How to write a C Program to Delete Duplicate Elements from an Array?. Before going into this article.
C Program to Delete Duplicate Elements from an Array Example 1
This program to remove duplicates from array in c allows the user to enter Array Size and array elements. Next, it is going to find the duplicate elements present in this array, and delete them using For Loop.
Please refer to Array in C article to understand the concept of Array size, index position, etc.
/* C Program to Delete Duplicate Elements from an Array */ #include <stdio.h> int main() { int arr[10], i, j, k, Size; printf("\n Please Enter Number of elements in an array : "); scanf("%d", &Size); printf("\n Please Enter %d elements of an Array \n", Size); for (i = 0; i < Size; i++) { scanf("%d", &arr[i]); } for (i = 0; i < Size; i++) { for(j = i + 1; j < Size; j++) { if(arr[i] == arr[j]) { for(k = j; k < Size; k++) { arr[k] = arr[k + 1]; } Size--; j--; } } } printf("\n Final Array after Deleteing Duplicate Array Elements is:\n"); for (i = 0; i < Size; i++) { printf("%d\t", arr[i]); } return 0; }
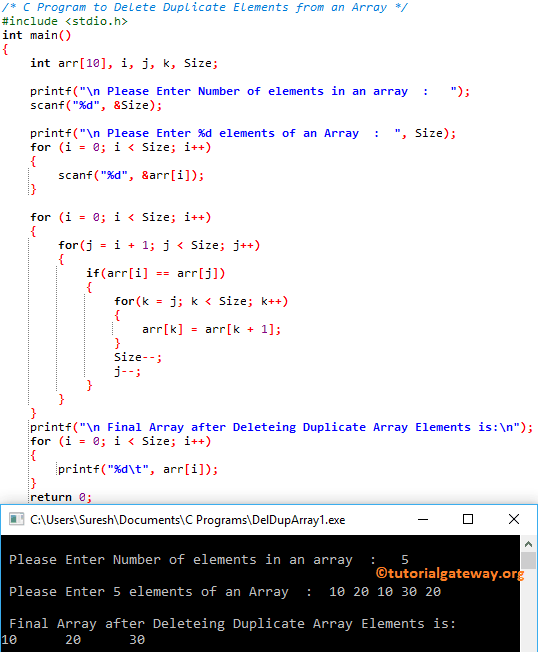
In this remove duplicates from array in c Program, We declared 1 One Dimensional Arrays arr[] of size 10 and also declared i to iterate the Array elements
Below C Programming printf statement asks the User to enter the array arr[] size (Number of elements an Array can hold). And, scanf statement will assign the user entered values to Size variable.
printf("\n Please Enter Number of elements in an array : "); scanf("%d", &Size);
Below For loop will help to iterate every cell present in the arr[5] array. Condition inside the for loop ensures the compiler, not to exceed the array limit.
scanf statement inside the for loop will store the user entered values in every individual array element such as arr[0], arr[1], arr[2], arr[3], arr[4]
for (i = 0; i < Size; i++) { scanf("%d", &arr[i]); }
In the next line, We have one more for loop used to iterate each element in an array. If Statement to check for the duplicates
for (i = 0; i < Size; i++) { for(j = i + 1; j < Size; j++) { if(arr[i] == arr[j]) { for(k = j; k < Size; k++) { arr[k] = arr[k + 1]; } Size--; j--; } } }
From the above screenshot, you can observe that the user inserted values are
a[5] = {10, 20, 10, 30, 20}
First For Loop – First Iteration: for(i = 0; i < 5; 0++)
The condition (0 < 5) is True.
Second For Loop – First Iteration: for(j = 0 + 1; 1 < 5; 1++)
The condition (1 < 5) is True. So, it will start executing the statements inside the loop
if(arr[i] == arr[j])
if(10 == 20) – Condition is False
Second For Loop – Second Iteration: for(j = 2; 2 < 5; 2++)
The condition (2 < 5) is True. So, it will start executing the statements inside the loop
if(arr[i] == arr[j])
if(10 == 10) – Condition is True
Third For Loop – First Iteration: for(k = j; k < Size; k++)
for(k = j; k < Size; k++) => for(k = 2; 2 < 5; 2++)
arr[k] = arr[k + 1]
arr[2] = arr[3] = 30
Size and j Value will be decremented. It means, Size = 4, j = 1, and arr[4] = {10 20 30 20}
Do the same for remaining iterations.
C Program to Delete Duplicate Elements from an Array Example 2
This program is the same as above, but this time we are using If Else statement
/* C Program to Delete Duplicate Elements from an Array */ #include <stdio.h> int main() { int arr[10], i, j, k, Size; printf("\n Please Enter Number of elements in an array : "); scanf("%d", &Size); printf("\n Please Enter %d elements of an Array \n", Size); for (i = 0; i < Size; i++) { scanf("%d", &arr[i]); } for (i = 0; i < Size; i++) { for(j = i + 1; j < Size;) { if(arr[i] == arr[j]) { for(k = j; k < Size; k++) { arr[k] = arr[k + 1]; } Size--; } else { j++; } } } printf("\n Final Array after Deleteing Duplicate Array Elements is:\n"); for (i = 0; i < Size; i++) { printf("%d\t", arr[i]); } return 0; }
Please Enter Number of elements in an array : 7
Please Enter 7 elements of an Array
10 20 30 40 20 10 90
Final Array after Deleteing Duplicate Array Elements is:
10 20 30 40 90
C Program to Delete Duplicate Elements from an Array Example 3
In this program to remove duplicates from array in c, we used multiple If Statements inside the Nested For Loop
/* C Program to Delete Duplicate Elements from an Array */ #include <stdio.h> int main() { int arr[10], b[10], Count = 0, i, j, k, Size; printf("\n Please Enter Number of elements in an array : "); scanf("%d", &Size); printf("\n Please Enter %d elements of an Array : ", Size); for (i = 0; i < Size; i++) { scanf("%d", &arr[i]); } for (i = 0; i < Size; i++) { for(j = 0; j < Count; j++) { if(arr[i] == b[j]) { break; } } if(j == Count) { b[Count] = arr[i]; Count++; } } printf("\n Final Array after Deleteing Duplicate Array Elements is:\n"); for (i = 0; i < Count; i++) { printf("%d\t", b[i]); } return 0; }
Please Enter Number of elements in an array : 10
Please Enter 10 elements of an Array : 10 20 30 10 20 50 60 70 80 150
Final Array after Deleteing Duplicate Array Elements is:
10 20 30 50 60 70 80 150