Write a Java Program to print hollow rectangle star pattern using for loop. This Java rectangle star example uses nested for loop to iterate the rectangle rows and columns. The if-else condition check whether it is a rectangle first and last row or column. If it is true, print star; otherwise, print empty space.
package ShapePrograms; import java.util.Scanner; public class HollowRectangle1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Rectangle Rows & Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.println("Printing Hollow Rectangle Star Pattern"); for (int i = 0; i < rows; i++ ) { for (int j = 0 ; j < columns; j++ ) { if (i == 0 || i == rows - 1 || j == 0 || j == columns - 1) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(); } } }
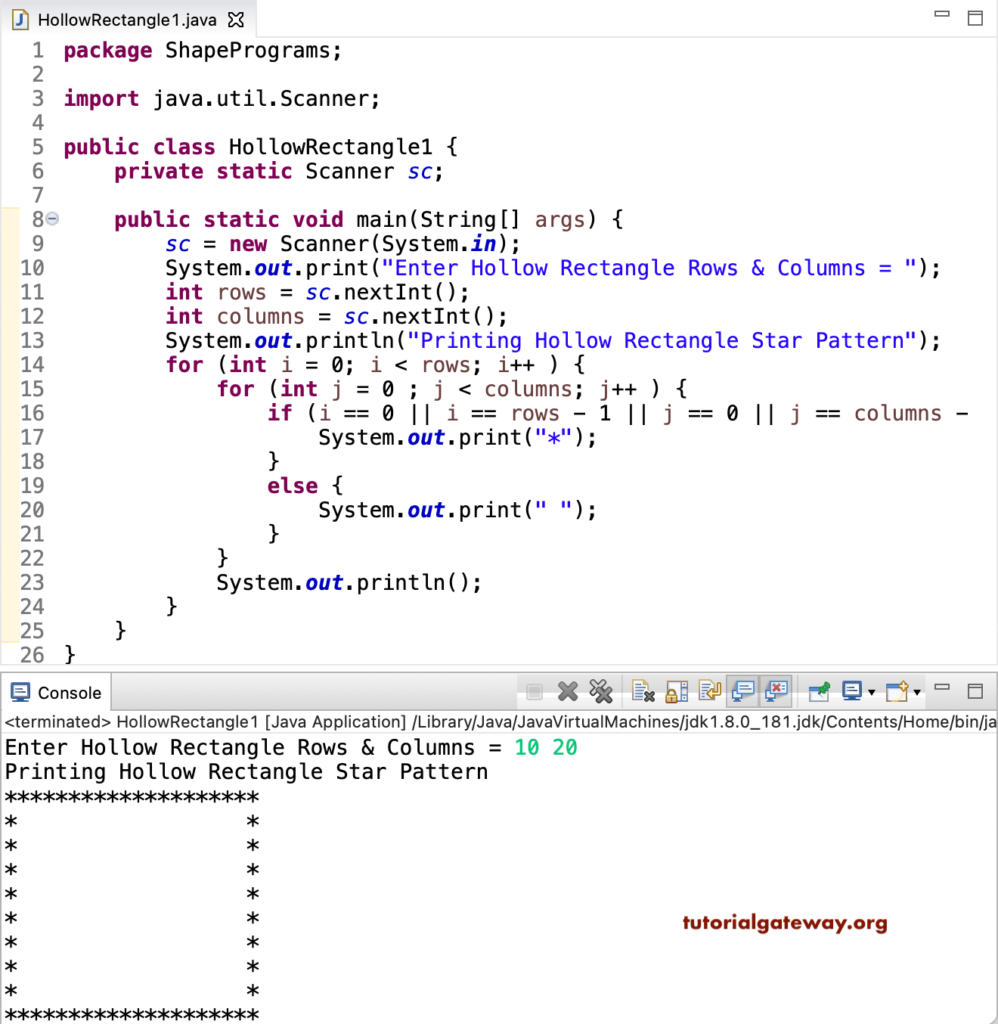
In this Java hollow rectangle star pattern program, we replaced the nested for loop with while loops.
package ShapePrograms; import java.util.Scanner; public class HollowRectangle2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Rectangle Rows & Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.println("-- Printing Hollow Rectangle Star Pattern --"); int i = 0; while (i < rows ) { int j = 0 ; while(j < columns ) { if (i == 0 || i == rows - 1 || j == 0 || j == columns - 1) { System.out.print("*"); } else { System.out.print(" "); } j++ ; } System.out.println(); i++; } } }
Enter Hollow Rectangle Rows & Columns = 8 15
-- Printing Hollow Rectangle Star Pattern --
***************
* *
* *
* *
* *
* *
* *
***************
Java Program to Print Hollow Rectangle Star Pattern using do while loop
package ShapePrograms; import java.util.Scanner; public class HollowRectangle3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Rectangle Rows & Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.println("-- Printing Hollow Rectangle Star Pattern --"); int j, i = 0; do { j = 0 ; do { if (i == 0 || i == rows - 1 || j == 0 || j == columns - 1) { System.out.print("*"); } else { System.out.print(" "); } } while(++j < columns ) ; System.out.println(); } while (++i < rows ) ; } }
Enter Hollow Rectangle Rows & Columns = 10 20
-- Printing Hollow Rectangle Star Pattern --
********************
* *
* *
* *
* *
* *
* *
* *
* *
********************
This Java code prints the hollow rectangle pattern of the user-entered symbol. Furthermore, we also created a HollowRectanglePattern function that prints the hollow rectangle pattern.
package ShapePrograms; import java.util.Scanner; public class HollowRectangle4 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Rectangle Rows & Columns = "); int rows = sc.nextInt(); int columns = sc.nextInt(); System.out.print("Enter Character for Hollow Rectangle Pattern = "); char ch = sc.next().charAt(0); System.out.println("Printing Hollow Rectangle Pattern"); HollowRectanglePattern(rows, columns, ch); } public static void HollowRectanglePattern(int rows, int columns, char ch) { for (int i = 0; i < rows; i++ ) { for (int j = 0 ; j < columns; j++ ) { if (i == 0 || i == rows - 1 || j == 0 || j == columns - 1) { System.out.print(ch); } else { System.out.print(" "); } } System.out.println(); } } }
Enter Hollow Rectangle Rows & Columns = 14 30
Enter Character for Hollow Rectangle Pattern = #
Printing Hollow Rectangle Pattern
##############################
# #
# #
# #
# #
# #
# #
# #
# #
# #
# #
# #
# #
##############################