Write a Java Program to Calculate Electricity Bill using Else If statement with examples.
Java Program to Calculate Electricity Bill using Else If
This Java program asks the user to enter the units consumed. Next, it calculates the Total Electricity bill. This approach is useful if the Electricity board is charging different tariffs for different units. For this example, we are using the Else If Statement.
TIP: The Java Else If statement checks the first condition if it is TRUE, it executes the statements inside that block. If the condition is FALSE, it checks the Next one (Else If condition) and so on.
import java.util.Scanner; public class ElectricityBill1 { private static Scanner sc; public static void main(String[] args) { int Units; double Amount, Sur_Charge, Total_Amount; sc = new Scanner(System.in); System.out.print(" Please Enter the Units that you Consumed : "); Units = sc.nextInt(); if (Units < 50) { Amount = Units * 2.60; Sur_Charge = 25; } else if (Units <= 100) { // For the First Fifty Units Charge = 130 (50 * 2.60) // Next, we are removing those 50 units from total units Amount = 130 + ((Units - 50 ) * 3.25); Sur_Charge = 35; } else if (Units <= 200) { // First Fifty Units charge = 130, and 50 - 100 is 162.50 (50 * 3.25) // Next, we are removing those 100 units from total units Amount = 130 + 162.50 + ((Units - 100 ) * 5.26); Sur_Charge = 45; } else { /* First Fifty Units = 130, 50 - 100 is 162.50, and 100 - 200 is 526 (100 * 5.65) Next, we are removing those 200 units from total units */ Amount = 130 + 162.50 + 526 + ((Units - 200 ) * 7.75); Sur_Charge = 55; } Total_Amount = Amount + Sur_Charge; System.out.println("\n Electricity Bill = " + Total_Amount); } }
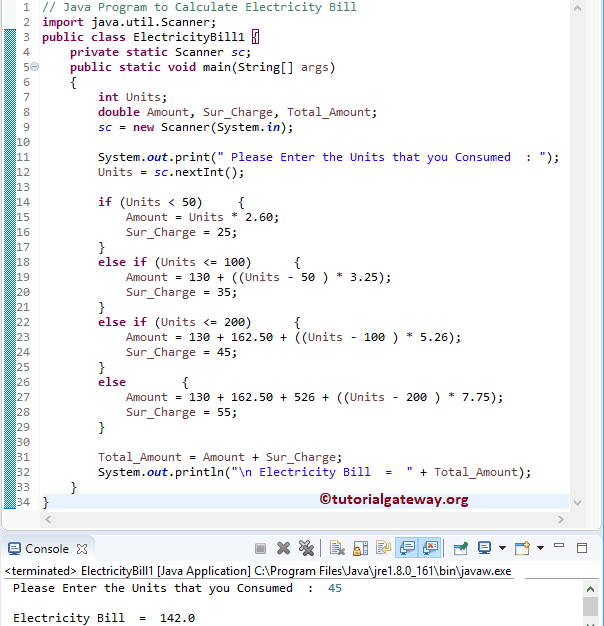
Java Program to find Electricity Bill using Method
This program to calculate electricity bills is the same as the first example. But we separated the logic and placed it in a separate method.
import java.util.Scanner; public class Example2 { private static Scanner sc; public static void main(String[] args) { int Units; double Total_Amount; sc = new Scanner(System.in); System.out.print(" Please Enter the Units that you Consumed : "); Units = sc.nextInt(); Total_Amount = ElecBill(Units); System.out.println("\n Electricity Bill = " + Total_Amount); } public static double ElecBill(int Units) { double Amount, Sur_Charge, Total_Amount; if (Units < 50) { Amount = Units * 2.60; Sur_Charge = 25; } else if (Units <= 100) { Amount = 130 + ((Units - 50 ) * 3.25); Sur_Charge = 35; } else if (Units <= 200) { Amount = 130 + 162.50 + ((Units - 100 ) * 5.26); Sur_Charge = 45; } else { Amount = 130 + 162.50 + 526 + ((Units - 200 ) * 7.75); Sur_Charge = 55; } Total_Amount = Amount + Sur_Charge; return Total_Amount; } }
Please Enter the Units that you Consumed : 95
Electricity Bill = 311.25
Let me try a different value.
Please Enter the Units that you Consumed : 450
Electricity Bill = 2811.0
Java Program to find Electricity Bill Example
This type of Java program is useful if the board has uniform rates. Something like: if you consume between 300 and 500 units, then charges are fixed as 7.75 Rupees for Units, etc.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { int Units; double Amount, Sur_Charge, Total_Amount; sc = new Scanner(System.in); System.out.print(" Please Enter the Units that you Consumed : "); Units = sc.nextInt(); if (Units > 500) { Amount = Units * 9.65; Sur_Charge = 85; } // Otherwise (fails), Compiler will move to Next Else If block else if (Units >= 300) { Amount = Units * 7.75; Sur_Charge = 75; } else if (Units >= 200) { Amount = Units * 5.26; Sur_Charge = 55; } else if (Units >= 100) { Amount = Units * 3.76; Sur_Charge = 35; } // Otherwise (fails), Compiler will move to Else block else { Amount = Units * 2.25; Sur_Charge = 25; } Total_Amount = Amount + Sur_Charge; System.out.println("\n Electricity Bill = " + Total_Amount); } }
Please Enter the Units that you Consumed : 750
Electricity Bill = 7322.5
Let me try a different value.
Please Enter the Units that you Consumed : 250
Electricity Bill = 1370.0
Comments are closed.