How to write a C Program to Check Two Matrices are Equal or Not. Or How to write a C program to check whether two Multi-Dimensional Arrays are equal or not with example.
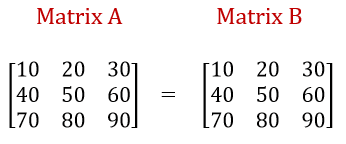
C Program to Check Two Matrices are Equal or Not
This program allows the user to enter the number of rows and columns of two Matrices. Next, C Program will check whether those two matrices are equal or not.
/* C Program to Check Two Matrices are Equal or Not */ #include<stdio.h> int main() { int i, j, rows, columns, a[10][10], b[10][10], isEqual; printf("\n Please Enter Number of rows and columns : "); scanf("%d %d", &i, &j); printf("\n Please Enter the First Matrix Elements\n"); for(rows = 0; rows < i; rows++) { for(columns = 0;columns < j;columns++) { scanf("%d", &a[rows][columns]); } } printf("\n Please Enter the Second Matrix Elements\n"); for(rows = 0; rows < i; rows++) { for(columns = 0;columns < j;columns++) { scanf("%d", &b[rows][columns]); } } isEqual = 1; for(rows = 0; rows < i; rows++) { for(columns = 0;columns < j;columns++) { if(a[rows][columns] != b[rows][columns]) { isEqual = 0; break; } } } if(isEqual == 1) { printf("\n Matrix a is Equal to Matrix b"); } else { printf("\n Matrix a is Not Equal to Matrix b"); } return 0; }
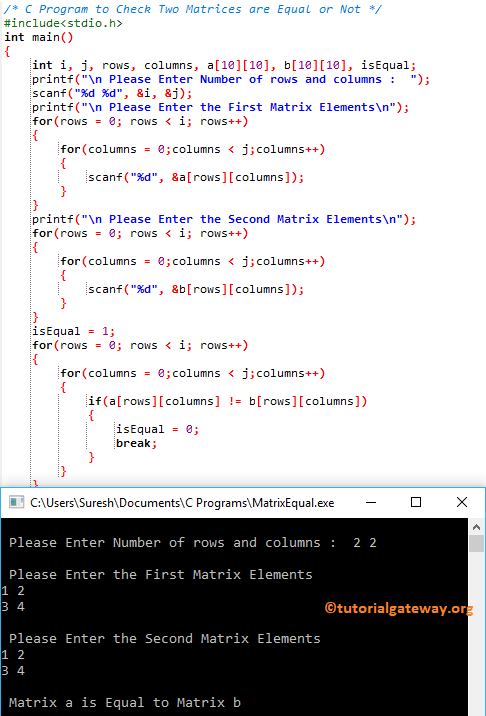
In this C program, We declared Two dimensional arrays a, b of size 10 * 10. Below statements asks the User to enter the Matrices a, b sizes (Number of rows and columns. For instance 2 Rows, 2 Columns = a[2][2] and b[2][2])
printf("\n Please Enter Number of rows and columns : "); scanf("%d %d", &i, &j);
Next, we used for loop to iterate each cell present in a[2][2] matrix. Conditions inside the for loops ((rows < i) and (columns < j)) will ensure the C Programming compiler, not to exceed the Matrix limit. Otherwise, the matrix will overflow
scanf statement inside the for loop will store the user entered values in every individual array element such as a[0][0], a[0][1], a[1][0], a[1][1]
for(rows = 0; rows < i; rows++). { for(columns = 0; columns < j; columns++) { scanf("%d", &a[rows][columns]); } }
Next, for Loop is to store user-entered values into b[2][2] matrix.
In the next line, We have one more for loop to check the array elements are equal or not.
for(rows = 0; rows < i; rows++) { for(columns = 0;columns < j; columns++) { if(a[rows][columns] != b[rows][columns]) { isEqual = 0; break; } } }
The user inserted values for this C Program to Check Two Matrices are Equal or Not are
a[2][2] = {{1, 2}, { 3, 4}}
b[2][2] = {{1, 2}, { 3, 4}}
IsEqual = 1
Row First Iteration: for(rows = 0; rows < 2; 0++)
The condition (0 < 2) is True. So, it will enter into second for loop
Column First Iteration: for(columns = 0; 0 < 2; 0++)
The condition (columns < 2) is True. So, it will enter into the If Statement.
if(a[rows][columns] != b[rows][columns]) => if(a[0][0] != b[0][0] ).
It means, if(1 != 1) – The condition is False. So, it will exit from If statement without executing statements inside the If block.
Column Second Iteration: for(columns = 1; 1 < 2; 1++)
The condition (1 < 2) is True.
if(a[0][1] != b[0][1] ) => if(2 != 2) – The condition is False so, it will exit from If statement
Next, column value will increment. After the increment, the condition inside the second for loop (columns < 2) will fail. So it will exit from the loop.
Now the value of rows will increment and become 1
Row Second Iteration: for(rows = 1; 1 < 2; 1++) // Still IsEqual = 1
The condition (1 < 2) is True. So, it will enter into second for loop
Column First Iteration: for(columns = 0; 0 < 2; 0++)
The condition (columns < 2) is True. So, it will enter into the If Statement.
if(a[1][0] != b[1][0] ) => if(3 != 3) – The condition is False so, it will exit from If statement
Column Second Iteration: for(columns = 1; 1 < 2; 1++)
The condition (1 < 2) is True.
if(a[1][1] != b[1][1] ) => if(4 != 4) – condition is False. So, it will exit from If statement
column value will be incremented. After the increment, the condition inside the second for loop (columns < 2) will fail. So it will exit from the loop.
Next, rows value will become 3. So, the condition inside the for loop (3 < 2) fails. So it will exit from the loop.
At last, we used if(isEqual == 1). If the condition is True, then Matrix A is Equal to B. Otherwise, they are not equal.
Let me try with Wrong values
Please Enter Number of rows and columns : 2 2
Please Enter the First Matrix Elements
1 2
3 4
Please Enter the Second Matrix Elements
1 3
3 4
Matrix a is Not Equal to Matrix b