How to write a C Program to Sort Array in Descending Order using For Loop and Functions with an example.
C Program to Sort Array in Descending Order
This program allows the user to enter the Size and the row elements of the One Dimensional Array. Next, in this C program we are using Nested For Loop to sort the array elements in descending order, and print all the elements in this array
#include <stdio.h> int main() { int Array[50], i, j, temp, Size; printf("\nPlease Enter the Number of elements in an array : "); scanf("%d", &Size); printf("\nPlease Enter %d elements of an Array \n", Size); for (i = 0; i < Size; i++) { scanf("%d", &Array[i]); } for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(Array[i] < Array[j]) { temp = Array[i]; Array[i] = Array[j]; Array[j] = temp; } } } printf("\n **** Array of Elemenst in Descending Order are : ****\n"); for (i = 0; i < Size; i++) { printf("%d\t", Array[i]); } return 0; }
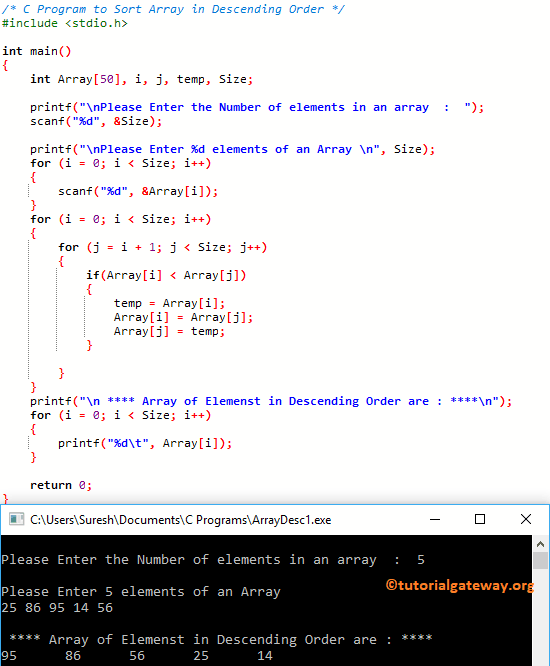
Here, For Loop will make sure that the number is between 0 and the maximum size value of One Dimensional Array. In this example, it will be from 0 to 4
for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(Array[i] < Array[j]) { temp = Array[i]; Array[i] = Array[j]; Array[j] = temp; } } }
In this C Program to Sort Array in Descending Order example,
First For Loop – First Iteration: for(i = 0; 0 < 5; 0++)
The condition is True so, it will enter into the second for loop.
Second For Loop – First Iteration: for(j = 0 + 1; 1 < 5; 1++)
Condition inside the For Loop is True so, the compiler will enter into the If Statement
if(Array[0] < Array[1]) = if(25 < 86) – It means, Condition is True
temp = 25
Array[i] = 86
Array[j] = 25
Now the array will be 86 25 95 14 56. Next, j will increment by 1.
Second For Loop – Second Iteration: for(j = 2; 2 < 5; 2++)
Condition inside the For Loop is True. So, the C Programming compiler will enter into the If Statement: if(Array[0] < Array[2])
if(86 < 95) – It means, the condition is True
temp = 86
Array[i] = 95
Array[j] = 86
Now the array will be 95 25 86 14 56. Next, j will increment by 1.
Second For Loop – Third Iteration: for(j = 3; 3 < 5; 3++)
Condition inside the For Loop is True.
if(Array[0] < Array[3]) => if(95 < 14) – It means, the Condition is False so, it will exit from the If block, and the j value will increment by 1.
Second For Loop – Fourth Iteration: for(j = 4; 4 < 4; 4++)
Condition inside the For Loop is True
if(Array[0] < Array[4]) => if(95 < 56) – It means, the condition is False so, it will exit from the If block, and the j value incremented by 1.
Second For Loop in C Program to Sort Array in Descending Order – Fifth Iteration: for(j = 5; 5 < 5; 5++)
Condition inside the For Loop is False. So, the compiler will exit from the For Loop. Next, i value will increment by 1.
First For Loop – Second Iteration: for(i = 1; 1 < 5; 1++)
The condition is True, so it will enter into the second for loop.
Second For Loop – First Iteration: for(j = 1 + 1; 2 < 5; 2++)
Condition inside the For Loop is True.
if(Array[1] < Array[2]) => if(25 < 86) – It means, Condition is True
temp = 25
Array[i] = 86
Array[j] = 25
Now the array will be 95 86 25 14 56. Next, j will increment by 1.
Second For Loop – Second Iteration: for(j = 3; 3 < 5; 3++)
Condition inside the For Loop is True. So, the compiler will enter into the If Statement: if(Array[1] < Array[3])
if(86 < 14) – It means the Condition is False, so it will exit from the If block and the Second For Loop.
Do the same for the remaining Iterations.
C Program to Sort Array in Descending Order using Functions
This program to sort arrays in descending order is the same as the first example. However, we separated the logic to sort array elements in descending order using Functions.
#include <stdio.h> int *Sort_ArrayDesc(int arr[], int Size); int main() { int Array[50], i, j, Size; int *arr; printf("\nPlease Enter the Number of elements in an array : "); scanf("%d", &Size); printf("\nPlease Enter %d elements of an Array \n", Size); for (i = 0; i < Size; i++) { scanf("%d", &Array[i]); } arr = Sort_ArrayDesc(Array, Size); printf("\n **** Array of Elemenst in Descending Order are : ****\n"); for (i = 0; i < Size; i++) { printf(" Element at Array[%d] = %d \n", i, arr[i]); } return 0; } int *Sort_ArrayDesc(int arr[], int Size) { int i, j, temp; for (i = 0; i < Size; i++) { for (j = i + 1; j < Size; j++) { if(arr[i] < arr[j]) { temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } } return arr; }
Sort Array in Descending Order
Please Enter the Number of elements in an array : 6
Please Enter 6 elements of an Array
25 89 63 48 105 56
**** Array of Elemenst in Descending Order are : ****
Element at Array[0] = 105
Element at Array[1] = 89
Element at Array[2] = 63
Element at Array[3] = 56
Element at Array[4] = 48
Element at Array[5] = 25