How to write a C Program to Reverse Order of Words in a String with an example. For example, the tutorial gateway will become gateway tutorial
C Program to Reverse Order of Words in a String Example 1
This program to reverse words in a string in c allows the user to enter a string (or character array), and a character value. Next, it will reverse the order of words inside a string.
/* C Program to Reverse Order of Words in a String */ #include <stdio.h> #include <string.h> int main() { char str[100]; int i, j, len, startIndex, endIndex; printf("\n Please Enter any String : "); gets(str); len = strlen(str); endIndex = len - 1; printf("\n ***** Given String in Reverse Order ***** \n"); for(i = len - 1; i >= 0; i--) { if(str[i] == ' ' || i == 0) { if(i == 0) { startIndex = 0; } else { startIndex = i + 1; } for(j = startIndex; j <= endIndex; j++) { printf("%c", str[j]); } endIndex = i - 1; printf(" "); } } return 0; }
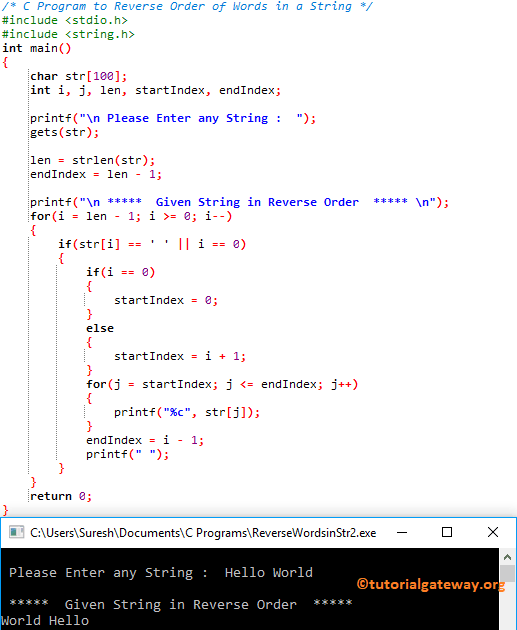
Within this C Program to reverse words in a string Here, we used For Loop to iterate each and every character in a String, and remove all the duplicate characters in it.
for(i = len - 1; i >= 0; i--) { if(str[i] == ' ' || i == 0) { if(i == 0) { startIndex = 0; } else { startIndex = i + 1; } for(j = startIndex; j <= endIndex; j++) { printf("%c", str[j]); } endIndex = i - 1; printf(" "); } }
User entered values in this C program to reverse words in a string
str[] = Hello World
len = 11
endIndex = len – 1 = 10
For Loop – First Iteration: for(i = len – 1; i >= 0; i–)
for(i = 10; 10 >= 0; 10–)
Next, it will enter into the If Statement to check whether the str[i] is equal to empty space or i = 0
This condition will be true once it reaches to empty space after World
for(i = 5; 5 >= 0; 5–)
if(str[5] == ‘ ‘ || 5 == 0) – Condition is True so, it will enter into next If statement
if(i == 0) => if(5 == 0) – Condition is False so, statement inside the else block will be executed
startIndex = i + 1
startIndex = 6
Second For Loop – First Iteration: for(j = startIndex; j <= endIndex; j++)
=> for(j = 6; 6 <= 10; 6++)
Within the For Loop we used C Programming printf statement to print single character
printf(“%c”, str[j]) => W
Do the same for j = 7, j = 8, j= 9, and j = 10. Next, it will start the First For Loop iteration where i = 4.
Do the same for i = 4, i = 3, i = 2, i = 1 and i = 0 in the reverse words in a given string in c program
C Program to Reverse Order of Words in a String Example 2
This reverse words in a string in c program will traverse the string from the end position to start position. Once it finds empty space, it will replace the empty space with a NULL value, and prints the string after that Null value.
/* C Program to Reverse Order of Words in a String */ #include <stdio.h> #include <string.h> int main() { char str[100]; int i, len; printf("\n Please Enter any String : "); gets(str); len = strlen(str); printf("\n ***** Given String in Reverse Order ***** \n"); for(i = len - 1; i >= 0; i--) { if(str[i] == ' ') { str[i] = '\0'; printf("%s ", &(str[i]) + 1); } } printf("%s", str); return 0; }
Please Enter any String : Welcome To Tutorial Gateway
***** Given String in Reverse Order *****
Gateway Tutorial To Welcome
C Program to Reverse Order of Words in a String Example 3
This program to reverse words in a string in c is the same as the first example, but this time we are saving the reverse order words in a separate string.
/* C Program to Reverse Order of Words in a String */ #include <stdio.h> #include <string.h> int main() { char str[100], revstr[100]; int i, j, index, len, startIndex, endIndex; printf("\n Please Enter any String : "); gets(str); len = strlen(str); index = 0; endIndex = len - 1; printf("\n ***** Given String in Reverse Order ***** \n"); for(i = len - 1; i > 0; i--) { if(str[i] == ' ') { startIndex = i + 1; for(j = startIndex; j <= endIndex; j++) { revstr[index] = str[j]; index++; } revstr[index++] = ' '; endIndex = i - 1; } } for(i = 0; i <= endIndex; i++) { revstr[index] = str[i]; index++; } revstr[index] = '\0'; printf("\n Given String in Reverse Order = %s", revstr); return 0; }
Please Enter any String : learn c programming with examples at tutorial gateway
***** Given String in Reverse Order *****
Given String in Reverse Order = gateway tutorial at examples with programming c learn