How to write a C Program to Swap First and Last Digit Of a Number with an example?.
C Program to Swap First and Last Digit Of a Number Example 1
This program allows the user to enter any number. And then, it is going to swap the First Digit and Last Digit of the user-entered value.
/* C Program to Swap First and Last Digit Of a Number */ #include <stdio.h> #include <math.h> int main() { int Number, FirstDigit, DigitsCount, LastDigit, a, b, SwapNum; printf("\n Please Enter any Number that you wish : "); scanf("%d", & Number); DigitsCount = log10(Number); FirstDigit = Number / pow(10, DigitsCount); LastDigit = Number % 10; a = FirstDigit * (pow(10, DigitsCount)); b = Number % a; Number = b / 10; SwapNum = LastDigit * (pow(10, DigitsCount)) + (Number * 10 + FirstDigit); printf(" \n The Number after Swapping First Digit and Last Digit = %d", SwapNum); return 0; }
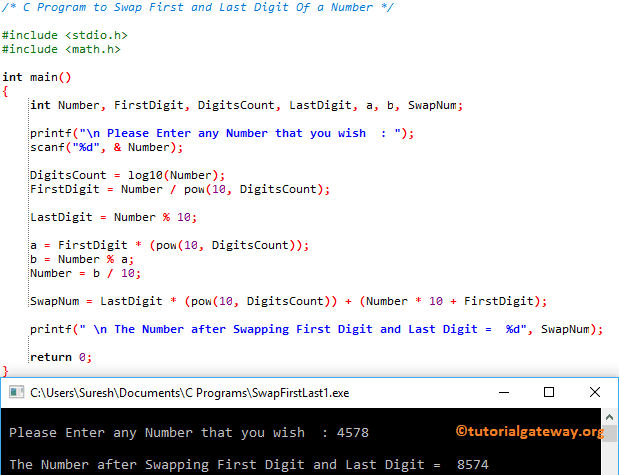
For this C Program to Swap First and Last Digit Of a Number demonstration, User entered Number = 4578
We already explained the Analysis part in our previous articles. So, Please refer First Digit and Last Digit article in C Programming to understand the same.
DigitCount = log10(Number) = 3
FirstDigit = 4578 / pow(10, 3) = 4578 / 1000 = 4.578 = 4
LastDigit = 4578 % 10 = 8
a = FirstDigit * (pow(10, DigitsCount))
a = 4 * pow(10, 3) = 4 * 1000 = 4000
b = Number % a = 4578 % 4000 = 578
Number = 578 / 10 = 57.8 = 57
SwapNum = LastDigit * (pow(10, DigitsCount)) + (Number * 10 + FirstDigit)
SwapNum = 8 * pow(10, 3) + (57 * 10 + 4)
SwapNum = 8000 + (570 + 4) = 8574
C Program to Swap First and Last Digit Of a Number Example 2
This program also allows user to enter any number, and then swap first and last digit of a number
/* C Program to Swap First and Last Digit Of a Number */ #include <stdio.h> #include <math.h> int main() { int Number, FirstDigit, DigitsCount, LastDigit, a, b, SwapNum; printf("\n Please Enter any Number that you wish : "); scanf("%d", & Number); DigitsCount = log10(Number); FirstDigit = Number / pow(10, DigitsCount); LastDigit = Number % 10; SwapNum = LastDigit; SwapNum = SwapNum * (round(pow(10, DigitsCount))); SwapNum = SwapNum + Number % (int)(round(pow(10, DigitsCount))); SwapNum = SwapNum - LastDigit; SwapNum = SwapNum + FirstDigit; printf(" \n The Number after Swapping First Digit and Last Digit = %d", SwapNum); return 0; }
Please Enter any Number that you wish : 95371
The Number after Swapping First Digit and Last Digit = 15379
From the above Program to Swap First and Last Digit Of a Number example, you can see that the user entered value = 95371
DigitsCount = 4
FirstDigit = 9
LastDigit = 1
SwapNum = LastDigit = 1
SwapNum = SwapNum * (round(pow(10, DigitsCount))) = 1 * pow(10, 4) = 10000
SwapNum = SwapNum + Number % (round(pow(10, digitsCount)))
SwapNum = 10000 + (95371 % pow(10, 4))
SwapNum = 10000 + 95371 % 10000 = 10000 + 5371 = 15371
SwapNum = SwapNum – LastDigit = 15371 – 1 = 15370
SwapNum = SwapNum + FirstDigit = 15370 + 9 = 15379