How to write a C Program to Put Positive and Negative Numbers in two Separate Arrays using For Loop, While Loop, Functions with example.
C Program to Put Positive and Negative Numbers in two Separate Arrays
This program allows the user to enter the Size and the row elements of One Dimensional Array.
/* C Program to Put Positive and Negative Numbers in two Separate Arrays */ #include<stdio.h> void PrintArray(int a[], int Size); int main() { int Size, i, a[10], Positive[10], Negative[10]; int Positive_Count = 0, Negative_Count = 0; printf("\n Please Enter the Size of an Array : "); scanf("%d", &Size); printf("\nPlease Enter the Array Elements : "); for(i = 0; i < Size; i++) { scanf("%d", &a[i]); } for(i = 0; i < Size; i ++) { if(a[i] >= 0) { Positive[Positive_Count] = a[i]; Positive_Count++; } else { Negative[Negative_Count] = a[i]; Negative_Count++; } } printf("\n Total Number of Positive Numbers in this Array = %d ", Positive_Count); printf("\n Array Elements in Positive Array : "); PrintArray(Positive, Positive_Count); printf("\n Total Number of Negative Numbers in this Array = %d ", Negative_Count); printf("\n Array Elements in Negative Array : "); PrintArray(Negative, Negative_Count); return 0; } void PrintArray(int a[], int Size) { int i; for(i = 0; i < Size; i++) { printf("%d \t ", a[i]); } printf("\n"); }
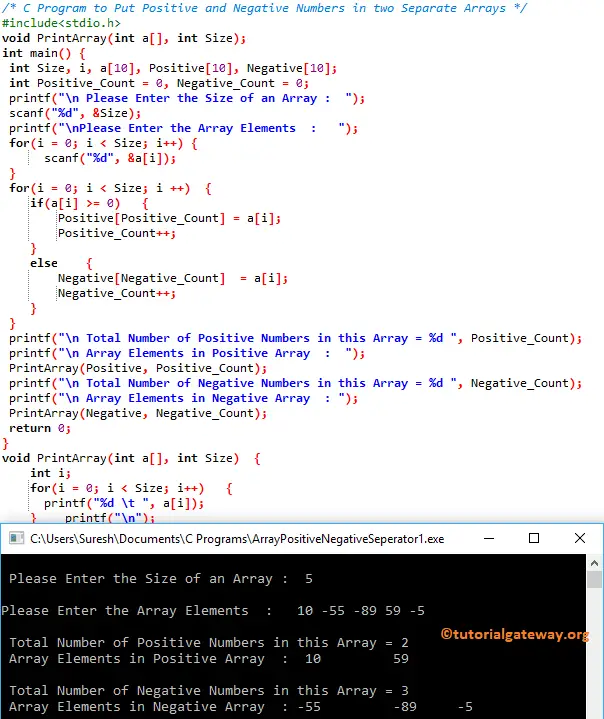
Here, For Loop will make sure that the number is between 0 and maximum size value. In this C Programming example, it will be from 0 to 4
for(i = 0; i < Size; i ++)
In the Next line, We declared the If statement
if(a[i] >= 0) { Positive[Positive_Count] = a[i]; Positive_Count++; }
Any number that is greater than or equal to 0 is positive. If condition will check whether the same.
- If the condition is True, then it is a positive number. So the compiler will assign that element to Positive Array at first position, and next, it will increment the Positive_Count value.
- If the condition is False, then it is a Negative number. So the compiler will assign that element to Negative Array at first position, and next, it will increment Negative_Count value.
void PrintArray(int a[], int Size) function is to print array elements in Positive array, and Negative array
C Program to Put Positive and Negative Numbers in two Separate Arrays using While Loop
This program is the same as above, but this time we used While Loop to Put Positive and Negative Numbers in two Separate Arrays
/* C Program to Put Positive and Negative Numbers in two Separate Arrays */ #include<stdio.h> void PrintArray(int a[], int Size); int main() { int Size, i, j = 0, a[10], Positive[10], Negative[10]; int Positive_Count = 0, Negative_Count = 0; printf("\n Please Enter the Size of an Array : "); scanf("%d", &Size); printf("\n Please Enter the Array Elements : "); for(i = 0; i < Size; i++) { scanf("%d", &a[i]); } while(j < Size) { if(a[j] >= 0) { Positive[Positive_Count] = a[j]; Positive_Count++; } else { Negative[Negative_Count] = a[j]; Negative_Count++; } j++; } printf("\n Total Number of Positive Numbers in this Array = %d ", Positive_Count); printf("\n Array Elements in Positive Array : "); PrintArray(Positive, Positive_Count); printf("\n Total Number of Negative Numbers in this Array = %d ", Negative_Count); printf("\n Array Elements in Negative Array : "); PrintArray(Negative, Negative_Count); return 0; } void PrintArray(int a[], int Size) { int i = 0; while(i < Size) { printf("%d \t ", a[i]); i++; } printf("\n"); }
Please Enter the Size of an Array : 7
Please Enter the Array Elements : 25 -88 -98 48 -45 15 79
Total Number of Positive Numbers in this Array = 4
Array Elements in Positive Array : 25 48 15 79
Total Number of Negative Numbers in this Array = 3
Array Elements in Negative Array : -88 -98 -45
Program to Put Positive and Negative Numbers in two Separate Arrays using Functions
This program is the same as the first example. But we separated the logic to put Positive numbers, and Negative Numbers in two separate arrays using Functions.
/* C Program to Put Positive and Negative Numbers in two Separate Arrays */ #include<stdio.h> void CountPositiveNumbers(int a[], int Size); void CountNegativeNumbers(int a[], int Size); void PrintArray(int a[], int Size); int main() { int Size, i, a[10]; printf("\n Please Enter the Size of an Array : "); scanf("%d", &Size); printf("\nPlease Enter the Array Elements : "); for(i = 0; i < Size; i++) { scanf("%d", &a[i]); } CountPositiveNumbers(a, Size); CountNegativeNumbers(a, Size); return 0; } void CountPositiveNumbers(int a[], int Size) { int i, Positive[10], Positive_Count = 0; printf("\n List of Array Elements in Positive Array: "); for(i = 0; i < Size; i ++) { if(a[i] >= 0) { Positive[Positive_Count] = a[i]; Positive_Count++; } } PrintArray(Positive, Positive_Count); printf(" Total Number of Positive Numbers in this Array = %d ", Positive_Count); } void CountNegativeNumbers(int a[], int Size) { int i, Negative[10], Negative_Count = 0; printf("\n List of Array Elements in Negative Array: "); for(i = 0; i < Size; i ++) { if(a[i] < 0) { Negative[Negative_Count] = a[i]; Negative_Count++; } } PrintArray(Negative, Negative_Count); printf(" Total Number of Negative Numbers in this Array = %d ", Negative_Count); } void PrintArray(int a[], int Size) { int i; for(i = 0; i < Size; i++) { printf("%d \t ", a[i]); } printf("\n"); }
Please Enter the Size of an Array : 10
Please Enter the Array Elements : 15 -89 -78 -89 -55 -25 12 75 105 869
List of Array Elements in Positive Array: 15 12 75 105 869
Total Number of Positive Numbers in this Array = 5
List of Array Elements in Negative Array: -89 -78 -89 -55 -25