Write a Java program to print hollow left pascals star triangle using for loop.
package ShapePrograms2; import java.util.Scanner; public class HollowLeftPascal1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j, k; System.out.print("Enter Hollow Left Pascals Triangle Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Hollow Left Pascals Triangle Star Pattern"); for (i = 1 ; i <= rows; i++ ) { for (j = rows ; j > i; j-- ) { System.out.print(" "); } for(k = 1; k <= i; k++) { if(k == 1 || k == i) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(); } for (i = 1; i <= rows - 1; i++ ) { for (j = 1 ; j <= i; j++ ) { System.out.print(" "); } for(k = rows - 1; k >= i; k--) { if(k == rows - 1 || k == i) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(); } } }
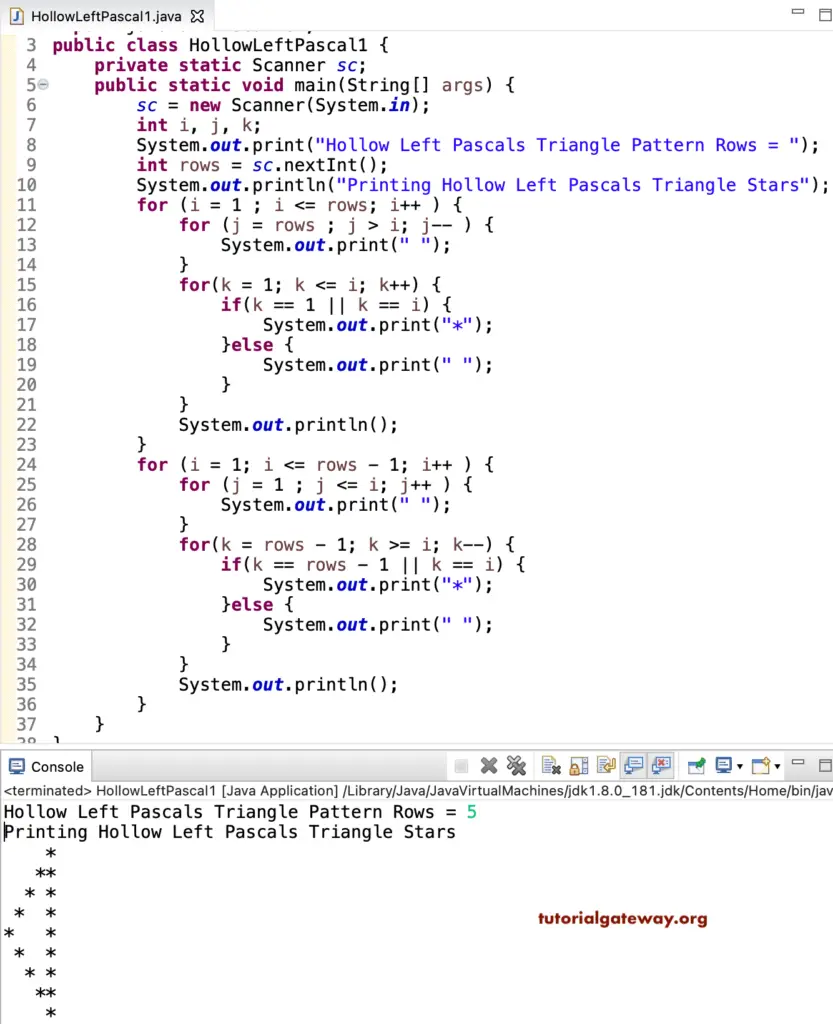
This Java example prints the stars in a hollow left pascals triangle pattern using a while loop.
package ShapePrograms2; import java.util.Scanner; public class HollowLeftPascal2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Left Pascals Triangle Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Hollow Left Pascals Triangle Star Pattern"); int i = 1, j, k; while (i <= rows ) { j = rows ; while ( j > i) { System.out.print(" "); j-- ; } k = 1; while( k <= i) { if(k == 1 || k == i) { System.out.print("*"); } else { System.out.print(" "); } k++; } System.out.println(); i++; } i = 1; while ( i <= rows - 1 ) { j = 1 ; while ( j <= i ) { System.out.print(" "); j++; } k = rows - 1; while( k >= i) { if(k == rows - 1 || k == i) { System.out.print("*"); } else { System.out.print(" "); } k--; } System.out.println(); i++; } } }
Enter Hollow Left Pascals Triangle Pattern Rows = 8
Printing Hollow Left Pascals Triangle Star Pattern
*
**
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
**
*
Java program to display hollow left pascals star triangle using do while loop.
package ShapePrograms2; import java.util.Scanner; public class HollowLeftPascal3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Left Pascals Triangle Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Hollow Left Pascals Triangle Star Pattern"); int i = 1, j, k; do { j = rows ; do { System.out.print(" "); } while ( j-- > i); k = 1; do { if(k == 1 || k == i) { System.out.print("*"); } else { System.out.print(" "); } } while( k++ <= i) ; System.out.println(); } while (++i <= rows ); i = 1; do { j = 1 ; do { System.out.print(" "); } while ( j++ <= i ); k = rows - 1; do { if(k == rows - 1 || k == i) { System.out.print("*"); } else { System.out.print(" "); } } while( --k >= i); System.out.println(); } while ( ++i <= rows - 1 ); } }
Enter Hollow Left Pascals Triangle Pattern Rows = 10
Printing Hollow Left Pascals Triangle Star Pattern
*
**
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
**
*