Write a Java Program to print right arrow star pattern using for loop. This Java right arrow star example divides the code into two parts, the first part (nested for loop) prints the top part of the right arrow, and the other code prints the bottom.
package ShapePrograms; import java.util.Scanner; public class RightArrow1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j; System.out.print("Please Enter Right Arrow Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Right Arrow Pattern of Stars"); for (i = 0 ; i < rows; i++ ) { for (j = 0 ; j < rows; j++ ) { if(j < i) { System.out.print(" "); } else { System.out.print("*"); } } System.out.println(); } for (i = 2 ; i <= rows; i++ ) { for (j = 0 ; j < rows; j++ ) { if(j < rows - i) { System.out.print(" "); } else { System.out.print("*"); } } System.out.println(); } } }
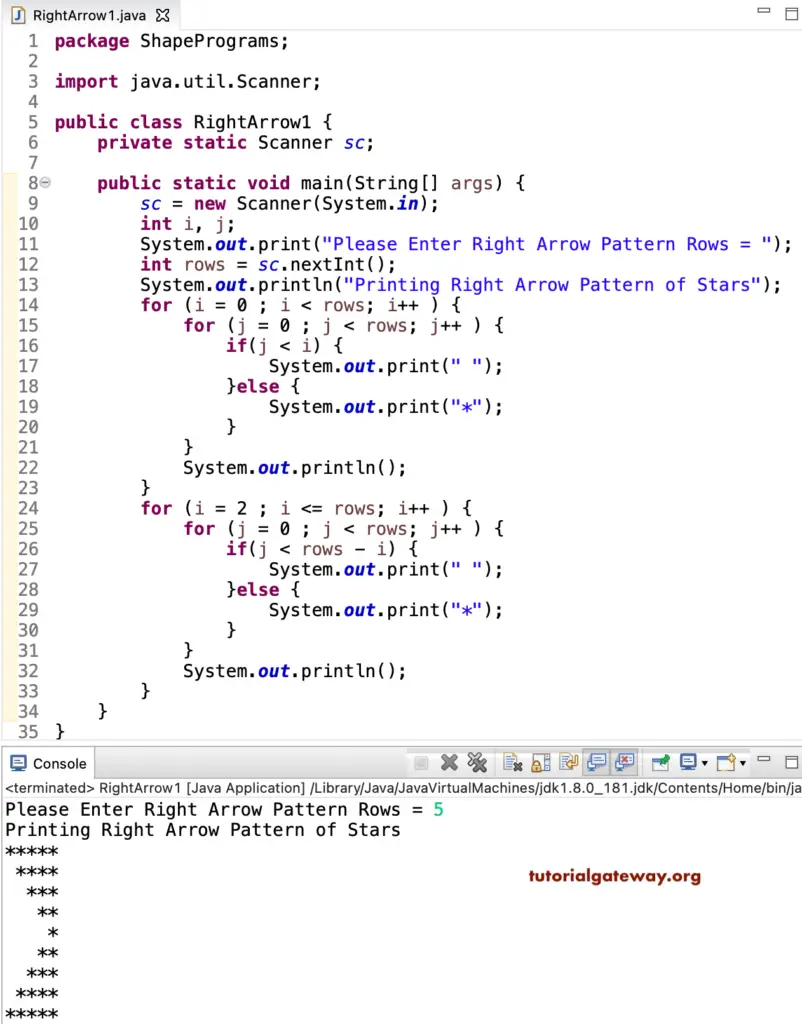
In this Java right arrow star pattern program, we replaced the for loop with a while loop.
package ShapePrograms; import java.util.Scanner; public class RightArrow2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j; System.out.print("Please Enter Right Arrow Pattern Rows = "); int rows = sc.nextInt(); System.out.println("---- Printing Right Arrow Pattern of Stars ------"); i = 0 ; while ( i < rows ) { j = 0 ; while( j < rows) { if(j < i) { System.out.print(" "); } else { System.out.print("*"); } j++; } System.out.println(); i++; } i = 2 ; while( i <= rows ) { j = 0 ; while( j < rows) { if(j < rows - i) { System.out.print(" "); } else { System.out.print("*"); } j++; } System.out.println(); i++; } } }
Please Enter Right Arrow Pattern Rows = 6
---- Printing Right Arrow Pattern of Stars ------
******
*****
****
***
**
*
**
***
****
*****
******
Java Program to Print Right Arrow Star Pattern using do while loop
package ShapePrograms; import java.util.Scanner; public class RightArrow3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j; System.out.print("Please Enter Right Arrow Pattern Rows = "); int rows = sc.nextInt(); System.out.println("---- Printing Right Arrow Pattern of Stars ------"); i = 0 ; do { j = 0 ; do { if(j < i) { System.out.print(" "); } else { System.out.print("*"); } j++; } while( j < rows); System.out.println(); i++; } while ( i < rows ); i = 2 ; do { j = 0 ; do { if(j < rows - i) { System.out.print(" "); } else { System.out.print("*"); } j++; } while( j < rows) ; System.out.println(); i++; } while( i <= rows ); } }
Please Enter Right Arrow Pattern Rows = 8
---- Printing Right Arrow Pattern of Stars ------
********
*******
******
*****
****
***
**
*
**
***
****
*****
******
*******
********
In this Java example, the RightArrowPattern function prints the right arrow pattern of a given symbol.
package ShapePrograms; import java.util.Scanner; public class RightArrow4 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Right Arrow Pattern Rows = "); int rows = sc.nextInt(); System.out.print("Please Enter Character for Right Arrow Pattern = "); char ch = sc.next().charAt(0); System.out.println("--- Printing Right Arrow Pattern of Stars ----"); RightArrowPattern(rows, ch); } public static void RightArrowPattern(int rows, char ch) { int i, j; for (i = 0 ; i < rows; i++ ) { for (j = 0 ; j < rows; j++ ) { if(j < i) { System.out.print(" "); } else { System.out.print(ch); } } System.out.println(); } for (i = 2 ; i <= rows; i++ ) { for (j = 0 ; j < rows; j++ ) { if(j < rows - i) { System.out.print(" "); } else { System.out.print(ch); } } System.out.println(); } } }
Please Enter Right Arrow Pattern Rows = 10
Please Enter Character for Right Arrow Pattern = #
--- Printing Right Arrow Pattern of Stars ----
##########
#########
########
#######
######
#####
####
###
##
#
##
###
####
#####
######
#######
########
#########
##########