Write a Java program to print Matrix items or elements or values with an example. Or Write a Java program to display Multidimensional array items.
Generally, we can use any of the available Java loops to display matrix items. In this Java items example, we declared a matrix of integer items. Next, we used the For Loop to iterate the rows and column elements. We used the format function to display or print the matrix items as the output within the for loop.
public class PrintMatrixItems { public static void main(String[] args) { int[][] x = {{10, 20, 30}, {40, 50, 60}, {70, 80, 90}}; int i, j; System.out.println("------ The Matrix items ------"); for(i = 0; i < x.length; i++) { for(j = 0; j < x[0].length; j++) { System.out.format("\nThe Matrix Item at " + i + "," + j + " position = " + x[i][j]); } System.out.println(""); } } }
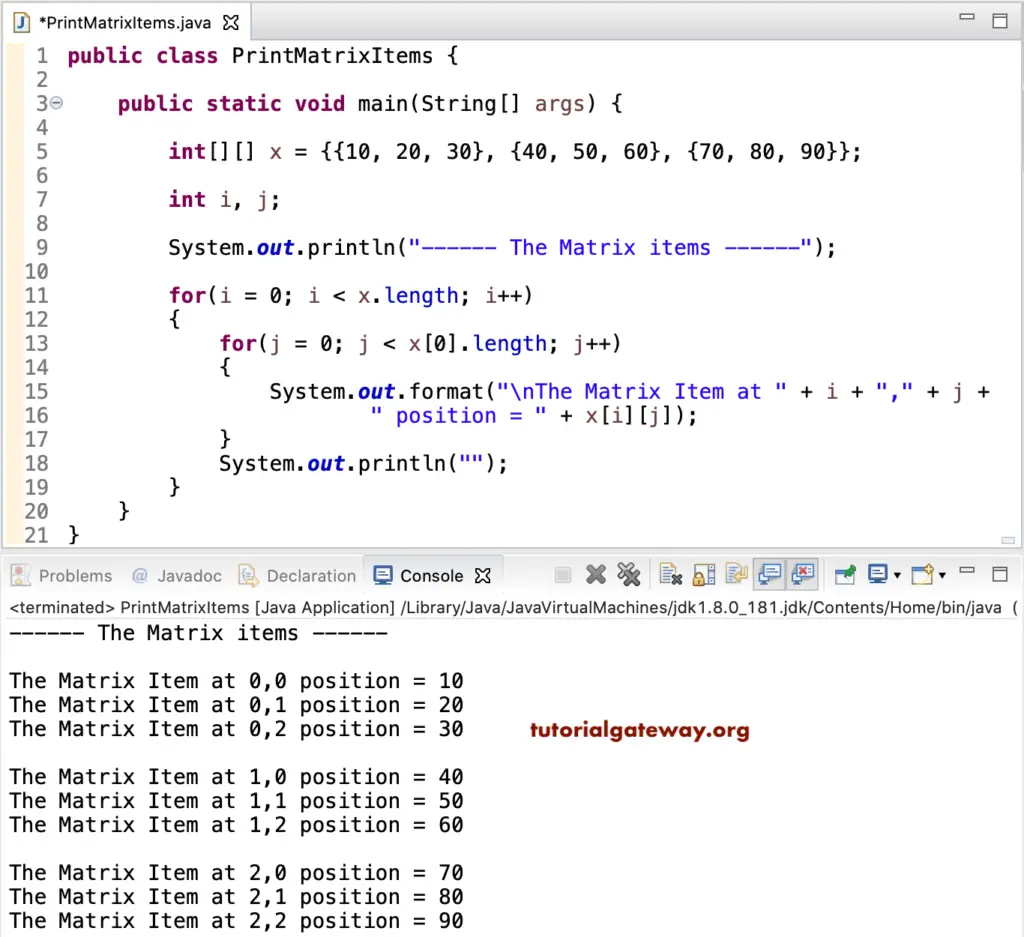
Java program to print Matrix items example 2
This Java display matrix items program is the same as above. However, this Java code allows the user to enter the number of rows, columns, and the matrix items using for loop.
import java.util.Scanner; public class PrintMatrixItems { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns; sc= new Scanner(System.in); System.out.println("\n Please Enter Rows and Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr1 = new int[rows][columns]; System.out.println("\n Please Enter the arr1 items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr1[i][j] = sc.nextInt(); } } System.out.println("\n---The total items are--- "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { System.out.format("\nThe Item at " + i + "," + j + " position = " + arr1[i][j]); } } } }
Please Enter Rows and Columns :
3 3
Please Enter the arr1 Items :
11 22 33
44 55 66
77 88 99
---The total items are---
The Item at 0,0 position = 11
The Item at 0,1 position = 22
The Item at 0,2 position = 33
The Item at 1,0 position = 44
The Item at 1,1 position = 55
The Item at 1,2 position = 66
The Item at 2,0 position = 77
The Item at 2,1 position = 88