Write a Java program for the largest of three numbers using the Else If Statement, Nested If, and Conditional Operator. There are many approaches to finding the largest numbers among the three, but we use these programs.
Java Program for Largest of Three Numbers using Else If
This program helps the user enter three different values and find the largest number among those three using the Else If Statement.
// using else if package SimpleNumberPrograms; import java.util.Scanner; public class LargestUsingElseIf { private static Scanner sc; public static void main(String[] args) { int x, y, z; sc = new Scanner(System.in); System.out.println("\n Please Enter three Different Value: "); x = sc.nextInt(); y = sc.nextInt(); z = sc.nextInt(); if (x > y && x > z) { System.out.format("\n% d is Greater Than both %d and %d", x, y, z); } else if (y > x && y > z) { System.out.format("\n% d is Greater Than both %d and %d", y, x, z); } else if (z > x && z > y) { System.out.format("\n% d is Greater Than both %d and %d", z, x, y); } else { System.out.println("\n Either any two values or all the three values are equal"); } } }
Java Program for Largest of Three Numbers Analysis
- The first if condition within the Else If Statement checks whether x is greater than y and x is greater than z. if the condition is True, then x is greater than y, z.
- The first Else if condition checks whether y is greater than x and y is greater than z. if it is True, then y is greater than both x and z.
- The second Else if condition checks whether z is greater than x and z is greater than y. if it is True, then z is greater than x and y.
- If all the above conditions fail, it means they are equal.
OUTPUT 1: Lets enter the values x = 50, y = 75, z = 98
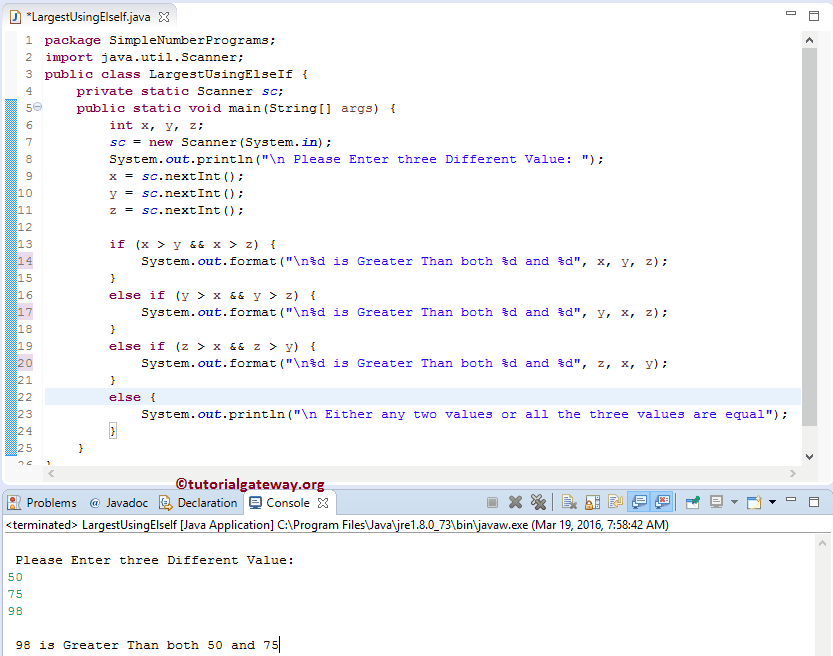
Lets enter the values 15, 5, and 4
Please Enter three Different Value:
15
5
4
15 is Greater Than both 5 and 4
OUTPUT 3
Please Enter three Different Value:
15
60
4
60 is Greater Than both 15 and 4
OUTPUT4
Please Enter three Different Value:
15
15
11
Either any two values or all the three values are equal
Java Program for Largest of Three Numbers using Nested If Statement
This Java program finds the largest numbers among those three using the Nested If statement.
package SimpleNumberPrograms; import java.util.Scanner; public class LargUsingNestedIf { private static Scanner sc; public static void main(String[] args) { int x, y, z; sc = new Scanner(System.in); System.out.println("\n Please Enter three Different Value: "); x = sc.nextInt(); y = sc.nextInt(); z = sc.nextInt(); LarOfTh(x, y, z); } public static void LarOfTh(int x, int y, int z) { if (x - y > 0 && x - z > 0) { System.out.format("\n% d is Greater Than both %d and %d", x, y, z); } else { if (y -z > 0) { System.out.format("\n% d is Greater Than both %d and %d", y, x, z); } else { System.out.format("\n% d is Greater Than both %d and %d", z, x, y); } } } }
Java Program for Largest of Three Numbers Analysis
- The first Java if condition checks whether (x – y) is greater than 0 and (x – z) is greater than 0. This condition fails if we subtract a small number from a big one. Otherwise, it will be True. If this condition is True, x is greater than y and z.
- Else statement will execute when the first if condition is False. So, there is no need to check for the x value. In the Else statement, we insert one more if condition (Nested IF) to check whether (y – z) is greater than 0. If it is True, y is greater than both x and z.
- Else, z is greater than both x and y.
OUTPUT: Lets enter the values x = 15, y = 87, z = 26.
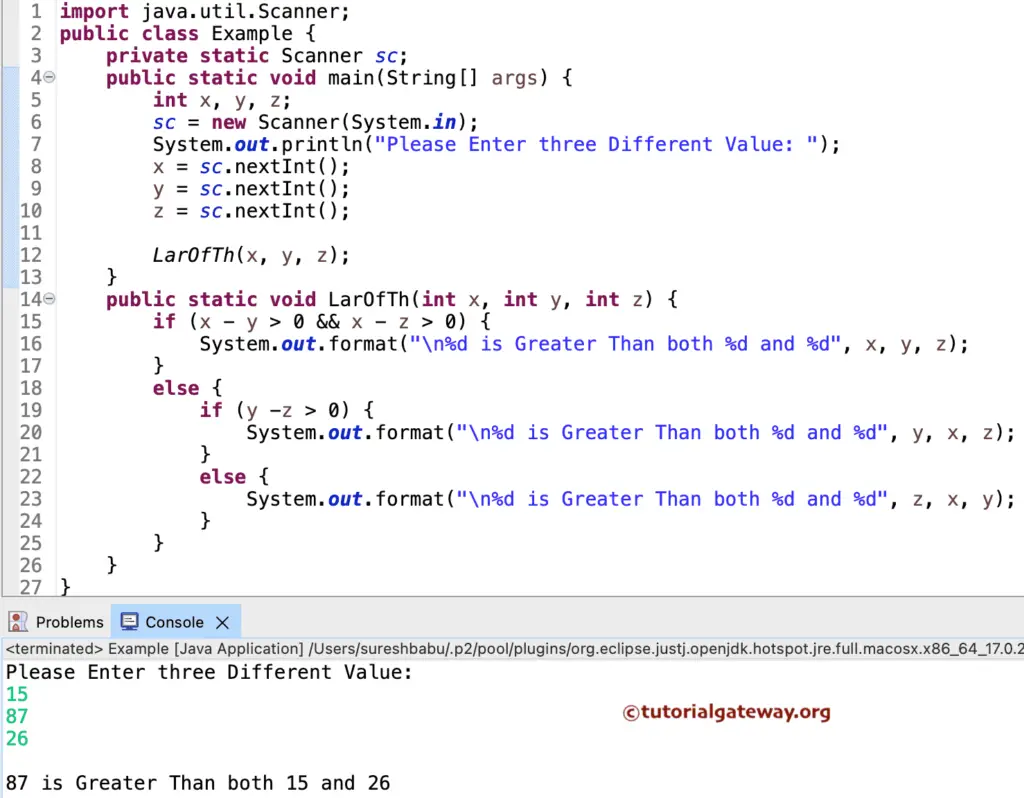
Java Program for Largest of Three Numbers using Ternary Operator
This Java program finds the largest number among those three numbers using the Ternary Operator
package SimpleNumberPrograms; import java.util.Scanner; public class LagUsingConditionalOper { private static Scanner sc; public static void main(String[] args) { int x, y, z, lar; sc = new Scanner(System.in); System.out.println("\n Please Enter three Different Value: "); x = sc.nextInt(); y = sc.nextInt(); z = sc.nextInt(); lar = ((x > y && x > z)? x: (y > z)?y:z); System.out.format("\n Largest number among three is: %d", lar); } }
Java Program for Largest of Three Numbers Analysis
- In this example, the first condition checks whether x is greater than y and x is greater than z. If this condition is True, will it return the first value after the ? symbol, variable x (x is greater than y, z).
- If the first condition fails, it will execute the variable after the : symbol. We check one more condition here (y > z) using the Nested conditional operator. If this condition is True, will it return the first value after the? Symbol, variable y (y is greater than both x, z).
- If the Nested condition fails, it will execute the variable after the: symbol, variable z. It means z is greater than both x and y.
OUTPUT 1: Let us enter the values 15, 6, and 9.
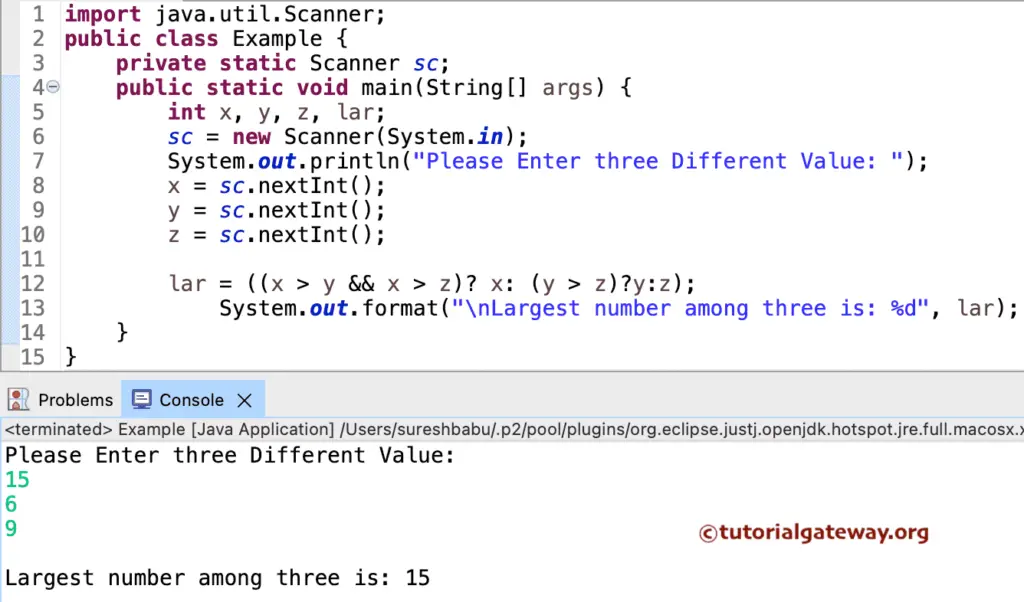
Let’s enter the different values
Please Enter three Different Value:
5
98
87
Largest number among three is: 98
OUTPUT 3:
Please Enter three Different Value:
100
500
980
Largest number among three is: 980
Java Program for Largest of Three Numbers using Oops
In this example, we divide the code using Object-Oriented Programming. To do this, we will create a class with two methods to find the largest of the two numbers.
package SimpleNumberPrograms; public class LargestNumber { int x, y, z, largest; public void LOT() { if (x - y > 0 && x - z > 0) { System.out.format("\n% d is Greater Than both %d and %d", x, y, z); } else { if (y -z > 0) { System.out.format("\n% d is Greater Than both %d and %d", y, x, z); } else { System.out.format("\n% d is Greater Than both %d and %d", z, x, y); } } } public int LOTNumber(int x, int y, int z) { largest = ((x > y && x > z)? x: (y > z)?y:z); return largest; } }
Within the Main Java Program for Largest of Three Numbers, we will create an instance of the above class and call the methods.
package SimpleNumberPrograms; import java.util.Scanner; public class LargestUsingClass { private static Scanner sc; public static void main(String[] args) { int a, b, c, largest; sc = new Scanner(System.in); System.out.println("\n Please Enter three Different Value: "); a = sc.nextInt(); b = sc.nextInt(); c = sc.nextInt(); LargestNumber ln = new LargestNumber(); ln.x = a; ln.y = b; ln.z = c; ln.LOT(); largest = ln.LOTNumber(a, b, c); System.out.format("\n Largest number among three is = %d", largest); } }
Please Enter three Different Value:
45
75
25
75 is Greater Than both 45 and 25
Largest number among three is: 75
LargestNumber Class Analysis:
- First, we declared a function LOT with zero arguments. Then, we used the Nested If statement within the function to find the largest of the three numbers. Finally, we used System.out.println statements within the function to print the output.
- Next, we declared an integer function LOTNumbers, with three arguments. We already explained the Logic in the above example.
Java Program for Largest of Three Numbers Main Class Analysis:
Within this program, we created an instance/created an Object of the LargestNumber Class
LargestNumber ln = new LargestNumber();
Next, we assign the user-entered values to the LargestNumber Class variables.
ln.x = a; ln.y = b; ln.z = c;
Next, we are calling the LOT method. Note that this is the first method we created with the void keyword, which will find the largest of three numbers and print the output from the LargestNumber Class itself.
ln.LOT();
Next, we are calling the LOTNumbers method by passing three arguments. This Java function will find the largest of three numbers in a program and return the largest value. That is why we are assigning the output to the largest variable.
largest = ln.LOTNumber(a, b, c);
Lastly, the System.out.println statement will print the output.