Write a Java program to print characters in a string with an example. In the following java example, we used for loop to iterate each and every character from start to end and print all the characters in the given string. Here, we used the Java string length function to get the str length.
import java.util.Scanner; public class PrintStringChars1 { private static Scanner sc; public static void main(String[] args) { String str; int i; sc= new Scanner(System.in); System.out.print("\n Please Enter any String to Print = "); str = sc.nextLine(); for(i = 0; i < str.length(); i++) { System.out.println("The Character at Position " + i + " = " + str.charAt(i)); } } }
Java string Characters output
Please Enter any String to Print = Hello
The Character at Position 0 = H
The Character at Position 1 = e
The Character at Position 2 = l
The Character at Position 3 = l
The Character at Position 4 = o
Java Program to Print Characters in a String Example 2
In this Java example, we converted the String to Character array using toCharArray() method. Next, we iterated that char array and printed the characters.
import java.util.Scanner; public class PrintStringChars2 { private static Scanner sc; public static void main(String[] args) { String str; int i; sc= new Scanner(System.in); System.out.print("\n Please Enter any String to Print = "); str = sc.nextLine(); char[] ch = str.toCharArray(); for(i = 0; i < ch.length; i++) { System.out.println("Character at Position " + i + " = " + ch[i]); } } }
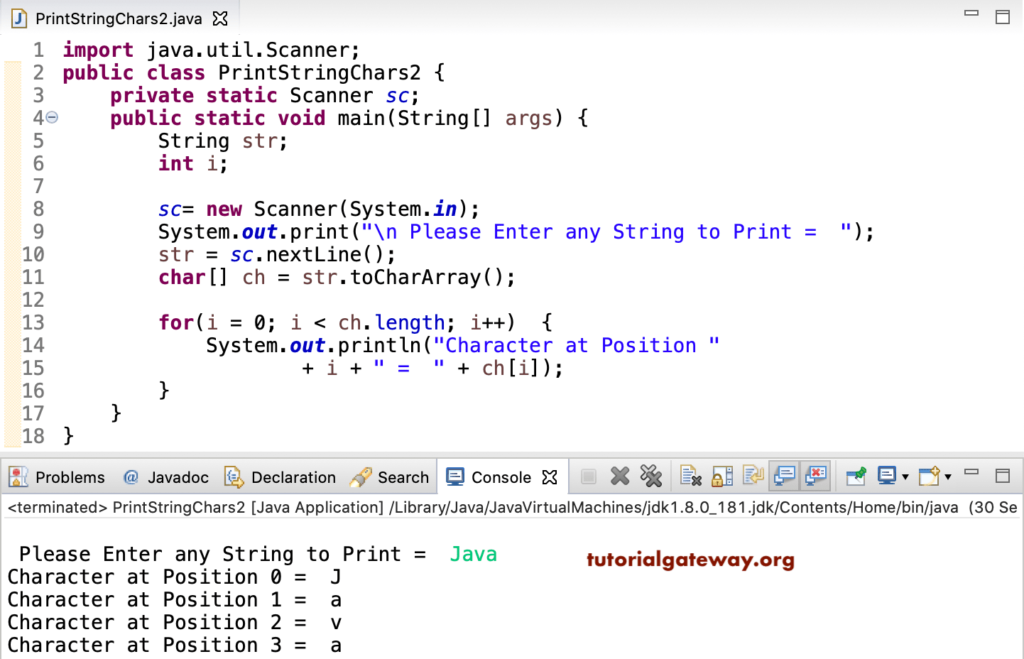
Java Program to display Characters in a String using While Loop
import java.util.Scanner; public class PrintStringChars3 { private static Scanner sc; public static void main(String[] args) { String str; int i = 0; sc= new Scanner(System.in); System.out.print("\nPlease Enter any String to Print = "); str = sc.nextLine(); while(i < str.length()) { System.out.println("The Character at Position " + i + " = " + str.charAt(i)); i++; } } }
Please Enter any String to Print = learn Java
Character at Position 0 = l
Character at Position 1 = e
Character at Position 2 = a
Character at Position 3 = r
Character at Position 4 = n
Character at Position 5 =
Character at Position 6 = J
Character at Position 7 = a
Character at Position 8 = v
Character at Position 9 = a
In this Java Print String Characters example, we speared the code by creating a separate method.
import java.util.Scanner; public class PrintStringChars4 { private static Scanner sc; public static void main(String[] args) { String str; sc= new Scanner(System.in); System.out.print("\n Please Enter any String to Print = "); str = sc.nextLine(); PrintStringCharacters(str); } public static void PrintStringCharacters(String str) { for(int i = 0; i < str.length(); i++) { System.out.println("The Character at Position " + i + " = " + str.charAt(i)); } } }
Please Enter any String to Print = Programs
The Character at Position 0 = P
The Character at Position 1 = r
The Character at Position 2 = o
The Character at Position 3 = g
The Character at Position 4 = r
The Character at Position 5 = a
The Character at Position 6 = m
The Character at Position 7 = s
It is another Java example to print string characters. Here, we are not allowing the Java code to print empty spaces or space between words.
import java.util.Scanner; public class PrintStringChars5 { private static Scanner sc; public static void main(String[] args) { String str; sc= new Scanner(System.in); System.out.print("\nPlease Enter any String to Print = "); str = sc.nextLine(); PrintStringCharacters(str); } public static void PrintStringCharacters(String str) { for(int i = 0; i < str.length(); i++) { if(str.charAt(i) != ' ') { System.out.println("The Character at Position " + i + " = " + str.charAt(i)); } } } }
Please Enter any String to Print = Gateway
The Character at Position 0 = G
The Character at Position 1 = a
The Character at Position 2 = t
The Character at Position 3 = e
The Character at Position 4 = w
The Character at Position 5 = a
The Character at Position 6 = y