How to write a C Program to Calculate Profit or Loss. If the actual amount is less than the Sales, then the product is in profits. Else, if the actual is greater than the Sale amount product is in Loss otherwise, none.
C Program to Calculate Profit or Loss Example
This program helps the user to enter the actual and Sales Amount. Using those two values, this Program will check whether the product is in profit or Loss using Else If.
#include<stdio.h> int main() { float Unit_Price, Sales_Amount, Amount; printf("\n Please Enter the Actrual Product Cost : "); scanf("%f", &Unit_Price); printf("\n Please Enter the Sale Price (or Selling Price) : "); scanf("%f", &Sales_Amount); if (Sales_Amount > Unit_Price) { Amount = Sales_Amount - Unit_Price; printf("\n Profit Amount = %.4f", Amount); } else if(Unit_Price > Sales_Amount) { Amount = Unit_Price - Sales_Amount; printf("\n Loss Amount = %.4f", Amount); } else printf("\n No Profit No Loss!"); return 0; }
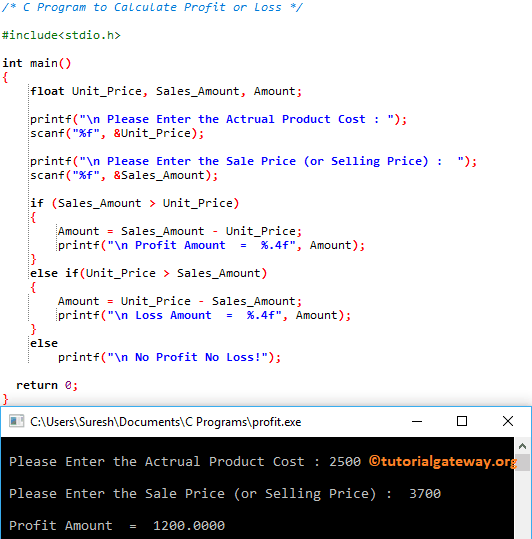
Let me try another value
Please Enter the Actrual Product Cost : 1225.25
Please Enter the Sale Price (or Selling Price) : 1175.69
Loss Amount = 49.5601
another one
Please Enter the Actrual Product Cost : 1400
Please Enter the Sale Price (or Selling Price) : 1400
No Profit No Loss!