How to write a C Program to Print Elements in an Array using For Loop, While Loop, and Functions with example.
C Program to Print Elements in an Array
This program to print an array in c allows the user to enter the Size and the row elements of one Dimensional. Next, we are using For Loop to iterate the array values and print all the elements in this array
#include <stdio.h> int main() { int Array[50], i, Number; printf("\nPlease Enter Number of elements in an array : "); scanf("%d", &Number); printf("\nPlease Enter %d elements of an Array \n", Number); for (i = 0; i < Number; i++) { scanf("%d", &Array[i]); } printf("\n Elemenst in this Array are :\n"); for (i = 0; i < Number; i++) { printf("%d\t", Array[i]); } return 0; }
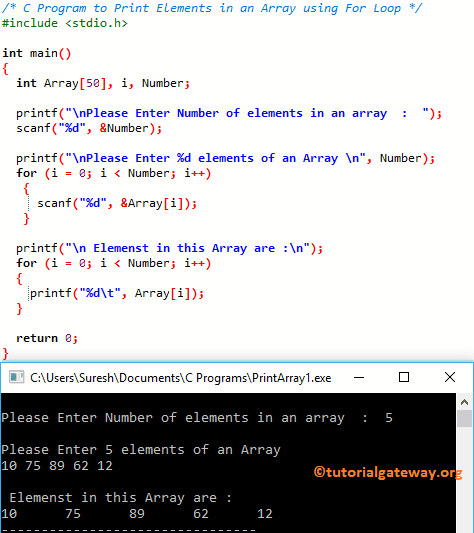
This program prints an array, and the For Loop will make sure that the number is between 0 and the maximum size value. In this example, it will be from 0 to 7
for(i = 0; i < Size; i ++)
First Iteration: for (i = 0; 0 < 5; 0++)
Condition is True, so the C Programming compiler will print the first element(10) in the One Dimensional Array.
Second Iteration: for (i = 1; 1 < 5; 1++)
Condition is True, so the compiler prints the second element (75) in an array
Third Iteration: for (i = 2; 2 < 5; 2++)
Condition is True, so the compiler will print the third element (89) in an array
Fourth Iteration: for (i = 3; 3 < 5; 3++)
Condition is True, so. the compiler will print the fourth element (62) in an array
Fifth Iteration: for (i = 4; 4 < 5; 4++)
Condition is True, so the compiler will print the fifth element (12) in an array
Sixth Iteration: for (i = 5; 5 < 5; 5++)
Condition is False, so the compiler will exit from the for loop
C Program to Print Elements in an Array using While Loop
This program of how to print an array is the same as above. But this time, we used While Loop to print array elements
#include <stdio.h> int main() { int Array[50], i = 0, j = 0, Number; printf("\nPlease Enter Number of elements in an array : "); scanf("%d", &Number); printf("\nPlease Enter %d elements of an Array \n", Number); while (i < Number) { scanf("%d", &Array[i]); i++; } printf("\n **** Elemenst in this Array are : ****\n"); while (j < Number) { printf(" Element at Array[%d] = %d \n", j, Array[j]); j++; } return 0; }
Please Enter Number of elements in an array : 7
Please Enter 7 elements of an Array
25 89 63 105 78 12 8
**** Elemenst in this Array are : ****
Element at Array[0] = 25
Element at Array[1] = 89
Element at Array[2] = 63
Element at Array[3] = 105
Element at Array[4] = 78
Element at Array[5] = 12
Element at Array[6] = 8
C Program to Print Elements in an Array using Functions
This program to print an array is the same as the first example. However, we separated the logic to print array elements using Functions.
#include <stdio.h> void PrintArray(int a[], int Size); int main() { int Array[50], i, Number; printf("\nPlease Enter Number of elements in an array : "); scanf("%d", &Number); printf("\nPlease Enter %d elements of an Array \n", Number); for (i = 0; i < Number; i++) { scanf("%d", &Array[i]); } PrintArray(Array, Number); return 0; } void PrintArray(int a[], int Size) { int i; printf("\n **** Elemenst in this Array are : ****\n"); for (i = 0; i < Size; i++) { printf(" Element at Array[%d] = %d \n", i, a[i]); } }
Please Enter Number of elements in an array : 6
Please Enter 6 elements of an Array
26 36 39 78 14 2
**** Elemenst in this Array are : ****
Element at Array[0] = 26
Element at Array[1] = 36
Element at Array[2] = 39
Element at Array[3] = 78
Element at Array[4] = 14
Element at Array[5] = 2
C Program to Print Elements in an Array using Recursion
This print an array program is the same as the above, but this time we used the Recursion concept.
#include <stdio.h> void PrintArray(int arr[], int Start, int Size); int main() { int Array[50], i, Number; printf("\nPlease Enter Number of elements in an array : "); scanf("%d", &Number); printf("\nPlease Enter %d elements of an Array \n", Number); for (i = 0; i < Number; i++) { scanf("%d", &Array[i]); } printf("\n **** Elemenst in this Array are : ****\n"); PrintArray(Array, 0, Number); return 0; } void PrintArray(int arr[], int Start, int Size) { if(Start >= Size) { return; } printf(" Element at Array[%d] = %d \n", Start, arr[Start]); PrintArray(arr, Start + 1, Size); }
Please Enter Number of elements in an array : 4
Please Enter 4 elements of an Array
14 89 35 82
**** Elemenst in this Array are : ****
Element at Array[0] = 14
Element at Array[1] = 89
Element at Array[2] = 35
Element at Array[3] = 82
Comments are closed.