Write a C program to print a sandglass star pattern using a for loop, while loop, do while loop, and functions with an example.
#include <stdio.h> int main(void) { int i, j, k, rows; printf("Enter Sandglass Star Pattern Rows = "); scanf("%d",&rows); for (i = 1; i <= rows; i++) { for (j = 1; j < i; j++) { printf(" "); } for (k = i; k <= rows; k++) { printf("* "); } printf("\n"); } for (i = rows - 1; i >= 1; i--) { for (j = 1; j < i; j++) { printf(" "); } for (k = i; k <= rows; k++) { printf("* "); } printf("\n"); } }
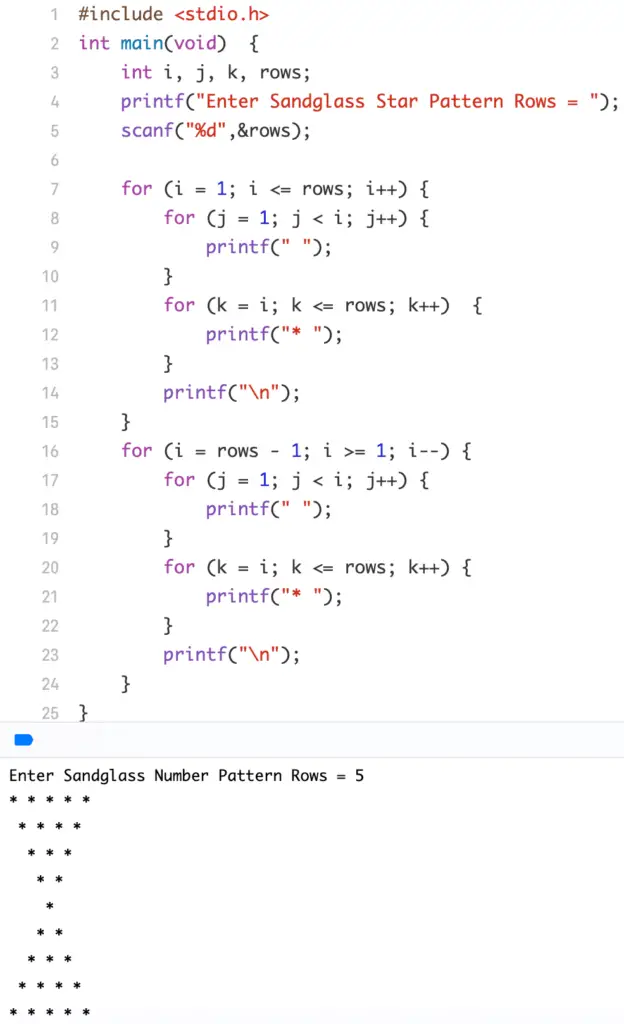
This C example uses a while loop to display the stars in a sandglass pattern or structure. Here, we replaced the for loop with a while loop.
#include <stdio.h> void loopLogic(int rows, int i) { int j = 1; while (j < i ) { printf(" "); j++; } int k = i; while (k <= rows ) { printf("* "); k++; } printf("\n"); } int main(void) { int i, j, k, rows; printf("Enter Sandglass Star Pattern Rows = "); scanf("%d",&rows); i = 1; while (i <= rows) { loopLogic(rows, i); i++; } i = rows - 1; while (i >= 1) { loopLogic(rows, i); i--; } }
Enter Sandglass Star Pattern Rows = 7
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
C program to print sandglass star pattern using do while loop
Please refer to the Do while loop article.
#include <stdio.h> void loopLogic(int rows, int i) { int j = 1; do { printf(" "); j++; } while (j < i ); int k = i; do { printf("* "); k++; } while (k <= rows ); printf("\n"); } int main(void) { int i, j, k, rows; printf("Enter Sandglass Star Pattern Rows = "); scanf("%d",&rows); i = 1; do { loopLogic(rows, i); i++; } while (i <= rows); i = rows - 1; do { loopLogic(rows, i); i--; } while (i >= 1); }
Enter Sandglass Star Pattern Rows = 11
* * * * * * * * * * *
* * * * * * * * * *
* * * * * * * * *
* * * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
* * * * * * * * * *
* * * * * * * * * * *
This example uses the functions and accepts the user-given symbol as an input and prints a given symbol’s sandglass pattern.
#include <stdio.h> void loopLogic(int rows, int i, char ch) { for (int j = 1; j < i; j++ ) { printf(" "); } for (int k = i; k <= rows; k++ ) { printf("%c ", ch); } printf("\n"); } int main(void) { int i, rows; char ch; printf("Enter Character = "); scanf("%c", &ch); printf("Enter Sandglass Star Pattern Rows = "); scanf("%d",&rows); for (i = 1; i <= rows; i++) { loopLogic(rows, i, ch); } for (i = rows - 1; i >= 1; i--) { loopLogic(rows, i, ch); } }
Enter Character = $
Enter Sandglass Star Pattern Rows = 9
$ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $
$ $ $ $ $ $ $
$ $ $ $ $ $
$ $ $ $ $
$ $ $ $
$ $ $
$ $
$
$ $
$ $ $
$ $ $ $
$ $ $ $ $
$ $ $ $ $ $
$ $ $ $ $ $ $
$ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $