Write a Java Program to Swap Two Numbers using a temporary variable and without a temporary or third variable. We will use temp variables, Arithmetic Operators, and Bitwise Operators for this program.
Java Program to Swap Two Numbers using Temp Variable
This Java program allows the user to enter two integer values. By using the third variable and the assignment operator, this example Swaps those two numbers.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int a, b, temp; sc = new Scanner(System.in); System.out.print(" Please Enter the First : "); a = sc.nextInt(); System.out.print(" Please Enter the Second : "); b = sc.nextInt(); System.out.println("\n Before : a = " + a + " and b = " + b); temp = a; a = b; b = temp; System.out.println("\n After : a = " + a + " and b = " + b); } }
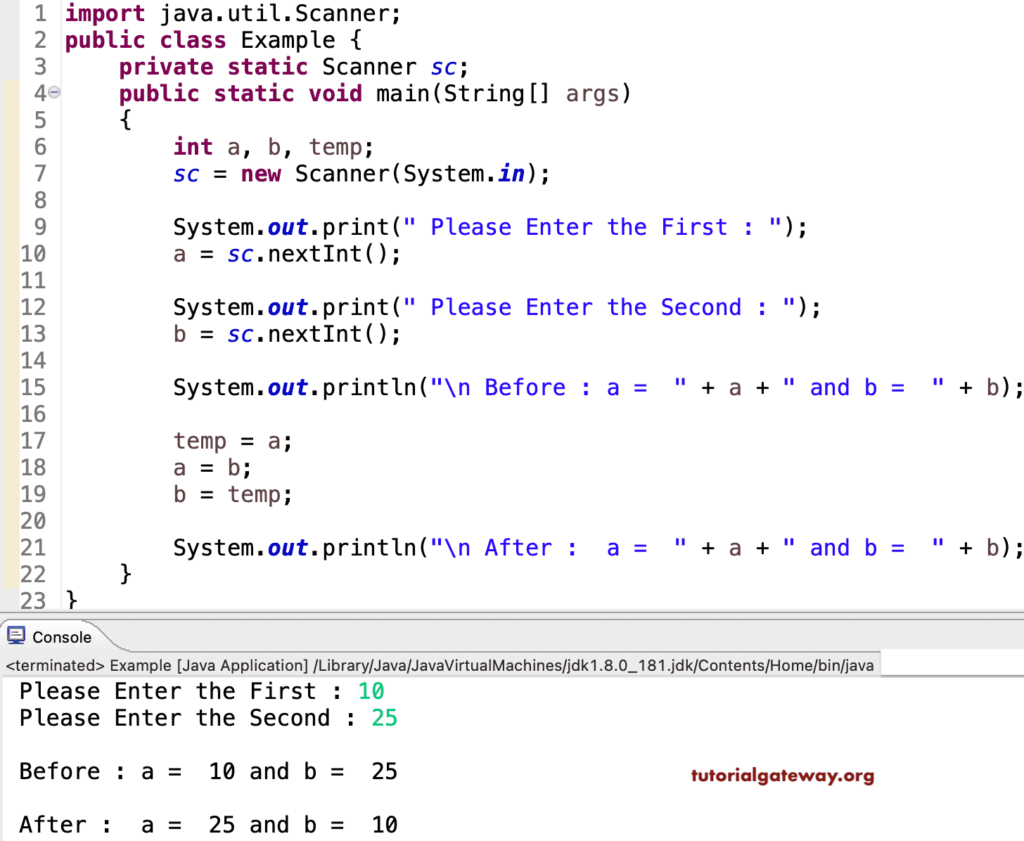
In the above example, we assigned a = 10 and b = 25.
Temp = a – it means assigning a value to the Temp variable.
Temp = 10
a = b – assigning b value to the variable a.
a = 25
b = Temp – assigning Temp value to b.
b = 6
Java Program to Swap Two Numbers without Using Temp Variable
In this example, Instead of using the temp or third variable to swap two numbers, we will use Arithmetic Operators to perform addition and subtraction.
import java.util.Scanner; public class Example2 { private static Scanner sc; public static void main(String[] args) { int a, b; sc = new Scanner(System.in); System.out.print(" Please Enter the First value : "); a = sc.nextInt(); System.out.print(" Please Enter the Second value : "); b = sc.nextInt(); System.out.println("\n Before : a = " + a + " and b = " + b); a = a + b; b = a - b; a = a - b; System.out.println("\n After : a = " + a + " and b = " + b); } }
Using arithmetic operators result.
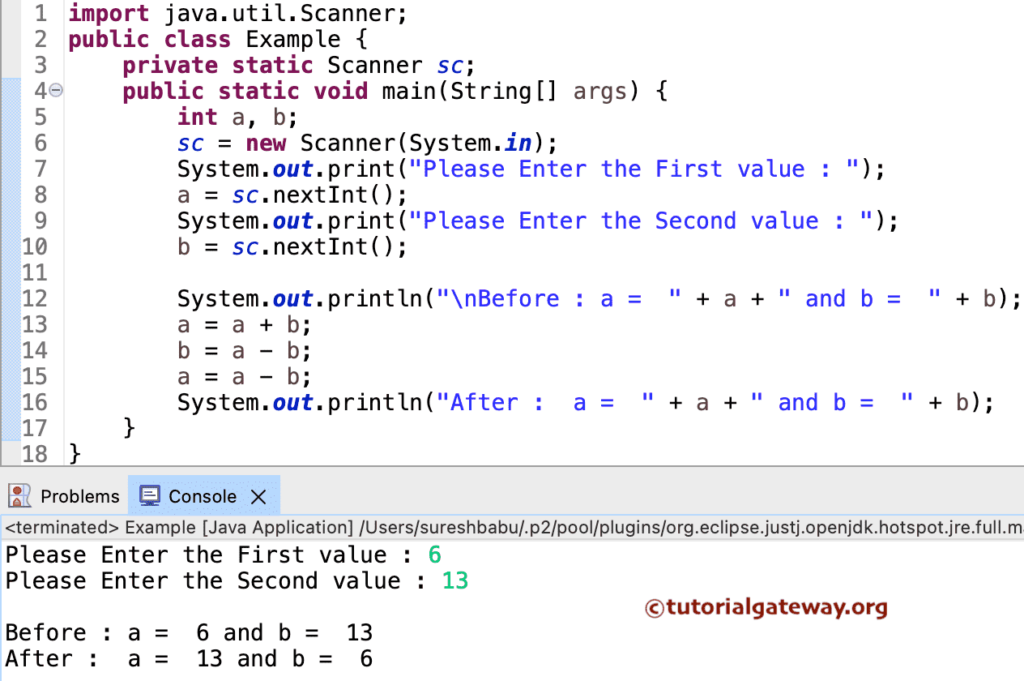
User Entered Values are a = 6 and b = 13
a = a + b
a = 6 + 13 = 19
b= a-b = 19 – 13 = 6
a = a-b = 19 – 6 = 13
Final Values of the 2 numbers: a = 13 and b = 6
NOTE: We can interchange 2 numbers using the multiplication and division approach. But it may produce strange values if we work with larger integer values or if any of them is 0.
Java Program to Swap Two Numbers using Bitwise OR Operator
In this example, we will use Bitwise Operators XOR, instead of using the temp variable to swap 2 numbers.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { int a, b; sc = new Scanner(System.in); System.out.print(" Please Enter the First value : "); a = sc.nextInt(); System.out.print(" Please Enter the Second value : "); b = sc.nextInt(); System.out.println("\n Before : a = " + a + " and b = " + b); a = a ^ b; b = a ^ b; a = a ^ b; System.out.println("\n After : a = " + a + " and b = " + b); } }
Output
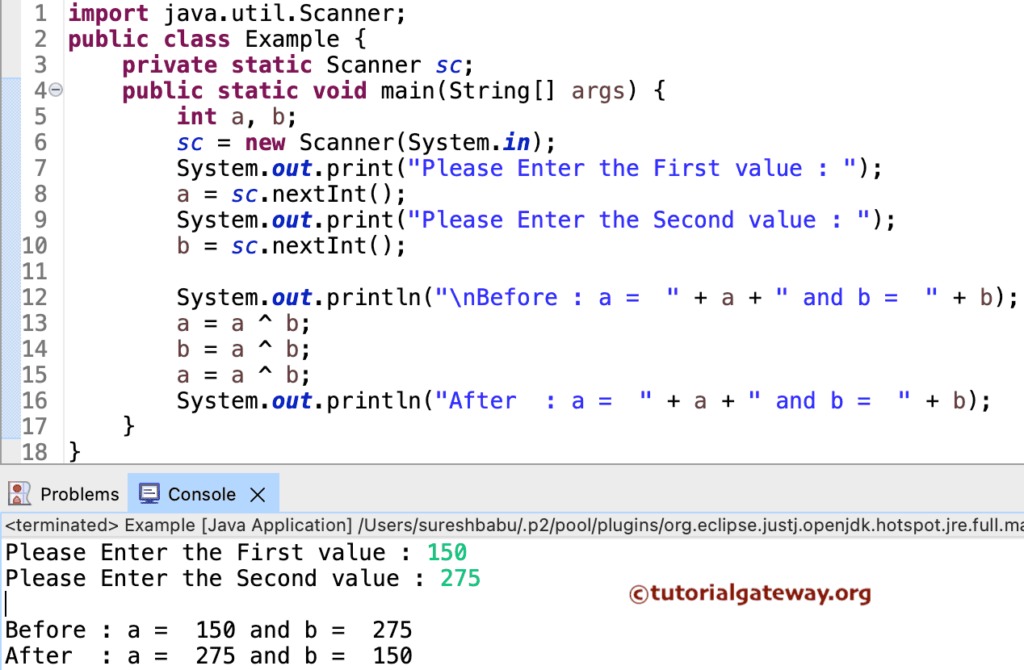
Java Program to Swap Two Numbers Using Functions
This program is the same as the first Java example of temporary variables. However, we separated the logic and placed it in a separate method.
import java.util.Scanner; public class Example4 { private static Scanner sc; public static void main(String[] args) { int a, b; sc = new Scanner(System.in); System.out.print(" Please Enter the First value : "); a = sc.nextInt(); System.out.print(" Please Enter the Second value : "); b = sc.nextInt(); swfunc(a, b); } public static void swfunc(int a, int b) { int temp; temp = a; a = b; b = temp; System.out.println("\n After : a = " + a + " and b = " + b); } }
Please Enter the First value : 12
Please Enter the Second value : 95
After : a = 95 and b = 12