How to write a C Program to Find Last Digit Of a Number with an example?.
C Program to Find Last Digit Of a Number
This program will allow the user to enter any number. And then, it is going to find the Last Digit of the user-entered value.
/* C Program to Find Last Digit Of a Number */ #include <stdio.h> int main() { int Number, LastDigit; printf("\n Please Enter any Number that you wish : "); scanf("%d", & Number); LastDigit = Number % 10; printf(" \n The Last Digit of a Given Number %d = %d", Number, LastDigit); return 0; }
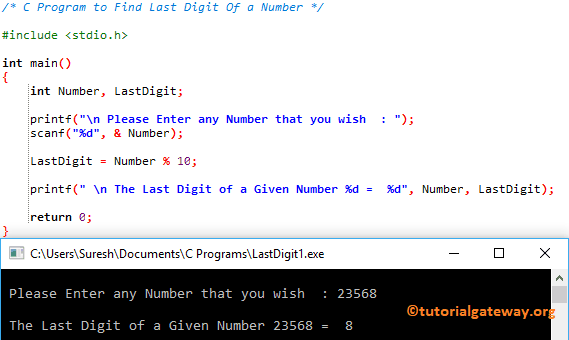
C Program to Find Last Digit Of a Number using Function
This C program for last digit in a number is the same as above. But this time we divided the code using the Functions concept in C Programming.
/* C Program to Find Last Digit Of a Number using Function */ #include <stdio.h> int Last_Digit(int num); int main() { int Number, LastDigit; printf("\n Please Enter any Number that you wish : "); scanf("%d", & Number); LastDigit = Last_Digit(Number); printf(" \n The Last Digit of a Given Number %d = %d", Number, LastDigit); return 0; } int Last_Digit(int num) { return num % 10; }
Please Enter any Number that you wish : 12457325
The Last Digit of a Given Number 12457325 = 5
Comments are closed.