in); System.out.print("\nPlease Enter String = "); vowConsStr = sc.nextLine(); String lw_vowConsStr = vowConsStr.toLowerCase(); while(i < lw_vowConsStr.length()) { ch = lw_vowConsStr.charAt(i); if(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') { vows++; } else if(ch >= 'a' && ch <= 'z') { cons++; } i++; } System.out.println("\nNumber of Vowel Characters = " + vows); System.out.println("Number of Consonant Characters = " + cons); } }
Please Enter String = Tutorial gateway
Number of Vowel Characters = 7
Number of Consonant Characters = 8
Using ASCII Values
In this Java Program example, we compare the ASCII values instead of comparing characters.
import java.util.Scanner; public class CountVowCons3 { private static Scanner sc; public static void main(String[] args) { String vowConsStr; int i, vows, const; vows = const = 0; int cp; sc= new Scanner(System.in); System.out.print("\nPlease Enter String = "); vowConsStr = sc.nextLine(); String up_vowConsStr = vowConsStr.toUpperCase(); for(i = 0; i < up_vowConsStr.length(); i++) { cp = up_vowConsStr.codePointAt(i); if(cp == 65 || cp == 69 || cp == 73 || cp == 79 || cp == 85) { vows++; } else if(cp >= 65 && cp <= 90 || cp >= 97 && cp <= 122) { const++; } } System.out.println("\nNumber of Vowel Characters = " + vows); System.out.println("Number of Consonant Characters = " + const); } }
Please Enter String = Hello World
Number of Vowel Characters = 3
Number of Consonant Characters = 7
Count Vowels and Consonants in a String using functions
This code to Count String Vowels and Consonants is the same as the above. Here, we separated the Vowels and Consonants logic using Java functions.
import java.util.Scanner; public class CountVowCons4 { private static Scanner sc; public static void main(String[] args) { String vowConsStr; sc= new Scanner(System.in); System.out.print("\nPlease Enter String = "); vowConsStr = sc.nextLine(); VowOrCons(vowConsStr.toLowerCase()); } public static void VowOrCons (String vowConsStr) { int i, vwl, cnts; vwl = cnts = 0; char ch; for(i = 0; i < vowConsStr.length(); i++) { ch = vowConsStr.charAt(i); if(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') { vwl++; } else if(ch >= 'a' && ch <= 'z') { cnts++; } } System.out.println("\nNumber of Vowel Characters = " + vwl); System.out.println("Number of Consonant Characters = " + cnts); } }
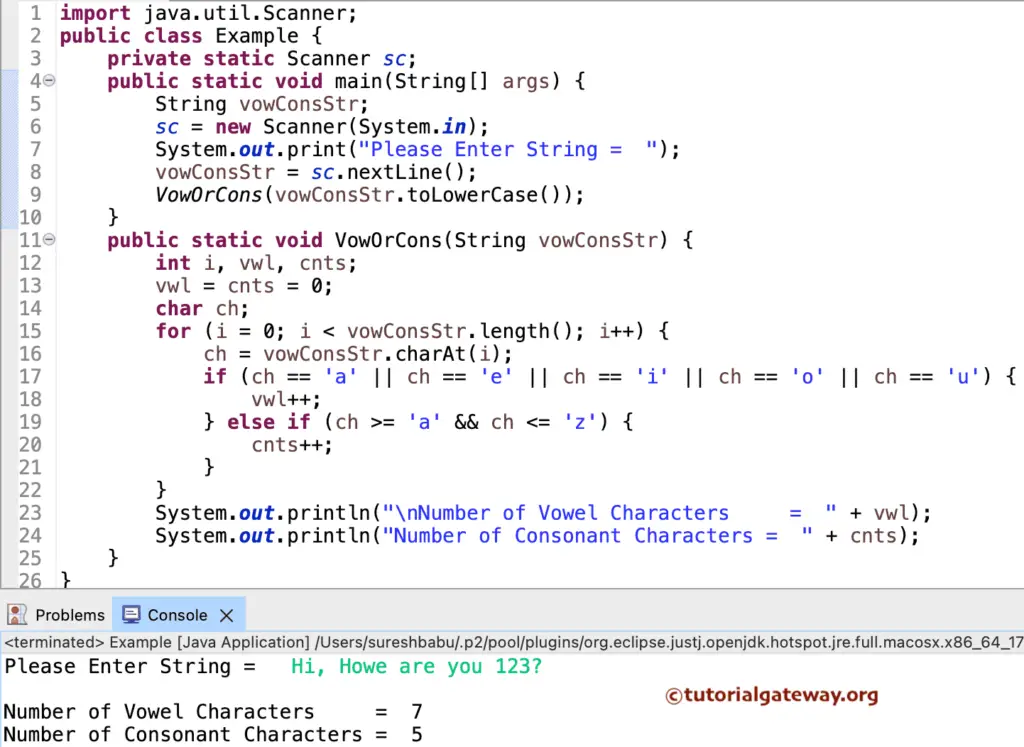