Write a C Program to check whether the Character is Alphabet or Digit by using the Built-in function isalpha, isdigit, and also not using any built-in function.
C Program to check whether the Character is Alphabet or Digit
This program allows the user to enter one character. Then it will check whether the user-specified character is an Alphabet or a digit.
#include <stdio.h> int main() { char ch; printf(" Please Enter any character : "); scanf("%c", &ch); if( (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z') ) { printf("\n %c is an Alphabet", ch); } else if (ch >= '0' && ch <= '9') { printf("\n %c is a Digit", ch); } else printf("\n %c is not an Alphabet, or a Digit", ch); return 0; }
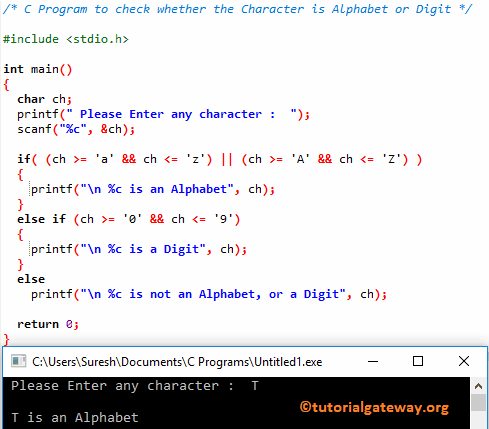
Let us check whether 7 is an Alphabet or Digit
Please Enter any character : 7
7 is a Digit
Let us check whether $ is an Alphabet or not (which is not TRUE)
Please Enter any character : $
$ is not an Alphabet, or a Digit
C program to Check whether the Character is Alphabet or Digit using ASCII Values
In this program, we are using the ASCII values to check whether the input character is Alphabet or Digit. I suggest you refer to the ASCII Table to understand the values of each character in C Programming.
#include <stdio.h> int main() { char ch; printf(" Please Enter any character : "); scanf("%c", &ch); if (ch >= 48 && ch <= 57) { printf("\n %c is a Digit", ch); } else if ( (ch >= 65 && ch <= 90) || (ch >= 97 && ch <= 122) ) { printf("\n %c is an Alphabet", ch); } else printf("\n %c is not an Alphabet, or a Digit", ch); return 0; }
Please Enter any character : 7
7 is a Digit
Let us check whether # is an Alphabet or not (Not TRUE)
Please Enter any character : #
# is not an Alphabet, or a Digit
Let us check whether g is an Alphabet or not (TRUE)
Please Enter any character : g
g is an Alphabet
In this program, we are using the built-in functions isalpha and isdigit to check whether the input character is Alphabet or Digit
#include <stdio.h> #include<ctype.h> int main() { char ch; printf(" Please Enter any character : "); scanf("%c", &ch); if (isdigit(ch)) { printf("\n %c is a Digit", ch); } else if ( isalpha(ch) ) { printf("\n %c is an Alphabet", ch); } else printf("\n %c is not an Alphabet, or a Digit", ch); return 0; }
Please Enter any character : @
@ is not an Alphabet, or a Digit