How to write a C program to find the Total, Average, and Percentage of Five Subjects or N number of subjects
C program to find the Total, Average, and Percentage of Five Subjects
This program helps the user to enter five different values for five subjects. And then, this c program calculates the Total, Average, and Percentage of those Five Subjects.
For this, we are using the C Arithmetic Operators to perform arithmetic operations.
#include <stdio.h> int main() { int english, chemistry, computers, physics, maths; float Total, Average, Percentage; printf("Please Enter the marks of five subjects: \n"); scanf("%d%d%d%d%d", &english, &chemistry, &computers, &physics, &maths); Total = english + chemistry + computers + physics + maths; Average = Total / 5; Percentage = (Total / 500) * 100; printf("Total Marks = %.2f\n", Total); printf("Average Marks = %.2f\n", Average); printf("Marks Percentage = %.2f", Percentage); return 0; }
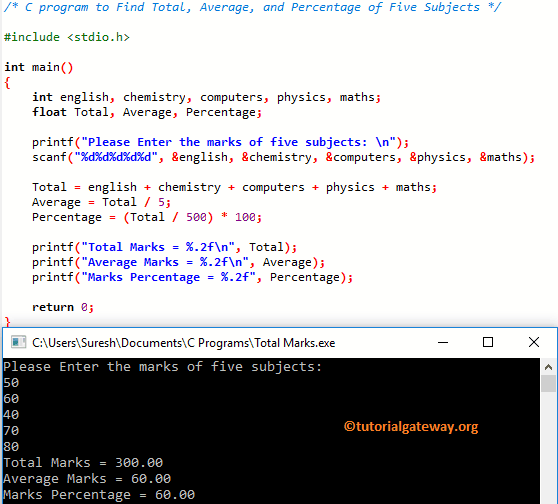
C program to Find the Total, Average, and Percentage of Five Subjects Marks
The above program is more of a fixed subject. In this C program, a user has the flexibility to choose a number of subjects. And then, it will find the Total marks, their Average, and the percentage
#include <stdio.h> int main() { int Total_Subjects, i; float Marks, Total, Average, Percentage; printf("Please enter the Total Number of Subjects\n"); scanf("%d", &Total_Subjects); printf("Please Enter the Marks for each Subject\n"); for(i = 0; i < Total_Subjects; i++) { scanf("%f", &Marks); Total = Total + Marks; } Average = Total / Total_Subjects; Percentage = (Total/(Total_Subjects * 100)) * 100; printf("Total Marks of %d Subjects = %0.2f\n",Total_Subjects,Total); printf("Average Marks = %.2f\n", Average); printf("Percentage = %.2f", Percentage); return 0; }
Please enter the Total Number of Subjects
6
Please Enter the Marks for each Subject
40
50
60
70
80
90
Total Marks of 6 Subjects = 390.00
Average Marks = 65.00
Percentage = 65.00
Within this C program to calculate the Total, Average, and Percentage of Five Subjects example
- The first C Programming printf statement will ask the user to enter the Total number of Subjects. For example, if the user enters three, the second printf statement will ask the user to enter those three values, one after the other.
- For loop will resist the user not entering more than 3 values by using the condition i<n. In the next line, we are adding the user entered number to Total.
- Outside the loop, we calculated the average and percentage.