Write a Java Program to print rhombus star pattern using for loop. This Java rhombus star example uses multiple for loops nested inside a for loop to iterate the rhombus rows.
package ShapePrograms; import java.util.Scanner; public class RhombusPattern1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Rhombus Pattern Rows = "); int rows = sc.nextInt(); System.out.println("-- Printing Rhombus Pattern of Stars ---"); for (int i = 1 ; i <= rows; i++ ) { for (int j = 1 ; j <= rows - i; j++ ) { System.out.print(" "); } for (int k = 1 ; k <= rows; k++ ) { System.out.print("*"); } System.out.println(); } } }
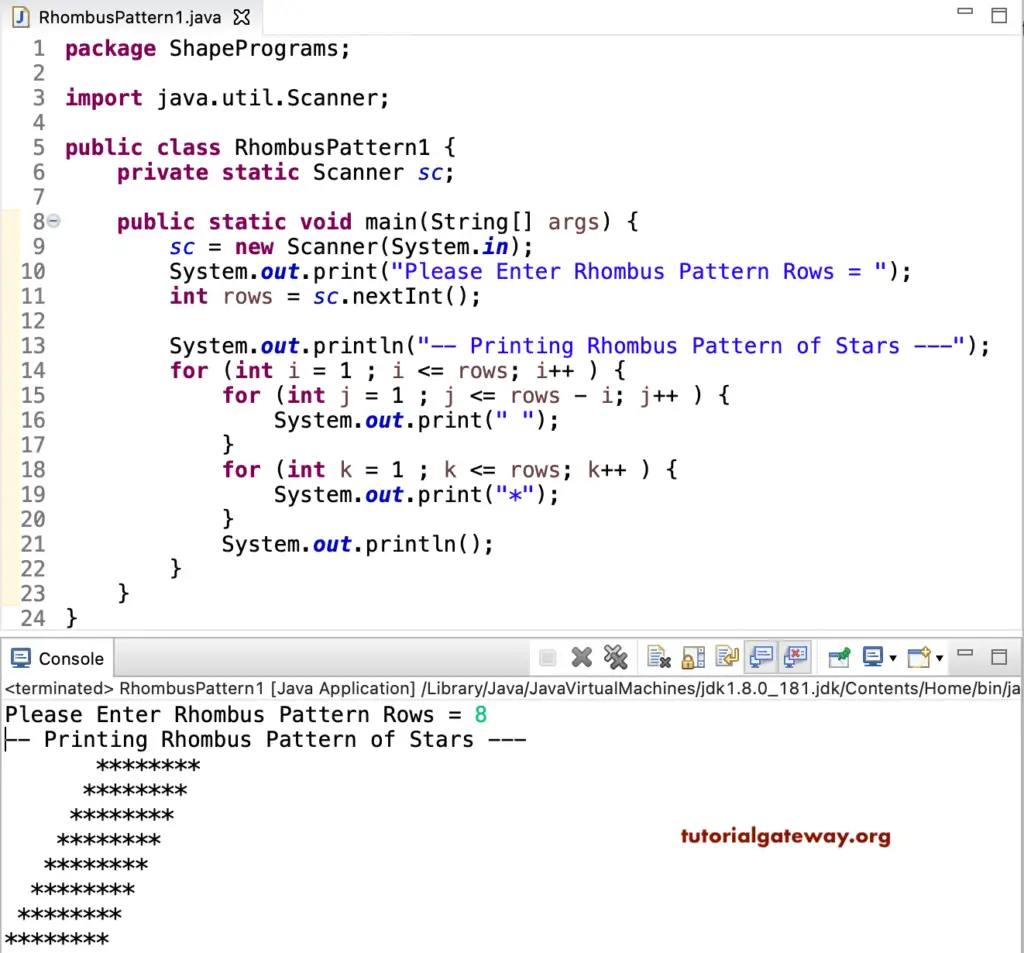
In this Java rhombus star pattern program, we replaced the nested for loop with while loops.
package ShapePrograms; import java.util.Scanner; public class RhombusPattern2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Rhombus Pattern Rows = "); int rows = sc.nextInt(); System.out.println("--- Printing Rhombus Pattern of Stars ---"); int i = 1 ; while( i <= rows ) { int j = 1; while(j <= rows - i) { System.out.print(" "); j++; } int k = 1; while(k <= rows) { System.out.print("*"); k++; } System.out.println(); i++; } } }
Please Enter Rhombus Pattern Rows = 12
--- Printing Rhombus Pattern of Stars ---
************
************
************
************
************
************
************
************
************
************
************
************
Java Program to Print Rhombus Star Pattern using do while loop
package ShapePrograms; import java.util.Scanner; public class RhombusPattern3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Rhombus Pattern Rows = "); int rows = sc.nextInt(); System.out.println("-- Printing Rhombus Pattern of Stars ---"); int i = 1 ; do { int j = 1; do { System.out.print(" "); }while(j++ <= rows - i); int k = 1; do { System.out.print("*"); }while(++k <= rows); System.out.println(); i++; }while(i <= rows); } }
Please Enter Rhombus Pattern Rows = 10
-- Printing Rhombus Pattern of Stars ---
**********
**********
**********
**********
**********
**********
**********
**********
**********
**********
In this Java example, the RhombusPattern function prints the rhombus pattern of a user-given symbol.
package ShapePrograms; import java.util.Scanner; public class RhombusPattern4 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Please Enter Rhombus Pattern Rows = "); int rows = sc.nextInt(); System.out.print("Please Enter Character for Rhombus Pattern = "); char ch = sc.next().charAt(0); System.out.println("---- Printing Rhombus Pattern ------"); RhombusPattern(rows, ch); } public static void RhombusPattern(int rows, char ch) { for (int i = 1 ; i <= rows; i++ ) { for (int j = 1 ; j <= rows - i; j++ ) { System.out.print(" "); } for (int k = 1 ; k <= rows; k++ ) { System.out.print(ch); } System.out.println(); } } }
Please Enter Rhombus Pattern Rows = 15
Please Enter Character for Rhombus Pattern = #
---- Printing Rhombus Pattern ------
###############
###############
###############
###############
###############
###############
###############
###############
###############
###############
###############
###############
###############
###############
###############