Write a Java Program to perform Scalar Matrix Multiplication with an example. Or write a Java program to calculate the Scalar Multiplication on a given Multi-dimensional array.
In this Java Scalar Matrix Multiplication example, we declared a Sc_Mat 3 * 3 integer matrix. Next, we allows the user to insert any integer value to perform scalar multiplication. Then, we used for loop to iterate the Matrix, and within that, we performed the multiplication.
import java.util.Scanner; public class ScalarMultiplication { private static Scanner sc; public static void main(String[] args) { int[][] Sc_Mat = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; sc= new Scanner(System.in); int i, j, num; System.out.println("\nPlease Enter the Multiplication Value : "); num = sc.nextInt(); System.out.println("\nMatrix after Scalar Multiplication are :"); for(i = 0; i < Sc_Mat.length ; i++) { for(j = 0; j < Sc_Mat[0].length; j++) { System.out.format("%d \t", (num * Sc_Mat[i][j])); } System.out.print("\n"); } } }
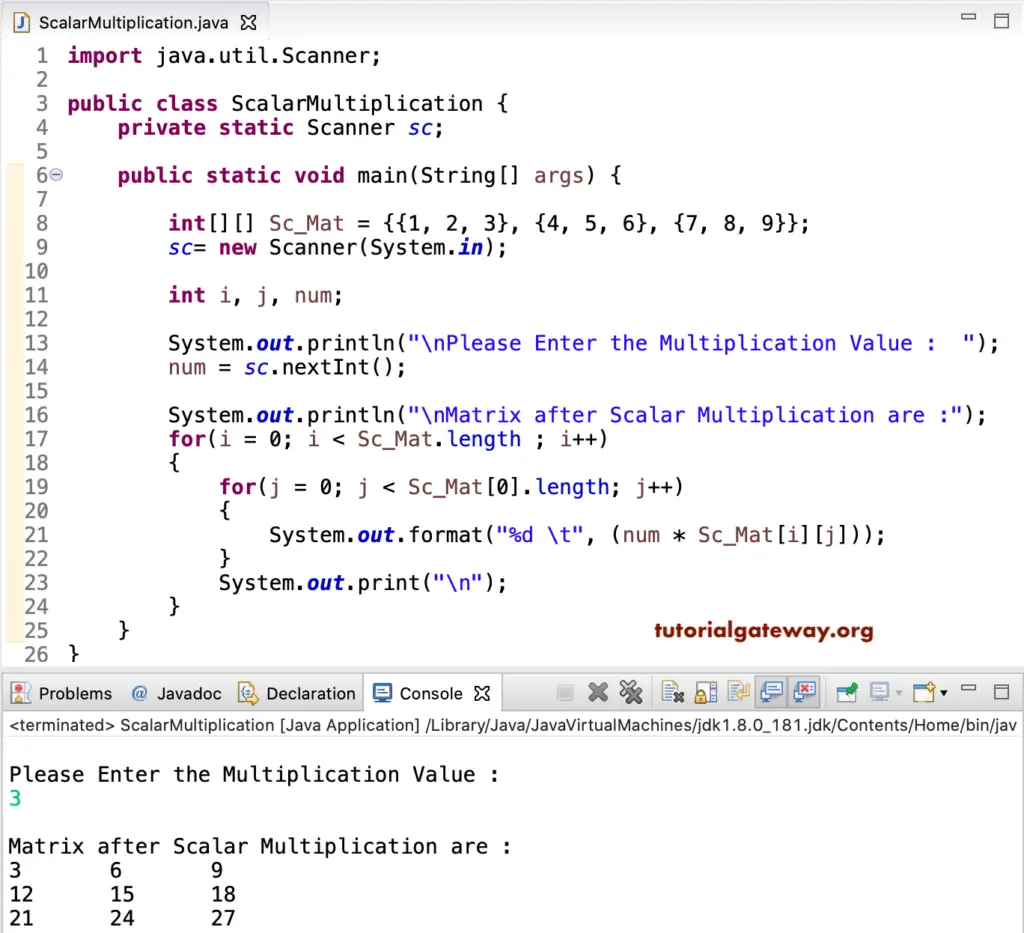
Java Scalar Matrix Multiplication Program example 2
This Java Scalar multiplication of a Matrix code is the same as the above. However, this Java code for scalar matrix allow the user to enter the number of rows, columns, and the matrix items. Please refer to C Program to perform the Scalar Matrix Multiplication article to understand loop execution in iteration-wise.
import java.util.Scanner; public class ScalarMultiplication { private static Scanner sc; public static void main(String[] args) { sc= new Scanner(System.in); int i, j, rows, columns, num; System.out.println("\n Enter Scalar Matrix Rows & Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] Sc_Mat = new int[rows][columns]; System.out.println("\n Enter the First Matrix Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { Sc_Mat[i][j] = sc.nextInt(); } } System.out.println("\nPlease Enter the Multiplication Value : "); num = sc.nextInt(); System.out.println("\nMatrix after Scalar Multiplication are :"); for(i = 0; i < Sc_Mat.length ; i++) { for(j = 0; j < Sc_Mat[0].length; j++) { System.out.format("%d \t", (num * Sc_Mat[i][j])); } System.out.print("\n"); } } }
Enter Scalar Matrix Rows & Columns :
3 3
Enter the First Matrix Items :
10 20 30
40 60 80
90 11 55
Please Enter the Multiplication Value :
4
Matrix after Scalar Multiplication are :
40 80 120
160 240 320
360 44 220