How to write a C Program to Print Hollow Square Star Pattern with example?. And also show you how to print hollow square pattern with different symbols.
C program to Print Hollow Square Star Pattern
This C program allows the user to enter any side of a square (In Square, all sides are equal). This value will decide the number of rows and columns of a hollow square. Here, we are going to print the stars until it reaches the user-specified rows and columns.
/* C Program to Print Hollow Square Star Pattern */ #include<stdio.h> int main() { int i, j, Side; printf("Please Enter Any Side of a Square\n"); scanf("%d", &Side); printf("Printing Hallow Square Star Pattern \n"); for(i = 0; i < Side; i++) { for(j = 0; j < Side; j++) { if(i == 0 || i == Side-1 || j == 0 || j == Side-1) { printf("*"); } else { printf(" "); } } printf("\n"); } return 0; }
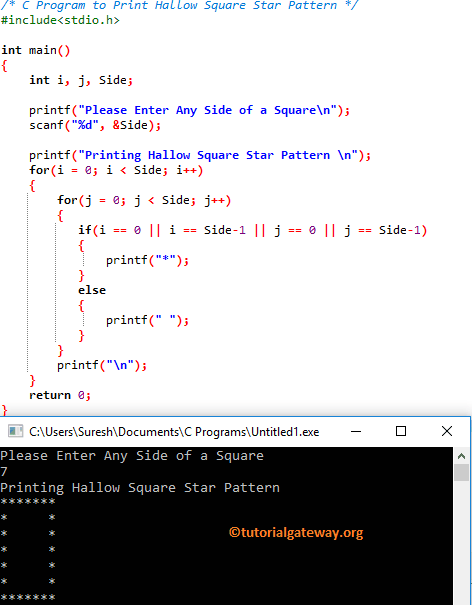
Let us see the Nested for loop
Outer Loop – First Iteration
From the above screenshot, you can observe that the value of i is 0, and Sides is 7. So, the C Programming condition (i < 7) is True. So, it will enter into second for loop.
Inner Loop – First Iteration
The j value is 0 and the condition (j < 7) is True. So, it will start executing the statement inside the loop. So, it will enter into the If Statement. Here, we are checking whether i = 0, j = 0, i = 6 (side – 1) , or j = 6. If any of this condition is true then the following statement will print *
printf("*");
It happens until it reaches 7, and after that, both the Inner Loop and Outer loop will terminate.
C program to display Hollow Square Star Pattern with Dynamic Character
This program allows the user to enter the Symbol that he/she want to print as a hollow square pattern.
#include<stdio.h> int main() { int i, j, Side; char Ch; printf("Please Enter any Symbol\n"); scanf("%c", &Ch); printf("Please Enter Any Side of a Square\n"); scanf("%d", &Side); printf("Printing Hallow Square Star Pattern \n"); for(i = 1; i <= Side; i++) { for(j = 1; j <= Side; j++) { if(i == 1 || i == Side || j == 1 || j == Side) { printf("%c", Ch); } else { printf(" "); } } printf("\n"); } return 0; }
Please Enter any Symbol
#
Please Enter Any Side of a Square
12
Printing Hallow Square Star Pattern
############
# #
# #
# #
# #
# #
# #
# #
# #
# #
# #
############