Write a Java program to print right triangle characters pattern using for loop.
package Alphabets; import java.util.Scanner; public class RightTriofChars1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Right Triangle of Characters Rows = "); int rows = sc.nextInt(); System.out.println("Right Angled Triangle of Characters Pattern"); int alphabet = 65; for (int i = 1; i <= rows; i++) { for (int j = 0; j <= (2 * i - 2); j++ ) { System.out.print((char)(alphabet + j) + " "); } System.out.println(); } } }
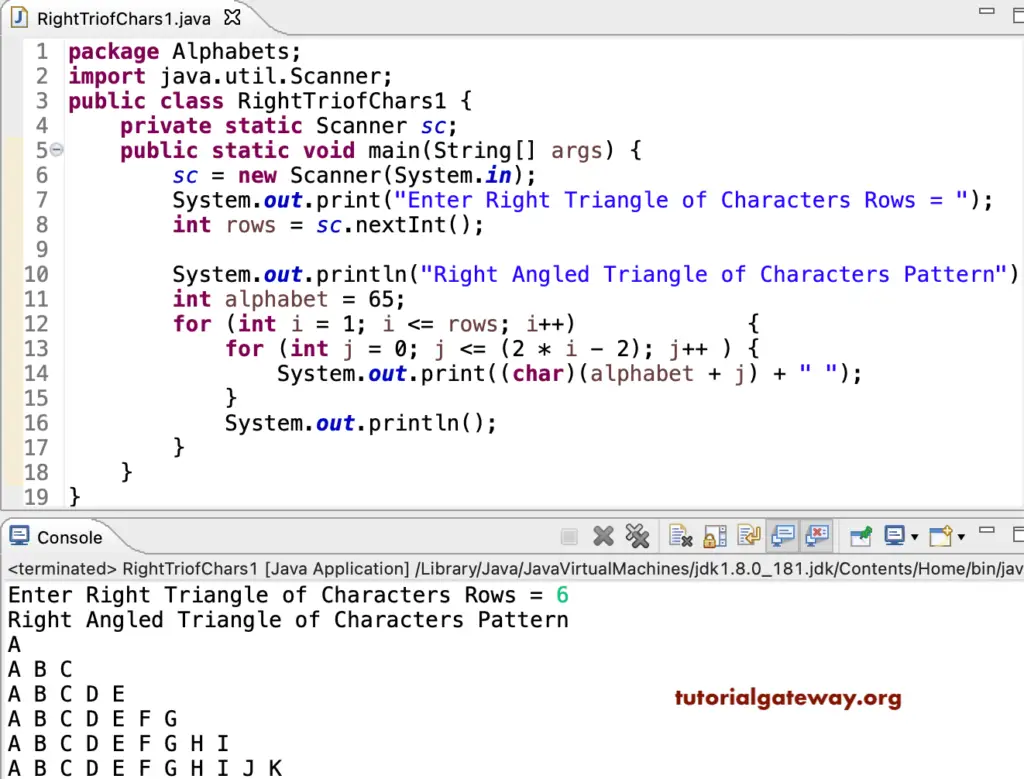
Java program to print right triangle characters pattern using while loop.
package Alphabets; import java.util.Scanner; public class RightTriofChars2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Rows = "); int rows = sc.nextInt(); System.out.println("Right Angled Triangle of Characters Pattern"); int alphabet = 65; int j, i = 1; while( i <= rows) { j = 0; while( j <= (2 * i - 2)) { System.out.print((char)(alphabet + j) + " "); j++; } System.out.println(); i++; } } }
Enter Rows = 13
Right Angled Triangle of Characters Pattern
A
A B C
A B C D E
A B C D E F G
A B C D E F G H I
A B C D E F G H I J K
A B C D E F G H I J K L M
A B C D E F G H I J K L M N O
A B C D E F G H I J K L M N O P Q
A B C D E F G H I J K L M N O P Q R S
A B C D E F G H I J K L M N O P Q R S T U
A B C D E F G H I J K L M N O P Q R S T U V W
A B C D E F G H I J K L M N O P Q R S T U V W X Y
This Java example prints the right angled triangle pattern of characters using while loop.
package Alphabets; import java.util.Scanner; public class RightTriofChars3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Rows = "); int rows = sc.nextInt(); System.out.println(""); int alphabet = 65; int j, i = 1; do { j = 0; do { System.out.print((char)(alphabet + j) + " "); } while( ++j <= (2 * i - 2)); System.out.println(); } while(++i <= rows); } }
Enter Rows = 16
A
A B C
A B C D E
A B C D E F G
A B C D E F G H I
A B C D E F G H I J K
A B C D E F G H I J K L M
A B C D E F G H I J K L M N O
A B C D E F G H I J K L M N O P Q
A B C D E F G H I J K L M N O P Q R S
A B C D E F G H I J K L M N O P Q R S T U
A B C D E F G H I J K L M N O P Q R S T U V W
A B C D E F G H I J K L M N O P Q R S T U V W X Y
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ]
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ] ^ _