Write a Java string reverse program using recursion or recursive functions. In this example, the user-defined method checks whether the string is empty, and if it’s true, it prints the exact same or empty space. Otherwise, it performs the string reverse recursively.
package RemainingSimplePrograms; import java.util.Scanner; public class StringReverseRecur1 { private static Scanner sc; public static void main(String[] args) { sc= new Scanner(System.in); System.out.print("Please Enter String to Reverse = "); String str = sc.nextLine(); String revString = stringReverse(str); System.out.println("The reversed String = " + revString); } public static String stringReverse(String str) { if(str.isEmpty()) return str; return stringReverse(str.substring(1)) + str.charAt(0); } }
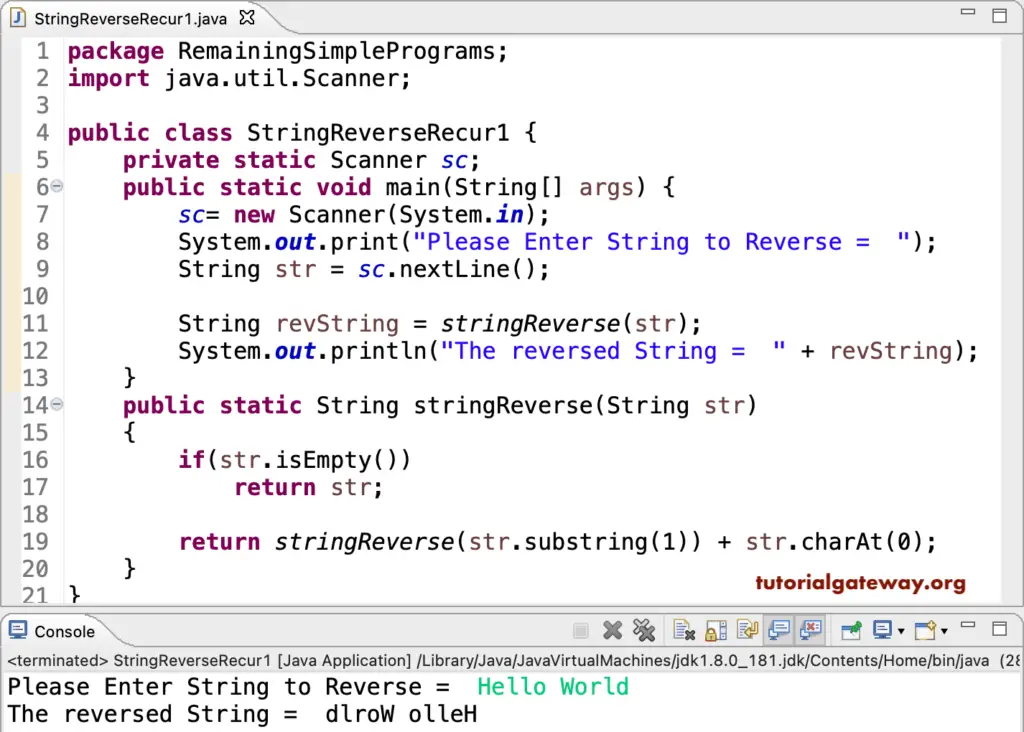
Java String Reverse Program using Recursion
It is another way of writing string reverse programs in Java using recursion.
package RemainingSimplePrograms; import java.util.Scanner; public class Example2 { private static Scanner sc; public static void main(String[] args) { sc= new Scanner(System.in); System.out.print("Please Enter = "); String str = sc.nextLine(); System.out.print("The Result = " ); strRev(str); } public static void strRev(String str) { if(str.isEmpty()) { System.out.print(str); } else { System.out.print(str.charAt(str.length() - 1)); strRev(str.substring(0, str.length() - 1)); } } }
Please Enter = Welcome to Java World!
The Result = !dlroW avaJ ot emocleW