Write a Java Program to print hollow diamond star patterns using nested for loops and if-else statements. This Java hollow diamond example checks for outer diamond lines and prints stars to get that hollow shape.
package ShapePrograms; import java.util.Scanner; public class HollowDiamondPattern1 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j, k; System.out.print("Enter Hollow Diamond Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Hollow Diamond Star Pattern"); for (i = 1 ; i <= rows; i++ ) { for (j = 1 ; j <= rows - i; j++ ) { System.out.print(" "); } for (k = 1 ; k <= i * 2 - 1; k++ ) { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(); } for (i = rows - 1 ; i > 0; i-- ) { for (j = 1 ; j <= rows - i; j++ ) { System.out.print(" "); } for (k = 1 ; k <= i * 2 - 1; k++ ) { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } } System.out.println(); } } }
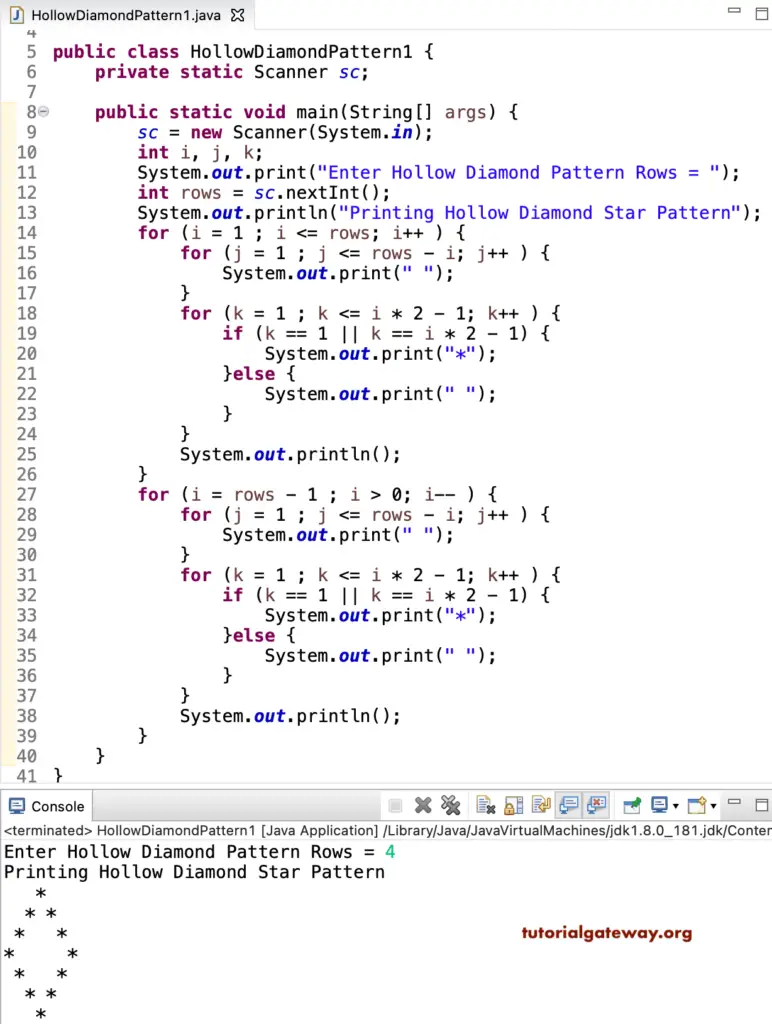
In this Java Hollow Diamond star pattern program, we replaced the for loop with a while loop.
package ShapePrograms; import java.util.Scanner; public class HollowDiamondPattern2 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j, k; System.out.print("Enter Diamond Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Diamond Star Pattern"); i = 1 ; while ( i <= rows) { j = 1 ; while ( j <= rows - i) { System.out.print(" "); j++; } k = 1 ; while ( k <= i * 2 - 1) { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } k++; } System.out.println(); i++ ; } i = rows - 1 ; while ( i > 0) { j = 1 ; while(j <= rows - i ) { System.out.print(" "); j++; } k = 1 ; while (k <= i * 2 - 1 ) { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } k++; } System.out.println(); i-- ; } } }
Enter Diamond Pattern Rows = 8
Printing Diamond Star Pattern
*
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
*
Java Program to Print Hollow Diamond Star Pattern using do while loop
package ShapePrograms; import java.util.Scanner; public class HollowDiamondPattern3 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); int i, j, k; System.out.print("Enter Diamond Pattern Rows = "); int rows = sc.nextInt(); System.out.println("Printing Diamond Star Pattern"); i = 1 ; do { j = 1 ; do { System.out.print(" "); } while ( j++ <= rows - i) ; k = 1 ; do { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } } while ( ++k <= i * 2 - 1); System.out.println(); } while ( ++i <= rows) ; i = rows - 1 ; do { j = 1 ; do { System.out.print(" "); } while(j++ <= rows - i ); k = 1 ; do { if (k == 1 || k == i * 2 - 1) { System.out.print("*"); } else { System.out.print(" "); } } while (++k <= i * 2 - 1 ); System.out.println(); } while ( --i > 0); } }
Enter Diamond Pattern Rows = 10
Printing Diamond Star Pattern
*
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
*
In this Java example, the HollowDiamondPattern function prints the hollow diamond pattern of a given symbol.
package ShapePrograms; import java.util.Scanner; public class HollowDiamondPattern4 { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.print("Enter Hollow Diamond Pattern Rows = "); int rows = sc.nextInt(); System.out.print("Enter Character for Hollow Diamond Pattern = "); char ch = sc.next().charAt(0); System.out.println("Printing Hollow Diamond Pattern"); HollowDiamondPattern(rows, ch); } public static void HollowDiamondPattern(int rows, char ch) { int i, j, k; for (i = 1 ; i <= rows; i++ ) { for (j = 1 ; j <= rows - i; j++ ) { System.out.print(" "); } for (k = 1 ; k <= i * 2 - 1; k++ ) { if (k == 1 || k == i * 2 - 1) { System.out.print(ch); } else { System.out.print(" "); } } System.out.println(); } for (i = rows - 1 ; i > 0; i-- ) { for (j = 1 ; j <= rows - i; j++ ) { System.out.print(" "); } for (k = 1 ; k <= i * 2 - 1; k++ ) { if (k == 1 || k == i * 2 - 1) { System.out.print(ch); } else { System.out.print(" "); } } System.out.println(); } } }
Enter Hollow Diamond Pattern Rows = 13
Enter Character for Hollow Diamond Pattern = $
Printing Hollow Diamond Pattern
$
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$