Write a Java Program to Print Prime Numbers from 1 to N using For Loop, While Loop, and Functions.
Java Program to Print Prime Numbers from 1 to N using For Loop
This program allows the user to enter any integer value. Next, this Java program displays all the Prime numbers from 1 to 100 using For Loop.
TIP: Please refer Check Prime Number article in Java to understand the steps involved in checking Prime Number
public class PrintPrimeNumbers1 { public static void main(String[] args) { int i, number, count; System.out.println(" Prime Numbers from 1 to 100 are : "); for(number = 1; number <= 100; number++) { count = 0; for (i = 2; i <= number/2; i++) { if(number % i == 0) { count++; break; } } if(count == 0 && number != 1 ) { System.out.print(number + " "); } } } }
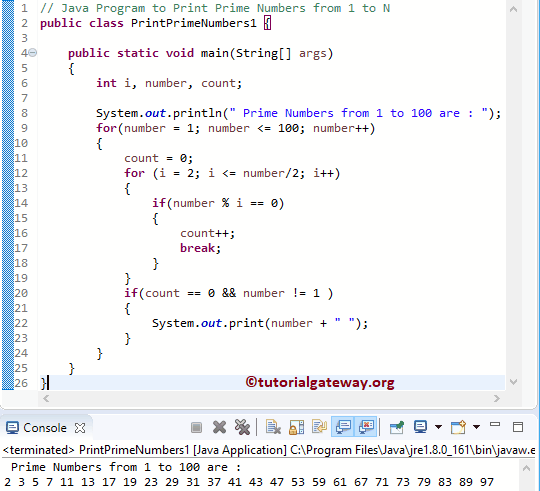
Java Program to Print Prime Numbers from 1 to 100 using While Loop
This program returns the list of prime numbers from 1 to 100 using While Loop.
public class Example2 { public static void main(String[] args) { int i, number = 1, count; System.out.println(" From 1 to 100 are : "); while(number <= 100) { count = 0; i = 2; while(i <= number/2 ) { if(number % i == 0) { count++; break; } i++; } if(count == 0 && number != 1 ) { System.out.print(number + " "); } number++; } } }
We just replaced the For loop in the above example with the While loop. If you don’t understand the While Loop, please refer to WHILE LOOP.
Program to print prime numbers from 1 to 100 using a while loop output
From 1 to 100 are :
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
Java Program to display Prime Numbers from 1 to N using Method
This program is the same as the first example. Still, we separated the logic of the prime numbers and placed them in a separate method.
import java.util.Scanner; public class Example3 { private static Scanner sc; public static void main(String[] args) { int number, minimum, maximum, i, count; sc = new Scanner(System.in); System.out.print(" Please Enter the Minimum value : "); minimum = sc.nextInt(); System.out.print(" Please Enter the Maximum value : "); maximum = sc.nextInt(); System.out.println(" Prime Numbers from 1 to 100 are : "); for(number = minimum; number <= maximum; number++) { count = 0; for (i = 2; i <= number/2; i++) { if(number % i == 0) { count++; break; } } if(count == 0 && number != 1 ) { System.out.print(number + " "); } } } }
Please Enter the Minimum value : 10
Please Enter the Maximum value : 200
Prime Numbers from 1 to 100 are :
11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97 101 103 107 109 113 127 131 137 139 149 151 157 163 167 173 179 181 191 193 197 199