Write a Java program to print right triangle of consecutive row alphabets pattern using for loop.
package Alphabets; import java.util.Scanner; public class RightTriConsecRowAlp1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Right Triangle of Consec Row Alphabets Rows = "); int rows = sc.nextInt(); System.out.println("Right Triangle of Consecutive Row Alphabets Pattern"); int alphabet = 64; for (int i = 1; i <= rows; i++) { int val = i; for (int j = 1; j <= i; j++ ) { System.out.print((char)(alphabet+ val) + " "); val = val + rows - j; } System.out.println(); } } }
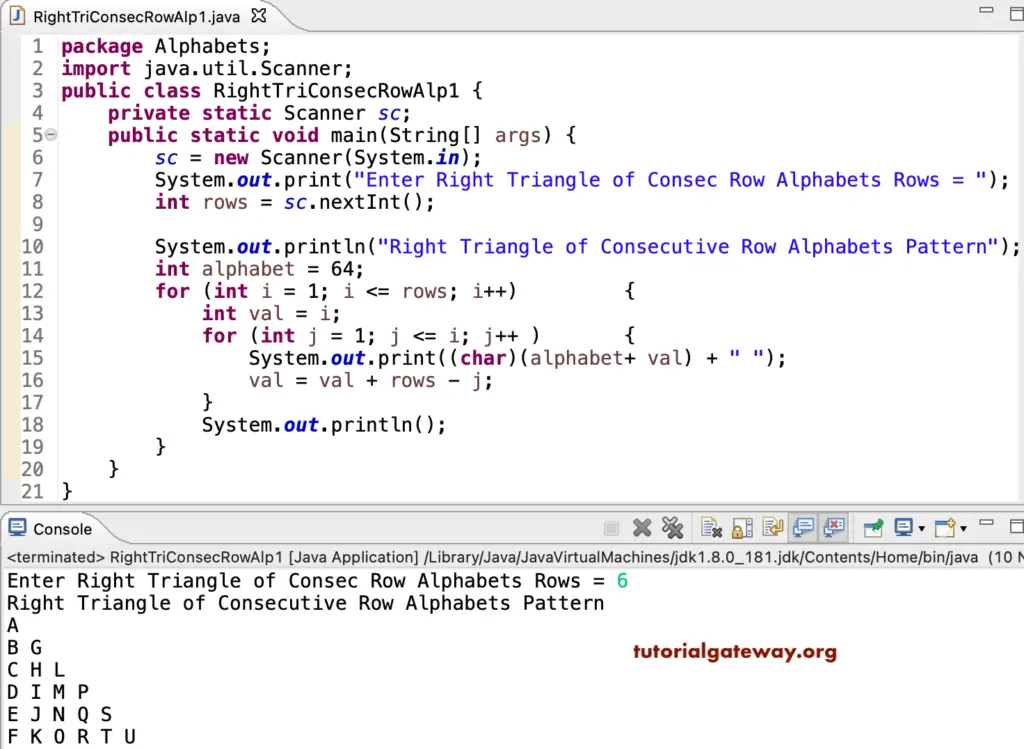
This Java pattern example displays the right angled triangle of consecutive alphabets in each row using while loop.
package Alphabets; import java.util.Scanner; public class RightTriConsecRowAlp2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Right Triangle of Consecutive Row Alphabets Rows = "); int rows = sc.nextInt(); System.out.println("Right Triangle of Consecutive Row Alphabets Pattern"); int alphabet = 64; int val, j, i = 1; while (i <= rows) { val = i; j = 1; while( j <= i ) { System.out.print((char)(alphabet+ val) + " "); val = val + rows - j; j++; } System.out.println(); i++; } } }
Enter Right Triangle of Consecutive Row Alphabets Rows = 8
Right Triangle of Consecutive Row Alphabets Pattern
A
B I
C J P
D K Q V
E L R W [
F M S X \ _
G N T Y ] ` b
H O U Z ^ a c d
This Java program uses the do while loop to display the right angled triangle pattern of consecutive row alphabets.
package Alphabets; import java.util.Scanner; public class RightTriConsecRowAlp3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Right Triangle of Consecutive Row Alphabets Rows = "); int rows = sc.nextInt(); System.out.println("Right Triangle of Consecutive Row Alphabets Pattern"); int alphabet = 64; int val, j, i = 1; do { val = i; j = 1; do { System.out.print((char)(alphabet+ val) + " "); val = val + rows - j; } while( ++j <= i ); System.out.println(); } while (++i <= rows); } }
Enter Right Triangle of Consecutive Row Alphabets Rows = 10
Right Triangle of Consecutive Row Alphabets Pattern
A
B K
C L T
D M U \
E N V ] c
F O W ^ d i
G P X _ e j n
H Q Y ` f k o r
I R Z a g l p s u
J S [ b h m q t v w