Write a C Program to Find the First Occurrence of a Word in a String using For Loop and While Loop with example.
C Program to Find First Occurrence of a Word in a String Example 1
This program allowed the user to enter any string or character array and stored it in the str variable. Next, it will ask the user to enter any word to search. Next, the compiler will search the entire string, and find the first occurrence of a word.
/* C Program to Find First Occurrence of a Word in a String */ #include <stdio.h> int main() { char str[100], word[100]; int i, j, Flag; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Word that you want to Search for : "); gets(word); for(i = 0; str[i] != '\0'; i++) { if(str[i] == word[0]) { Flag = 1; for(j = 0; word[j] != '\0'; j++) { if(str[i + j] != word[j]) { Flag = 0; break; } } } if(Flag == 1) { break; } } if(Flag == 0) { printf("\n Sorry!! We haven't found the Word '%s' ", word); } else { printf("\n We found '%s' at position %d ", word, i + 1); } return 0; }
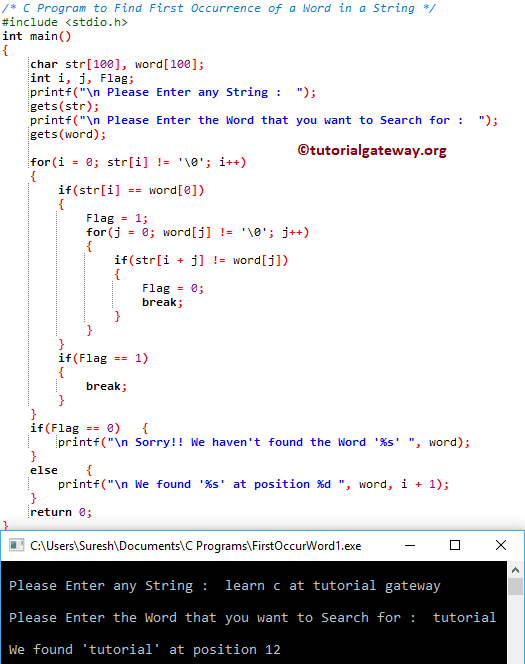
str = learn c at tutorial gateway
word = tutorial
First For Loop – First Iteration: for(i = 0; str[i] != ‘\0’; i++)
The condition is True because str[0] = l
if(str[i] == word[0]) => if(l == t ) – Condition is False
Please do the same until i reaches 11 because at index position 11 str[i] is equal to the word[0]
First For Loop – 12th Iteration: for(i = 11; str[11] != ‘\0’; i++)
The condition is True because str[11] = t
if(str[i] == word[0]) => if(t == t ) – Condition is True
Flag = 1
Next, the C Programming compiler will enter into second For loop. Here, Second For loop is to check whether every character in a word is equal to a word in str or not.
Second For Loop – First Iteration: for(j = 0; word[j] != ‘\0’; j++)
The condition is True because word[0] = t
if(str[i + j] != word[j]) => if(str[11 + 0] != word[0])
=>if(t != t) – Condition is False
Do the same for the remaining iterations until you complete the comparison of str[i + j] vs word[j]. As you know, the condition if(str[i + j] != word[j]) will always return false. If any single character is not matching then if(str[i + j] != word[j]) will become true, and Flag will become 0.
Once the j value becomes 8, the condition inside the second for loop will fail. Next, compiler will enter into the If statement
if(Flag == 0) – Condition is False. So, the compiler will print the statement inside the Else block. Remember, i value is the index position, and i + 1 means actual position.
C Program to Find First Occurrence of a Word in a String Example 2
In this first occurrence word C program, we just replaced the For Loop with the While Loop. I suggest you refer While Loop article to understand the logic.
/* C Program to Find First Occurrence of a Word in a String */ #include <stdio.h> int main() { char str[100], word[100]; int i, j, Flag; printf("\n Please Enter any String : "); gets(str); printf("\n Please Enter the Word that you want to Search for : "); gets(word); i = 0; while(str[i] != '\0') { if(str[i] == word[0]) { Flag = 1; j = 0; while(word[j] != '\0') { if(str[i + j] != word[j]) { Flag = 0; break; } j++; } } if(Flag == 1) { break; } i++; } if(Flag == 0) { printf("\n Sorry!! We haven't found the Word '%s' ", word); } else { printf("\n We found '%s' at position %d ", word, i + 1); } return 0; }
Please Enter any String : c programming tutorial at tutorial gateway website
Please Enter the Word that you want to Search for : tutorial
We found 'tutorial' at position 15