How to write a C Program to Calculate Gross Salary of an Employee with an example using Else If Statement?.
C Program to Calculate Gross Salary of an Employee Example
This program helps the user to enter any basic salary of an Employee and then calculate the total salary in C.
For the C Programming demonstration purpose, we are using the following HRA and DA percentages
- If the Basic Salary is less than or equal to 10000 then HRA = 8% of the basic, and DA = 10% of the basic
- Basic Salary is less than or equal to 20000 then HRA = 16% and DA = 20%
- Basic Salary is greater than 20000 then HRA = 24% and DA = 30%
To achieve the same, we are using the Else If Statement.
/* C Program to Calculate Gross Salary of an Employee */ #include <stdio.h> int main() { float Basic, HRA, DA, Gross_Salary; printf("\n Please Enter the Basic Salary of an Employee : "); scanf("%f", &Basic); if (Basic <= 10000) { HRA = (Basic * 8) / 100; // or HRA = Basic * (8 / 100) DA = (Basic * 10) / 100; // Or Da= Basic * 0.1 } else if (Basic <= 20000) { HRA = (Basic * 16) / 100; DA = (Basic * 20) / 100; } else { HRA = (Basic * 24) / 100; DA = (Basic * 30) / 100; } Gross_Salary = Basic + HRA + DA; printf("\n Gross Salary of this Employee = %.2f", Gross_Salary); return 0; }
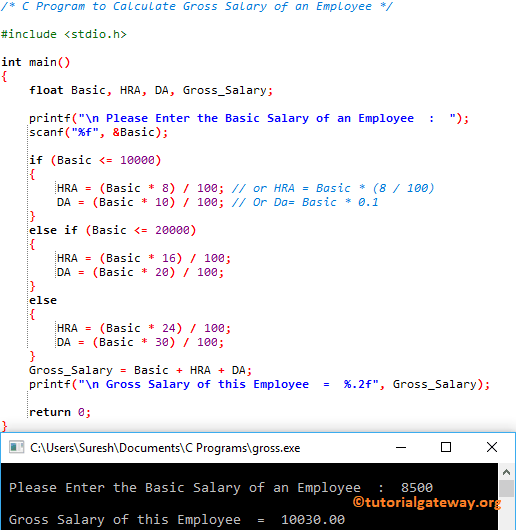
let me try another amount
Please Enter the Basic Salary of an Employee : 35000
Gross Salary of this Employee = 53900.00
C Program to Calculate Gross Salary of an Employee Example 2
This C program allows the user to enter the Basic Salary, HRA percentage, and DA percentage. By using those values, this Program to Calculate Gross Salary of an Employee or calculate the total salary of an employee.
/* C Program to Calculate Gross Salary of an Employee */ #include <stdio.h> int main() { char name[60]; float Basic, HRA_Per, DA_Per, HRA, DA, Gross_Salary; printf("\n Please Enter the Employee Name : "); gets(name); printf(" Please Enter the Basic Salary of an Employee : "); scanf("%f", &Basic); printf(" Please Enter the HRA Percentage of an Employee : "); scanf("%f", &HRA_Per); printf(" Please Enter the DA Percentage of an Employee : "); scanf("%f", &DA_Per); HRA = Basic * (HRA_Per /100); DA = Basic * (DA_Per / 100); Gross_Salary = Basic + HRA + DA; printf("\n Name = %s \n Basic Salary = %.2f \n HRA Amount = %.2f \n DA Amount = %.2f \n Gross Salary = %.2f", name, Basic, HRA, DA, Gross_Salary); return 0; }
Please Enter the Employee Name : Tutorial Gateway
Please Enter the Basic Salary of an Employee : 35000
Please Enter the HRA Percentage of an Employee : 25
Please Enter the DA Percentage of an Employee : 35
Name = Tutorial Gateway
Basic Salary = 35000.00
HRA Amount = 8750.00
DA Amount = 12250.00
Gross Salary = 56000.00
Program to Calculate Gross Salary of an Employee 3
This program is the same as above, but this time we are asking the user to enter Basic Salary, HRA Amount, and DA Amount.
/* C Program to Calculate Gross Salary of an Employee */ #include <stdio.h> int main() { char name[60]; float Basic, HRA, DA, Gross_Salary; printf("\n Please Enter the Employee Name : "); gets(name); printf(" Please Enter the Basic Salary of an Employee : "); scanf("%f", &Basic); printf(" Please Enter the HRA Amount of an Employee : "); scanf("%f", &HRA); printf(" Please Enter the DA Amount of an Employee : "); scanf("%f", &DA); Gross_Salary = Basic + HRA + DA; printf("\n Name = %s \n Basic Salary = %.2f \n HRA Amount = %.2f \n DA Amount = %.2f \n Gross Salary = %.2f", name, Basic, HRA, DA, Gross_Salary); return 0; }
Please Enter the Employee Name : Tutorial Gateway
Please Enter the Basic Salary of an Employee : 25500
Please Enter the HRA Amount of an Employee : 4500
Please Enter the DA Amount of an Employee : 3200
Name = Tutorial Gateway
Basic Salary = 25500.00
HRA Amount = 4500.00
DA Amount = 3200.00
Gross Salary = 33200.00