How to write a C Program to Print Odd Numbers from 1 to N using For Loop and While Loop?.
C Program to Print Odd Numbers from 1 to N using For Loop
This C program to display Odd Numbers from 1 to N allows the user to enter the maximum limit value. Next, it is going to print the list of all odd numbers from 1 to user-entered value.
/* C Program to Print Odd Numbers from 1 to N using For Loop and If */ #include<stdio.h> int main() { int i, number; printf("\n Please Enter the Maximum Limit Value : "); scanf("%d", &number); printf("\n Odd Numbers between 1 and %d are : \n", number); for(i = 1; i <= number; i++) { if ( i % 2 != 0 ) { printf(" %d\t", i); } } return 0; }
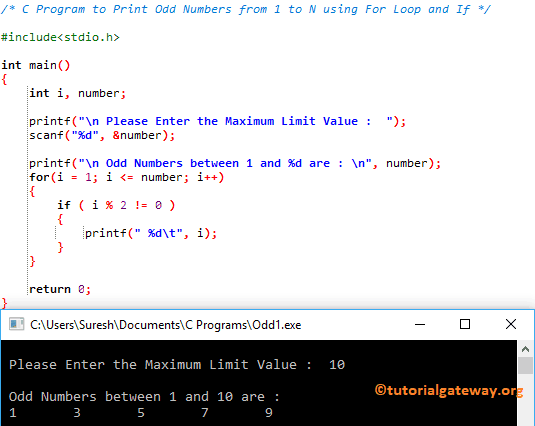
Within this Program to Print Odd Numbers from 1 to N example, For Loop will make sure that the number is between 1 and maximum limit value.
for(i = 1; i <= number; i++)
In the Next line, We declared the If statement
if ( number % 2 != 0 )
Any number that is not divisible by 2 is an Odd number. If condition will check whether the remainder of the number divided by 2 is not equal to 0 or not. If the condition is True, then it is an Odd number, and the compiler will print i value.
Program to Print Odd Numbers from 1 to N without If Statement
This program to Print Odd Numbers from 1 to N in c is the same as the above but, we just altered the for loop to eliminate If statement.
If you observe the below C Programming code snippet, We started i from 1 and incremented it by 2 (not 1). It means, for the first iteration, i will be 1, and for the second iteration, i will be 3 (not 2), etc.
#include<stdio.h> int main() { int i, number; printf("\n Please Enter the Maximum Limit Value : "); scanf("%d", &number); printf("\n Odd Numbers between 1 and %d are : \n", number); for(i = 1; i <= number; i= i+2) { printf(" %d\t", i); } return 0; }
Please Enter the Maximum Limit Value : 18
Odd Numbers between 1 and 18 are :
1 3 5 7 9 11 13 15 17
C Program to Print Odd Numbers from 1 to 100 using While Loop
This Print Odd Numbers from 1 to N is the same as above. We just replaced the For Loop with While Loop.
#include<stdio.h> int main() { int i = 1, number; printf("\n Please Enter the Maximum Limit Value : "); scanf("%d", &number); printf("\n Odd Numbers between 1 and %d are : \n", number); while(i <= number) { printf(" %d\t", i); i = i + 2; } return 0; }
C odd numbers from 1 to 100 output
Please Enter the Maximum Limit Value : 100
Odd Numbers between 1 and 100 are :
1 3 5 7 9 11 13 15 17 19 21 23 25 27 29 31 33 35 37 39 41 43 45 47 49 51 53 55 57 59 61 63 65 67 69 71 73 75 77 79 81 83 85 87 89 91 93 95 97 99
C Program to Print Odd Numbers in a Given Range
This program allows the user to enter Minimum and maximum value. Next, the C program will print a list of all even numbers between Minimum value and maximum value.
#include<stdio.h> int main() { int i, Minimum, Maximum; printf("\n Please Enter the Minimum Limit Value : "); scanf("%d", &Minimum); printf("\n Please Enter the Maximum Limit Values : "); scanf("%d", &Maximum); if ( Minimum % 2 == 0 ) { Minimum++; } printf("\n Odd Numbers between %d and %d are : \n", Minimum, Maximum); for(i = Minimum; i <= Maximum; i= i+2) { printf(" %d\t", i); } return 0; }
Please Enter the Minimum Limit Value : 14
Please Enter the Maximum Limit Values : 125
Odd Numbers between 15 and 125 are :
15 17 19 21 23 25 27 29 31 33 35 37 39 41 43 45 47 49 51 53 55 57 59 61 63 65 67 69 71 73 75 77 79 81 83 85 87 89 91 93 95 97 99 101 103 105 107 109 111 113 115 117 119 121 123 125