Write a C program to print the Inverted Triangle Star Pattern using for loop, while loop, and functions with an example. The below inverted program uses the nested for loops to iterate the rows and columns of a Triangle and prints stars.
#include<stdio.h> int main(void) { int rows; printf("Enter Rows to Print Inverted Triangle = "); scanf("%d", &rows); for (int i = rows ; i > 0; i-- ) { for (int j = 1 ; j <= rows - i; j++ ) { printf(" "); } for (int k = 1 ; k <= i * 2 - 1; k++ ) { printf("* "); } printf("\n"); } }
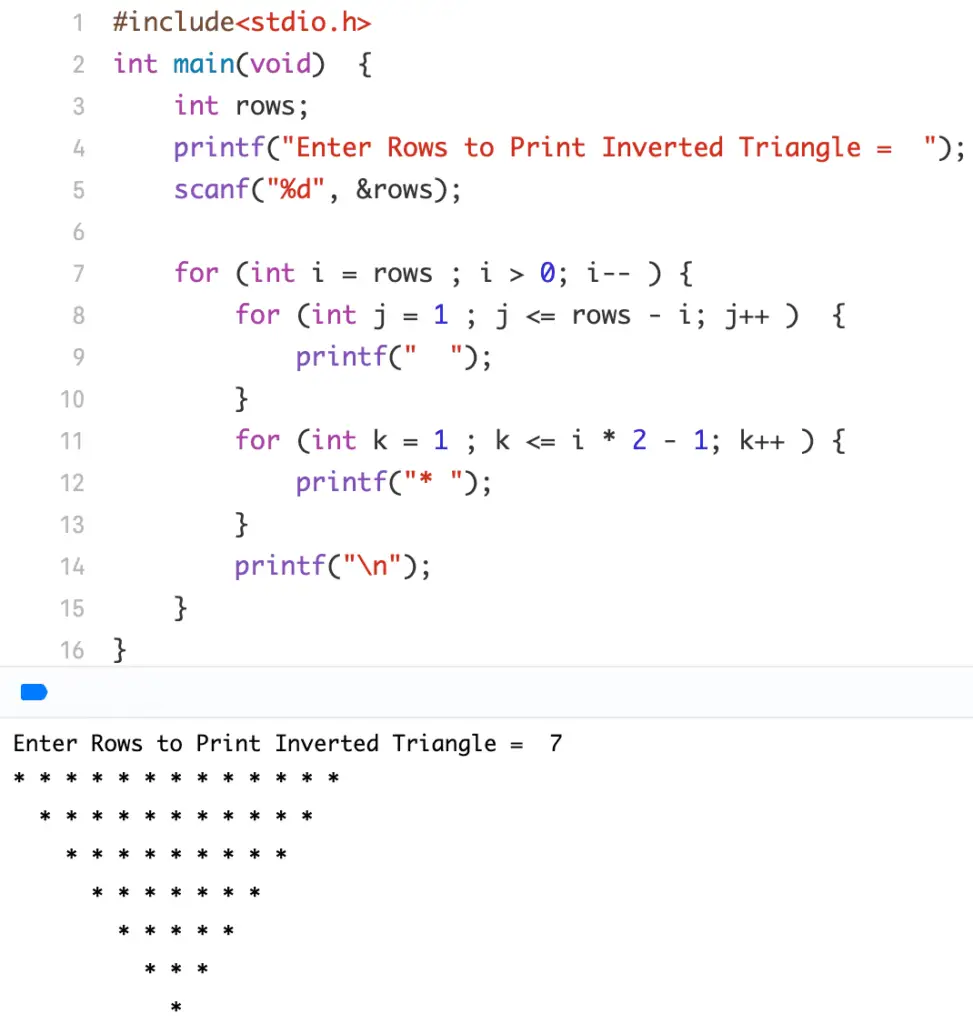
C Program to Print Inverted Triangle Star Pattern using while loop
In this Inverted Triangle Star Pattern program, we replaced the for loop with the while loop.
#include<stdio.h> int main(void) { int rows; printf("Enter Rows to Print Inverted Triangle = "); scanf("%d", &rows); int i = rows ; while (i > 0) { int j = 1 ; while ( j <= rows - i ) { printf(" "); j++; } int k = 1 ; while ( k <= i * 2 - 1 ) { printf("* "); k++; } printf("\n"); i--; } }
Output
Enter Rows to Print Inverted Triangle = 8
* * * * * * * * * * * * * * *
* * * * * * * * * * * * *
* * * * * * * * * * *
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
This example uses the do while loop and prints the Inverted Triangle Stars pattern.
#include<stdio.h> int main(void) { int rows; printf("Enter Rows to Print Inverted Triangle = "); scanf("%d", &rows); int i = rows ; do { int j = 0 ; do { printf(" "); j++; } while ( j <= rows - i ); int k = 1 ; do { printf("* "); k++; } while ( k <= i * 2 - 1 ); printf("\n"); i--; } while (i > 0); }
Output
Enter Rows to Print Inverted Triangle = 12
* * * * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * *
* * * * * * * * * * * * *
* * * * * * * * * * *
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
In the below Inverted Triangle pattern of the Stars program, the loopLogic function accepts the rows and characters to print the pattern using the symbol.
#include<stdio.h> void loopLogic(int rows, char ch) { for (int i = rows ; i > 0; i-- ) { for (int j = 1 ; j <= rows - i; j++ ) { printf(" "); } for (int k = 1 ; k <= i * 2 - 1; k++ ) { printf("%c ", ch); } printf("\n"); } } int main(void) { int rows; char ch; printf("Enter Character = "); scanf("%c", &ch); printf("Enter Rows to Print Inverted Triangle = "); scanf("%d", &rows); loopLogic(rows, ch); }
Output
Enter Character = $
Enter Rows to Print Inverted Triangle = 16
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $
$ $ $ $ $
$ $ $
$