How to write a C program to find a Positive or Negative Number or zero using the If Else Statement, Else If, and Conditional Operator with an example?
C program to check whether the number is Positive or Negative: If the number is greater than 0, then the given number is positive, and if it is less than 0, then it is negative. If the number is equal to 0, then the given number is 0.
C program to find Positive or Negative Number using Nested If Condition
This program lets the user enter any number. And then, this C program checks whether the entered number is either positive or negative or zero using Nested If
In this C Positive or Negative Number example, First, if condition checks whether the given number is greater than or equal to 0.
- If the condition fails, then the given number will be negative.
- And, If the condition is true, then we have to check whether the number is greater than 0 or not. If the number is greater than 0, then the given number is positive. Else the value we entered is 0.
#include <stdio.h> int main() { int n; printf("Enter any number:\n"); scanf("%d",&n); if (n >= 0) { if (n > 0) printf("%d is Positive", n); else printf("You have entered Value zero."); } else printf("%d is Negative", n); return 0; }
Positive or Negative output
Enter any number:
223
223 is Positive
Enter any number:
-3
-3 is Negative
Enter any number:
0
You have entered Value zero.
C program to find Positive or Negative Number using Else If
This C program lets the user enter any number and then checks whether the entered value is either positive or negative or zero using the Else If Statement.
This program is structured as if the first condition fails, it will traverse to the second condition. If both the conditions fail, the else statement will execute. Let’s see the working principle of both conditions below.
- First Condition(if (number > 0)): Checks whether the given number is greater than 0. If this condition is true, the given value is a positive number.
- The second condition(else if (number < 0)): Checks whether the given number is less than 0. If this condition is true, it is a negative number
- If both the above conditions fail, the number is equal to 0.
#include <stdio.h> int main() { int number; printf(" Enter any number:\n "); scanf("%d",&number); if (number > 0) printf("%d is positive number", number); else if (number < 0) printf("%d is a negative number.", number); else printf(" You entered value zero."); return 0; }
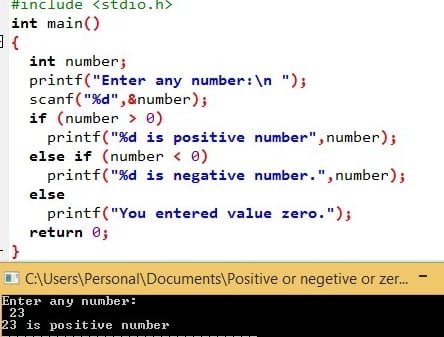
Check for Negative Value
Enter any number:
-11
-11 is a negative number.
Check for Zero
Enter any number:
0
You entered value zero.
C program to find Positive or Negative Number using Conditional Operator
This program lets the user enter any number. Next, this C program checks whether the given value is positive or negative or zero using the Conditional Operator or Ternary operator
To check positive or negative numbers within this program, Let us look into the conditional operator in the code below. Here we used a nested conditional operator rather than the normal one.
If the condition (num > 0) is true, the first statement will execute. And, if it fails, it will check the second condition, which is after the : symbol (num < 0).
- If this condition is true, it will print the first condition printf(“\n It is Negative”)
- and, If this condition is false, printf(“\n It is zero”); will print.
#include <stdio.h> int main() { int num; printf(" Enter any num \n"); scanf("%d",&num); (num > 0)? printf(" It is Positive \n"): (num < 0)? printf(" It is Negative \n"):printf(" It is zero \n"); return 0; }
Enter any num
12
It is Positive
Enter any num
-19
It is Negative
Enter any num
0
It is zero