In C Programming, there are many approaches to find the largest number of the three numbers. Let me show you how to write a C program to find the largest of three numbers using the Else If Statement, Nested If, and Conditional Operator.
C Program to find Largest of Three numbers using Else If Statement
This program helps the user to enter three different values. Then this C program finds the largest number among three numbers using the Else If Statement.
#include <stdio.h> int main() { int a, b, c; printf("Please Enter three different values\n"); scanf("%d %d %d", &a, &b, &c); if (a > b && a > c) { printf("\n%d is Highest Among both %d and %d", a, b, c); } else if (b > a && b > c) { printf("\n%d is Highest Among both %d and %d", b, a, c); } else if (c > a && c > b) { printf("\n%d is Highest Among both %d and %d", c, a, b); } else { printf("\nEither any two values or all the three values are equal"); } return 0; }
Analysis of the Else If Statement in C Program to Find the Largest of Three Numbers.
- In this example, the first if condition checks whether a is greater than b and a is greater than c. If this condition is True, a is highest among both b and c.
- The first Else if condition checks whether b is greater than a and b is greater than c. If this condition is True, b is highest among both a and c.
- The second Else if condition checks whether c is greater than a and c is greater than b. If this condition is True, c is highest among both a and b.
- If all the above conditions fail, it means they are equal.
Output 1
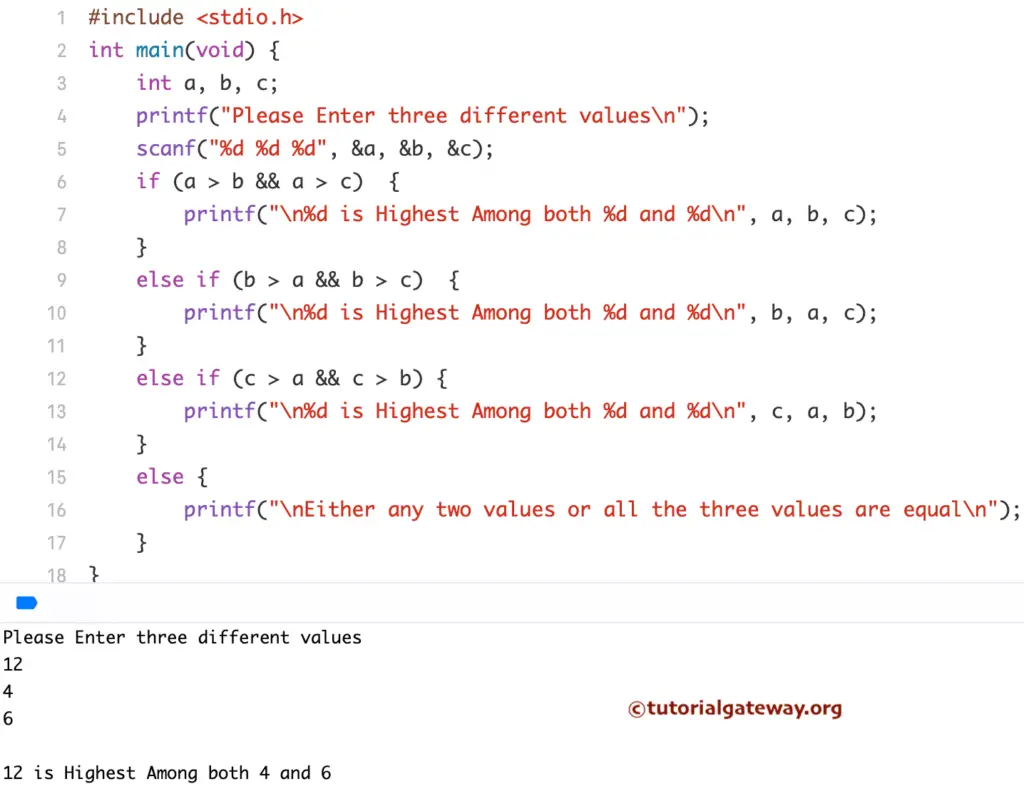
OUTPUT 2:
Please Enter three different values
19
25
20
25 is Highest Among both 19 and 20
3rd OUTPUT:
Please Enter three different values
45
36
96
96 is Highest Among both 45 and 36
Let’s enter 5 as all three numbers
Please Enter three different values
5
5
5
Either any two values or all the three values are equal
C Program to find Largest of Three numbers using Nested If Statement
This program helps the user to enter three different values. Next, this C program finds the Largest of Three numbers using Nested If.
#include<stdio.h> int main() { int a, b, c; printf("Please Enter three different values\n"); scanf("%d %d %d", &a, &b, &c); if(a-b>0 && a-c>0) { printf("\n%d is Greater Than both %d and %d", a, b, c); } else { if(b-c>0) { printf("\n%d is Greater Than both %d and %d", b, a, c); } else { printf("\n%d is Greater than both %d and %d", c, a, b); } } return 0; }
In this C program to find the largest of three numbers example.
- First, if condition checks whether a-b and a-c are greater than 0. If we subtract a small number from a big one, this condition fails. Otherwise, it will be True. If this condition is True, then a is greater than both b and c.
- Else statement will execute when the first If condition is False, so there is no need to check for a value. In the C Programming Else statement, we are inserting one more if condition (Nested IF) to check whether b-c is greater than 0. If this condition is True, then b is greater than both a and c.
- Else c is highest among both a, b.
OUTPUT 1: Lets enter the values a = 32, b = 45, c= 98
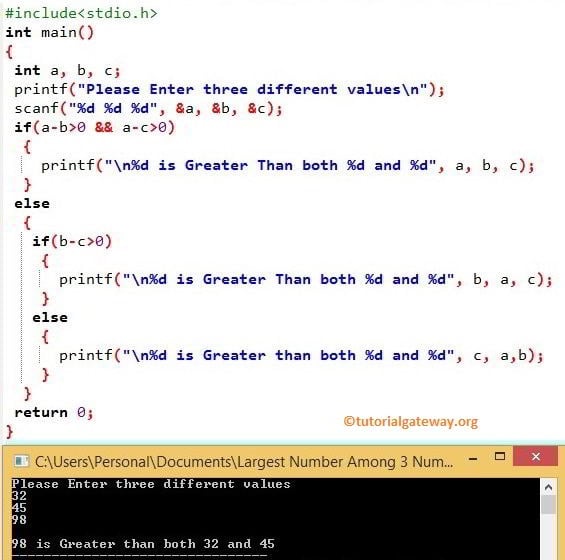
Lets enter the values a = 22, b = 5, c= 7
Please Enter three different values
22
5
7
22 is Greater Than both 5 and 7
OUTPUT 3:
Please Enter three different values
56
222
98
222 is Greater Than both 56 and 98
C Program to find Largest of Three numbers using Conditional Operator
This program helps the user to enter three different values. Next, this C Program finds the largest among three numbers using a Conditional Operator.
#include<stdio.h> int main() { int a, b, c, largest; printf("Please Enter three different values\n"); scanf("%d %d %d", &a, &b, &c); largest =((a>b && a>c)?a: (b>c)?b:c); printf("\nLargest : %d", largest); return 0; }
Within this conditional operator example
- We declared an integer variable called the largest, and we assigned this variable to the conditional operator functionality.
- The first condition checks whether a is greater than b and a is greater than c. If this condition is True, it returns the first value after the ? symbol, which is variable a (a is greater than both b, c).
- If the first condition fails, it executes the variable after the : symbol. We are checking one more condition (b>c) using the Nested conditional operator. If this condition is True, it returns the first value after the ? symbol, which is variable b (b is greater than both a and c.
- If the Nested condition fails, return the variable after the : symbol, which is variable c. It means c is greater than both a and b.
OUTPUT 1: Let’s enter the values a= 56, b= 9, c= 26.
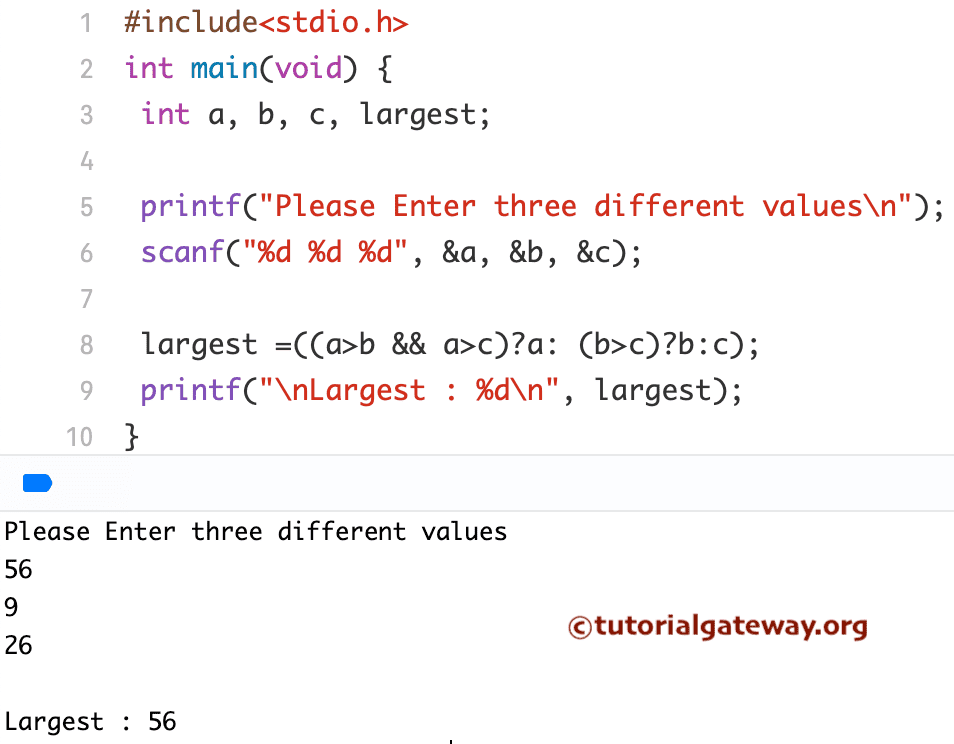
output 2:
Please Enter three different values
12
48
39
Largest : 48
a= 2, b= 56, c=98
Please Enter three different values
2
56
98
Largest : 98
Comments are closed.