How to write a Java Program to print the ASCII Value of a Character with an example? In Java programming, every character has its own ASCII value (it is nothing but an integer). When we assign a character to a variable, instead of storing itself, the ASCII value of that character is stored. For example, the ASCII value for the A is 65.
Java Program to print ASCII Value of a Character Example
In this program, the user requested to enter any letter. Next, this program displays the ASCII value of that particular character.
import java.util.Scanner; public class ASCIIofCharacter { private static Scanner sc; public static void main(String[] args) { char ch; sc = new Scanner(System.in); System.out.print(" Please Enter any Character : "); ch = sc.next().charAt(0); //Assigning the Character to Integer value int num1 = ch; // You can also Type cast the Character Value to convert it into Integer value int num2 = (int)ch; System.out.println("\n The ASCII value of a given character " + ch + " = " + num1); System.out.println("\n The ASCII value of a given character " + ch + " = " + num2); // Type casting directly inside the system.out.println System.out.println("\n The ASCII value of a given character " + ch + " = " + (int)ch); } }
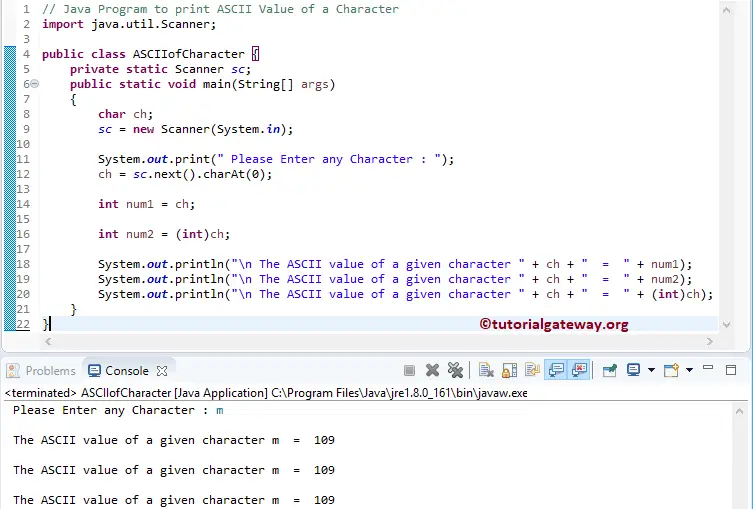
This time we are inserting words. Though it is a word, this Java program accepts the first letter only because of sc.next().charAt(0). It read a letter at the position at index position 0. Please refer ASCII Table article to understand the list of ASCII Characters and their decimal, Hexadecimal, and Octal numbers.
Please Enter any Character : Tutorial
The ASCII value of a given character T = 84
The ASCII value of a given character T = 84
The ASCII value of a given character T = 84