How to write a C Program to Print Even Numbers from 1 to N using For Loop and While Loop?.
C Program to Print Even Numbers from 1 to N using For Loop
This program allows the user to enter the maximum limit value. And then, it is going to print the list of all even numbers from 1 to user-entered value.
/* C Program to Print Even Numbers from 1 to N using For Loop and If */ #include<stdio.h> int main() { int i, number; printf("\n Please Enter the Maximum Limit Value : "); scanf("%d", &number); printf("\n Even Numbers between 1 and %d are : \n", number); for(i = 1; i <= number; i++) { if ( i % 2 == 0 ) { printf(" %d\t", i); } } return 0; }
C Even Numbers from 1 to N using For Loop output
Please Enter the Maximum Limit Value : 10
Even Numbers between 1 and 10 are :
2 4 6 8 10
Within this C Program to Print Even Numbers from 1 to 100 example, For Loop will make sure that the number is between 1 and maximum limit value.
for(i = 1; i <= number; i++)
In the Next line, We declared the If statement
if ( i % 2 == 0 )
Any number that is divisible by 2 is even number. If condition will check whether the remainder of the number divided by 2 is exactly equal to 0 or not. If the condition is True, it is an Even number, and the compiler will print i value.
C Program to Print Even Numbers from 1 to N without If Statement
This program to Print Even Numbers from 1 to n is the same as above. But we altered for loop to eliminate the If statement.
If you observe the below C Programming code snippet, We started i from 2 and incremented it by 2 (not 1). It means, for the first iteration, i will be 2, and for the second iteration, i will be 4 (not 3), etc.
#include<stdio.h> int main() { int i, number; printf("\n Please Enter the Maximum Limit Value : "); scanf("%d", &number); printf("\n Between 1 and %d are : \n", number); for(i = 2; i <= number; i= i+2) { printf(" %d\t", i); } return 0; }
Please Enter the Maximum Limit Value : 25
Between 1 and 25 are :
2 4 6 8 10 12 14 16 18 20 22 24
C Program to Print Even Numbers from 1 to 100 using While Loop
This program to Print Even Numbers from 1 to N is the same as above. We just replaced the For Loop with While Loop.
#include<stdio.h> int main() { int i = 2, number; printf("\n Please Enter the Maximum Limit Value : "); scanf("%d", &number); printf("\n Between 1 and %d are : \n", number); while(i <= number) { printf(" %d\t", i); i = i+2; } return 0; }
C Even Numbers from 1 to 100 using a While Loop output
Please Enter the Maximum Limit Value : 100
Between 1 and 100 are :
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 78 80 82 84 86 88 90 92 94 96 98
C Program to display Even Numbers in a Given Range
This program allows the user to enter Minimum and maximum value. Next, the C program will display the list of all even numbers between Minimum value and maximum value.
/* C Program to Print Even Numbers within a Range */ #include<stdio.h> int main() { int i, Minimum, Maximum; printf("\n Please Enter the Minimum Limit Value : "); scanf("%d", &Minimum); printf("\n Please Enter the Maximum Limit Values : "); scanf("%d", &Maximum); if ( Minimum % 2 != 0 ) { Minimum++; } printf("\n Even Numbers between %d and %d are : \n", Minimum, Maximum); for(i = Minimum; i <= Maximum; i= i+2) { printf(" %d\t", i); } return 0; }
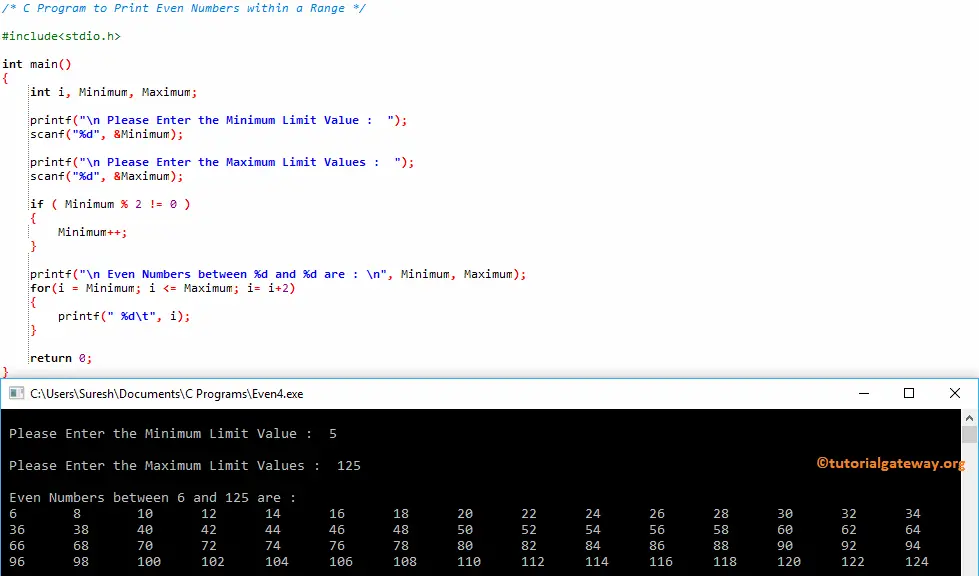